Understanding the Nuances of JavaScript's 'in' Operator A Deep Dive into Property Checking
Understanding the Nuances of JavaScript's 'in' Operator A Deep Dive into Property Checking - Basic Syntax and Functionality of the 'in' Operator
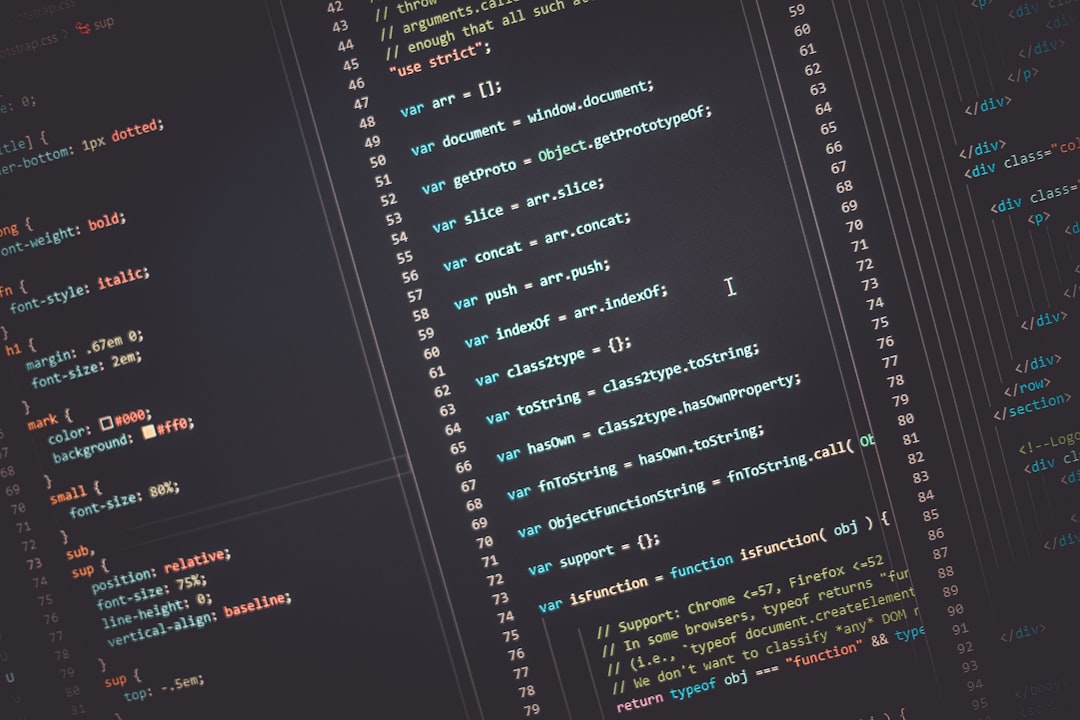
At its core, the `in` operator in JavaScript is a simple yet effective way to determine whether a particular property exists within an object. Its output is a boolean value, true or false, reflecting the property's presence. The basic format is intuitive: you provide the property's name (as a string or symbol), then the `in` keyword, and lastly, the object itself, e.g., `"propertyName" in object`.
It's crucial to realize that the `in` operator isn't limited to checking properties directly defined on an object. It also delves into the inheritance chain, examining if the property exists anywhere along the prototype hierarchy. This characteristic differentiates it from methods like `hasOwnProperty`, which are more focused on properties explicitly attached to a given object. This broader scope can be beneficial, particularly when working with complex object structures.
Moreover, this versatile operator isn't confined to just objects. Arrays can also leverage it to check for the existence of indices. For instance, `0 in array` will verify if the array has an element at the zeroth index. Grasping how the `in` operator handles prototype chains is vital when managing sophisticated object-oriented designs in JavaScript.
At its core, JavaScript's `in` operator determines whether a property, be it owned or inherited, exists within an object. This contrasts with how some other languages handle property existence, where a check might solely rely on whether a property is not `null` or `undefined`. JavaScript's `in` operator simply focuses on the property's presence, regardless of its actual value.
Interestingly, this property-checking behavior extends to strings, which JavaScript treats akin to arrays. When used on strings, `in` checks the index of a character, a feature that can lead to confusion if not acknowledged. The same principle holds for arrays, where `in` checks for the existence of an index. This attribute can serve as a useful debugging tool, particularly for comprehending sparse arrays.
Though typically efficient, the `in` operator's performance can degrade in intricate object structures due to the need to traverse the prototype chain. Also, it's worth noting that `in` can return `true` even for non-enumerable properties, potentially misleading developers who expect checks to only consider explicitly listed properties.
It's curious that JavaScript allows the `in` operator to be used with undeclared variables, yet results in a reference error if a variable hasn't been declared at all. This highlights the importance of meticulous variable scoping.
When working with TypeScript, `in` aids in runtime object type determination, boosting type safety and error reduction. Its applicability extends to array-like objects like DOM NodeLists, enabling verification of element inclusion in these data structures.
While valuable for property checks, overreliance on `in` can contribute to less clear coding practices, especially when compared to more explicit property validation techniques. Relying excessively on it might encourage less maintainable code and can potentially obscure the intent of the code when read by other developers. Understanding when `in` is best utilized and when more specific property validation methods are preferable remains crucial for crafting robust and understandable JavaScript applications.
Understanding the Nuances of JavaScript's 'in' Operator A Deep Dive into Property Checking - Comparing 'in' with the hasOwnProperty Method
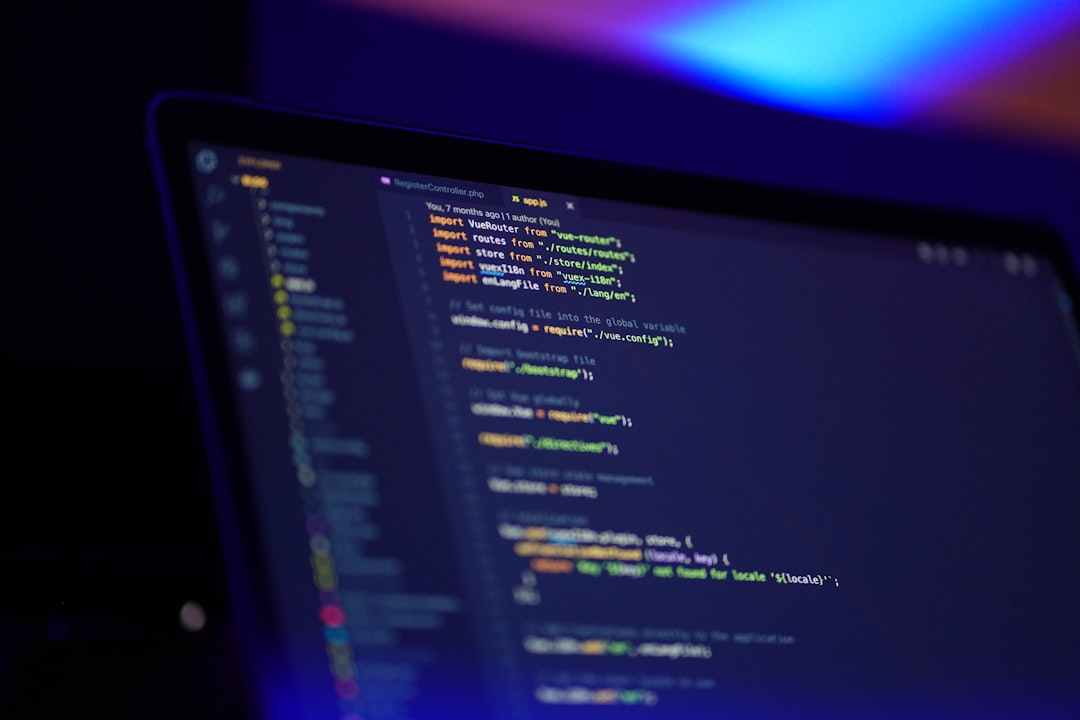
When deciding between using the `in` operator and the `hasOwnProperty` method, their core differences come into play. The `hasOwnProperty` method isolates a property's direct ownership within an object, ignoring any properties inherited from its prototype. In contrast, `in` probes both directly owned and inherited properties. This means `hasOwnProperty` shines when you need to ensure a property is genuinely part of an object, eliminating confusion from inherited properties. On the other hand, `in`'s broader scope can be helpful when working with object hierarchies where inherited properties are relevant. Choosing between them relies on carefully considering what you need your property checks to achieve within your JavaScript code. The choice hinges on whether your code necessitates verifying direct ownership or if inherited properties are equally important in the context of your checks.
Let's delve into the nuances of comparing the `in` operator with the `hasOwnProperty` method. Both are geared towards property checking within objects, but their approaches and outcomes can differ significantly.
The `hasOwnProperty` method, being a part of the `Object.prototype`, is inherited by every object, making it universally accessible. This contrasts with the `in` operator, which operates independently of an object's prototype chain. While `in` explores the entire inheritance hierarchy to determine if a property exists anywhere, `hasOwnProperty` focuses solely on whether a property is directly associated with the object itself. This distinction is pivotal when you aim to prevent inheritance-related quirks or unexpected behavior.
Interestingly, `hasOwnProperty` overlooks symbols, while `in` can handle both string and symbol properties. This adds a layer of granularity to property checks, particularly in modern JavaScript applications where symbols play a role. Additionally, `in` can produce unexpected outcomes when it comes to non-enumerable properties, returning `true` even for properties that aren't accessible through a standard `for...in` loop. On the other hand, `hasOwnProperty` strictly returns `true` only for properties directly defined on the object, without relying on inheritance.
When applied to arrays, the `in` operator checks if a specific index exists, unlike `hasOwnProperty`, which isn't relevant for array elements due to their non-string keys. `0 in []` will rightfully return `true`, while `[].hasOwnProperty(0)` might return `false` in some undocumented scenarios, highlighting potential inconsistencies.
Performance-wise, `in` can experience slower execution in complex inheritance hierarchies due to the traversal of the prototype chain. Conversely, `hasOwnProperty` maintains a more predictable performance because it avoids this traversal.
It's worth noting that within strict mode, the `in` operator displays subtle behavior changes when encountering undeclared variables, potentially leading to reference errors. In contrast, `hasOwnProperty` remains unaffected by this situation since it operates solely on objects.
In some cases, `in` may unexpectedly interpret an object's `toString` method as a property, potentially causing confusion for developers who might not typically consider inherited methods in the same vein as other properties.
It's also worth acknowledging that object mutability can impact the behavior of both operators. If you add or change properties dynamically, the results of using either operator might diverge from initial expectations.
Given that JavaScript allows objects to be extended dynamically, this flexibility can introduce complexities when utilizing either `in` or `hasOwnProperty`. Developers should be mindful of how property modifications can affect these checks to prevent potential errors in their code.
By understanding these comparisons, we can make more informed decisions when checking for property existence in JavaScript, ultimately fostering more robust and predictable applications.
Understanding the Nuances of JavaScript's 'in' Operator A Deep Dive into Property Checking - Prototype Chain Considerations When Using 'in'
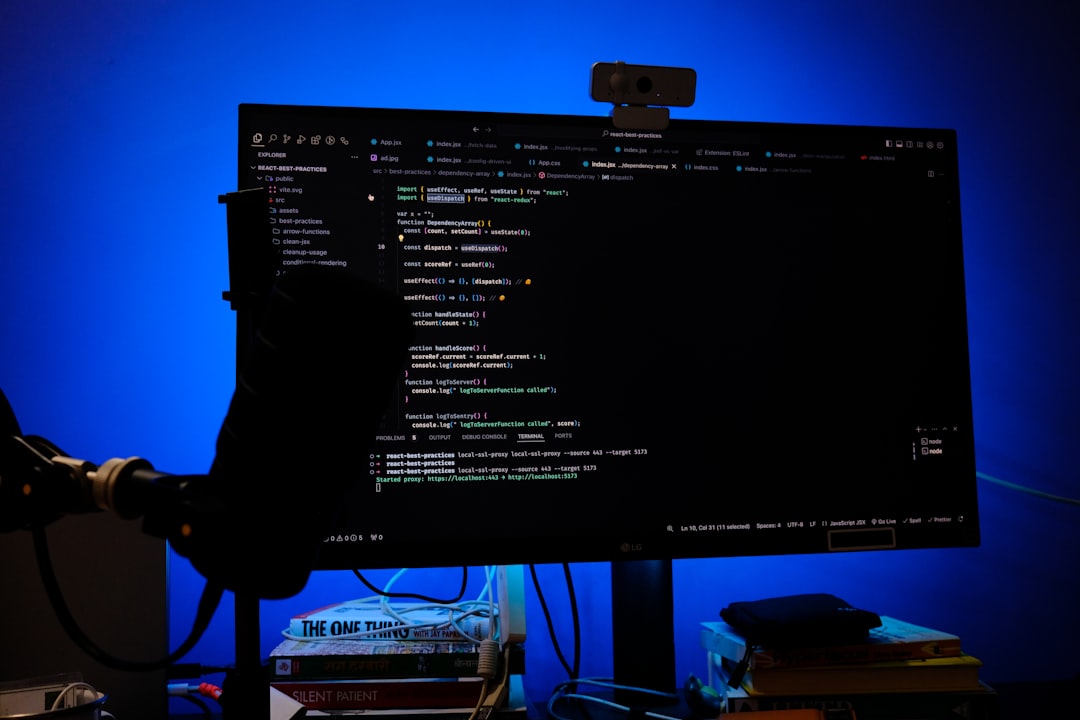
### Prototype Chain Considerations When Using 'in'
The `in` operator's behavior is intertwined with JavaScript's prototype chain. This means it doesn't just check if a property is directly on an object, but also searches up the inheritance hierarchy. While this can be convenient for verifying properties across multiple levels of an object's lineage, it can also introduce performance challenges. If an object has a deep prototype chain, the `in` operator might need to search through many levels before finding a property or concluding that it's not present. This process of traversing up the chain adds an overhead that can become significant if not managed. Consequently, developers need to be mindful of the potential for performance impacts when working with objects that have complex prototype structures and the `in` operator. Keeping prototype chains reasonably shallow can prevent the operator from becoming a source of performance issues, promoting the efficient use of this fundamental JavaScript tool.
JavaScript's `in` operator, while useful for checking property existence, takes on a unique character due to its interaction with the prototype chain. This characteristic can introduce a level of complexity that developers need to be aware of. For example, you might find yourself unexpectedly accessing properties that aren't directly related to the object's intended function, which can make debugging a little more difficult.
One subtle aspect of `in` is its lack of differentiation between property attributes like writeability, enumerability, or configurability. This can lead to situations where you might not have a full picture of a property's role, which can be problematic when your code expects specific behavior based on these attributes.
When working with objects created using `Object.create(null)`, which intentionally lack a prototype, `in` behaves differently. This might lead to unexpected results because `in` will only return `true` for properties that are directly on the object, not inherited through a prototype. Developers accustomed to prototype-based inheritance should be aware of this.
It's curious that when you mix string keys with array indices, `in` behaves in a way that can obscure the intended behavior. For instance, if you assign a property to an array using a string key like `array["0"] = "test"`, then `0 in array` will return `true`, but `array.hasOwnProperty(0)` will be `false`. This can be confusing as it shows how `in` can sometimes present a broader view of property existence than expected.
The performance of `in` depends heavily on the length and complexity of an object's prototype chain. As such, if you use it frequently in complex object structures, it could lead to performance bottlenecks, particularly in performance-sensitive applications.
Proxy objects in JavaScript introduce a twist to `in`'s interaction. When a Proxy is used to intercept property accesses, using `in` triggers the `has` trap. This allows developers to define custom behavior for property checks, which might differ from what developers anticipate when working with objects without a Proxy in place. This can be something to keep in mind for developers working with more advanced JavaScript patterns.
Another aspect to consider is the interaction of `in` with non-enumerable properties. While `in` confirms the presence of a property even if it comes from a parent in the prototype chain, it's important to remember that non-enumerable properties won't show up in a `for...in` loop. This discrepancy between property existence and discoverability can cause issues if you're relying on both checks in your code.
The `in` operator isn't limited to working only with complex objects, it also interacts with primitive values and their wrapper objects. If you take the primitive 0 and wrap it in an object `Object(0)`, `0 in Object(0)` evaluates to `true`. It's an example of how `in`'s approach to property existence checks extends beyond traditional object structures.
Modern JavaScript's use of Symbol properties finds a counterpart in the `in` operator, allowing developers to check for the existence of Symbol properties associated with an object. It offers more complete control over property checking in the context of JavaScript's object model, especially where developers leverage Symbol properties for specific application needs.
When combining objects using techniques like `Object.assign()`, keep in mind that `in` checks might be affected. The merging of objects can potentially create scenarios where new properties are introduced, leading to unexpected "true" results for property checks. This can create difficulties in checking data integrity or ensuring your code reacts correctly to data within an application. These interactions, while seemingly basic, highlight how being aware of nuances in how JavaScript handles inheritance, prototypes, and property checking can be critical in developing robust and predictable software.
Understanding the Nuances of JavaScript's 'in' Operator A Deep Dive into Property Checking - Error Prevention Strategies with 'in' for Dynamic Properties
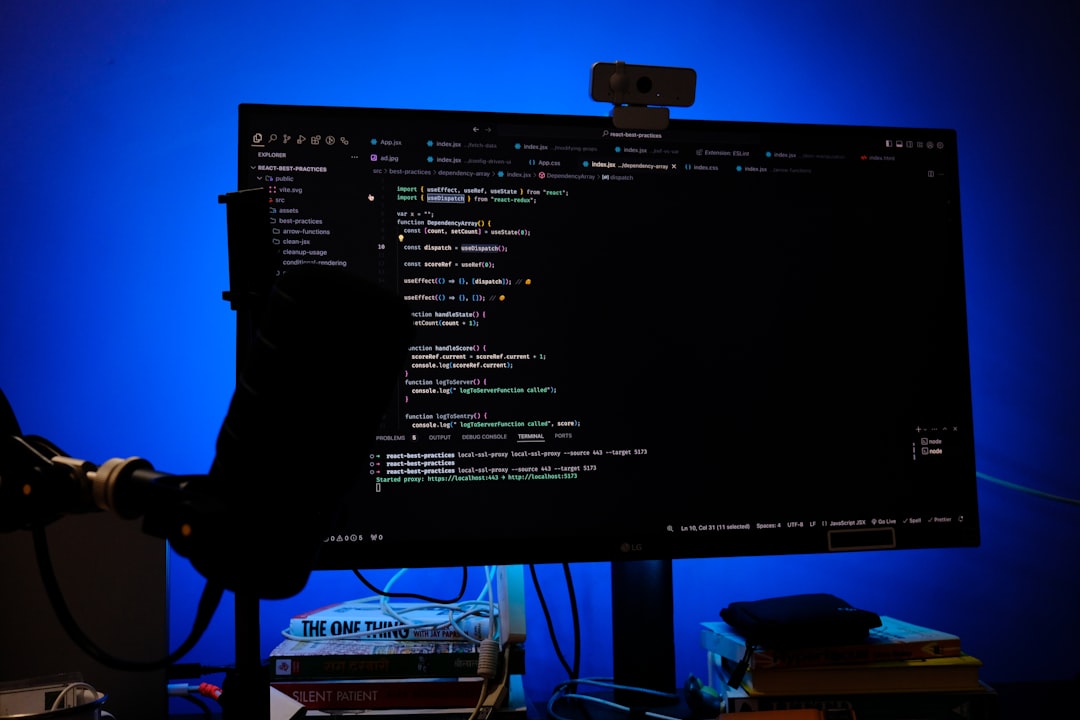
When dealing with dynamic properties in JavaScript, leveraging the `in` operator effectively hinges on understanding its interaction with both direct properties and the prototype chain. To prevent errors during runtime, it's crucial to verify the presence of a property before attempting to access or modify it. This proactive approach avoids the potential pitfalls of interacting with properties that might not exist. However, excessive reliance on `in` can lead to code that is less clear and harder to maintain, especially when working with intricate objects or situations involving inheritance. To mitigate these risks, consider implementing comprehensive error prevention strategies. These strategies might involve explicit checks for property existence and adhering to consistent coding patterns for greater clarity. Furthermore, understanding how `in` treats non-enumerable properties and being mindful of potential performance impacts when traversing lengthy prototype chains helps in optimizing the use of the operator and contributes to a more robust foundation for your JavaScript applications.
When exploring how JavaScript's `in` operator interacts with dynamic properties, a few intriguing aspects emerge. First, if you're adding properties to an object dynamically, `in` will readily reflect those changes. This can occasionally lead to surprising results if your code assumes a static state before modifications.
Secondly, using `in` can affect performance, especially when working with intricate prototype chains. In performance-critical sections of your application, it might be wise to use profiling tools to determine if `in` is causing slowdowns, as its traversal of the prototype chain can add overhead.
A rather curious behavior is its treatment of non-enumerable properties. Even if these aren't visible via `for...in` loops, `in` will still flag their existence. This can complicate debugging because you might accidentally check for a property that's not easily accessible through conventional methods.
Another interesting aspect is that `in` requires variable declarations. Using it with undeclared variables will result in an error, underscoring the importance of proper variable scoping, particularly in strict mode.
Furthermore, TypeScript developers can benefit from `in`'s runtime type determination, improving type safety and error prevention. This adds a layer of rigor to dynamic property checks in the TypeScript ecosystem.
When employing Proxy objects in JavaScript to control property access, the `in` operator activates the `has` trap, enabling custom logic for property checks. This additional customization layer adds another dimension to how you can manage property existence within JavaScript, but also increases complexity.
`in` handles both string and symbol properties, showcasing its adaptability. However, this flexibility might introduce confusion when managing different types of properties within complex applications.
The interactions with arrays are somewhat subtle. While `in` checks indices, using a string key to assign properties to an array can produce results that conflict with typical integer-based indexing. This can potentially impact the intended behavior of array-related code.
Another interesting case is when dealing with objects created via `Object.create(null)`. These objects lack a prototype, causing `in` to behave differently – only checking for directly defined properties, not inherited ones. It's a subtle change that can lead to unexpected behavior if you're not aware of it.
Finally, the mutable nature of JavaScript objects can make predicting `in`'s behavior a bit tricky. Properties can be added, removed, or modified on the fly. This dynamic landscape means `in`'s results can fluctuate with the object's lifecycle, so you need to account for these changes when you're relying on its output.
These insights highlight the intricate nature of using the `in` operator for dynamic property checks. It's essential to thoroughly test and ensure code clarity to prevent errors arising from unexpected behavior and complex interactions with JavaScript's object model.
Understanding the Nuances of JavaScript's 'in' Operator A Deep Dive into Property Checking - Performance Implications of 'in' vs Other Property Checking Methods
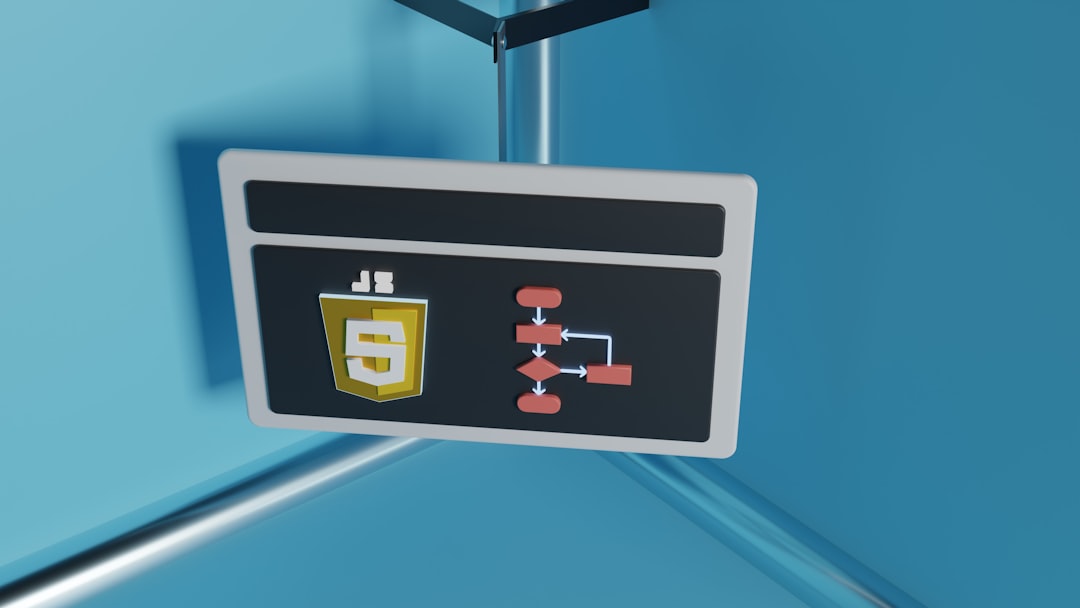
When evaluating the performance of the `in` operator compared to alternatives like `hasOwnProperty`, we need to understand their distinct operational approaches. The `in` operator, due to its exploration of the prototype chain, can become less efficient when dealing with complex inheritance hierarchies. The prototype chain traversal, while beneficial for checking inherited properties, adds an extra step. Conversely, `hasOwnProperty` is often more efficient since its sole focus is on confirming a property's direct association with an object, avoiding the overhead of navigating the prototype chain. Further, `hasOwnProperty` eliminates any ambiguity that can arise with inherited properties that might lead to unexpected results when using `in`. Thus, in scenarios where performance is paramount, knowing the specific nature of the property check—whether it needs to consider inherited traits or only direct properties—allows developers to optimize their JavaScript code by selecting the most fitting approach.
The `in` operator's performance can be affected by the complexity of an object's prototype chain. When dealing with objects having multiple levels of inheritance, the search for a property becomes a multi-layered process, potentially slowing things down. This is especially notable when the prototype chain is deep and involves many levels.
One curious behavior of `in` is its ability to detect non-enumerable properties. While they aren't visible using tools like `for...in` loops, `in` will still register them as existing. This can be a source of confusion because you might accidentally assume a property is available through a standard loop when it's not.
`in` is flexible enough to check for properties represented as both strings and Symbols. This feature is handy but can be a source of potential confusion, especially if developers aren't aware of or consistently using Symbol properties.
Surprisingly, the `in` operator throws an error if you use it with undeclared variables. This serves as a reminder to be careful about your variable scoping, especially if you're working in strict mode.
Arrays and the `in` operator have an interesting relationship. While it can be used to check for array indices, assigning properties to an array using string keys can introduce inconsistencies. You might see `0 in array` return true, while `array.hasOwnProperty(0)` returns false. This inconsistency is something to keep in mind when working with arrays.
If you dynamically add properties to an object, `in` immediately reflects those changes. This responsiveness can be helpful, but it also means that if your code assumes a static state before changes are made, the `in` operator may produce unexpected results.
Proxy objects make using `in` a bit more complex because it activates the `has` trap. This feature allows you to customize the behavior of property existence checks, which is handy, but also means that your code could behave differently from what you'd expect in a scenario without a proxy.
Objects created with `Object.create(null)` lack a prototype. This means that `in` only checks for properties that are directly defined on the object, ignoring any inheritance. Developers who are used to working with objects that have prototypes should be aware of this.
Given the potential for performance bottlenecks with the `in` operator in more complex scenarios, consider using profiling tools to check if its usage in your code is impacting performance, especially in parts of your app where performance is crucial.
The mutable nature of JavaScript objects means that the results of the `in` operator can change as properties are added, removed, or modified. Therefore, it's essential to keep this dynamism in mind to avoid unintended consequences and surprises in your code.
Understanding the Nuances of JavaScript's 'in' Operator A Deep Dive into Property Checking - Common Pitfalls and Best Practices for 'in' Usage in JavaScript
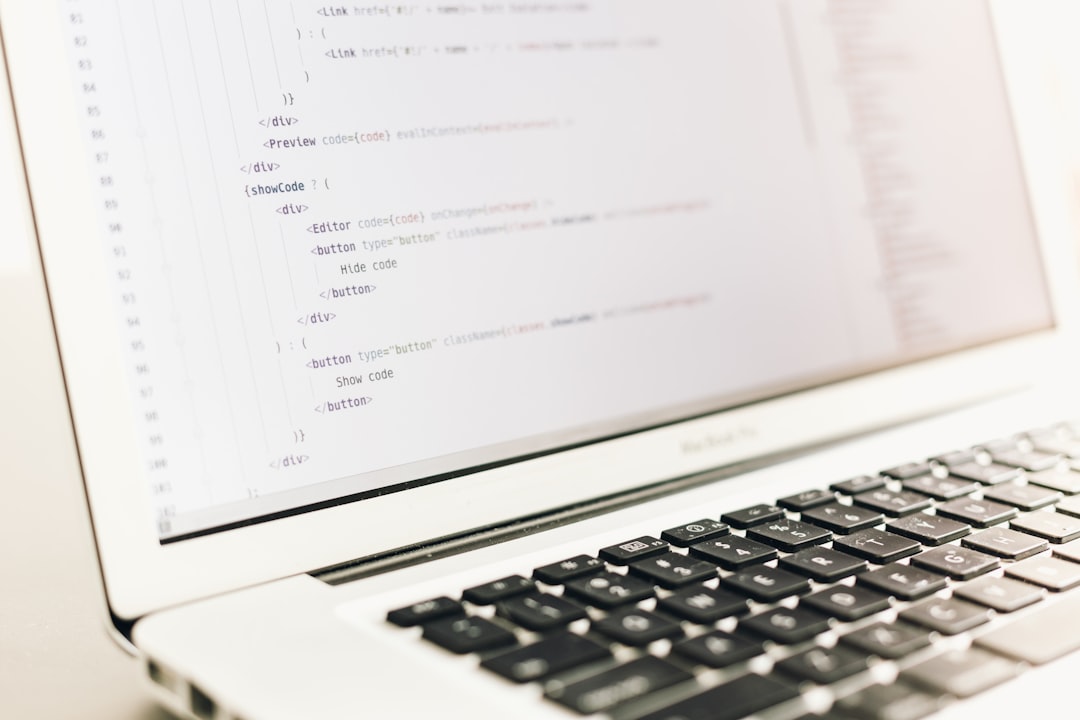
The `in` operator in JavaScript, while useful for determining if a property exists within an object, can lead to unexpected results if not used carefully. One frequent misunderstanding is that it only verifies properties directly on the object itself. Instead, it also explores the prototype chain, checking if the property exists at any level. This behavior can cause confusion, especially when encountering properties inherited from parent objects or properties that are non-enumerable. To avoid this, it's generally a good practice to use `hasOwnProperty` when you specifically want to check for properties that are directly defined on the object and not inherited.
Furthermore, keep in mind that while the `in` operator is a flexible tool, it can also impact performance, particularly if an object has a deeply nested prototype chain. This traversal can create performance bottlenecks in parts of your code where efficiency is crucial. Therefore, you should consider the trade-offs when using `in` in loops or frequently in your code. Prioritizing clear and well-defined property checks ultimately leads to more reliable and easier-to-maintain JavaScript code. By understanding these potential challenges and best practices, you can utilize the `in` operator more effectively within your projects.
When using the `in` operator in JavaScript, several aspects can lead to unexpected behavior or performance issues. One notable aspect is its traversal of the prototype chain to check for property existence. This can impact performance, particularly in scenarios with intricate inheritance structures, where it might be slower than options like `hasOwnProperty`, which only looks for directly owned properties.
The `in` operator can produce surprising results when dealing with non-enumerable properties. While it returns `true` for such properties, they don't appear in `for...in` loops. This difference can confuse developers who might not anticipate this behavior.
Furthermore, the `in` operator adapts to changes in object properties in real-time. This flexibility can be useful for dynamic properties but might lead to issues if your code expects a fixed state before property modifications occur.
Using `in` with undeclared variables will result in a reference error, emphasizing the importance of careful variable scoping, particularly in strict mode. This differs from property checks like `hasOwnProperty`, which only works on existing objects.
The operator's ability to check for both string and symbol properties is quite flexible but can cause problems if developers aren't careful about how they handle different property types across their code.
When used with arrays, `in` might behave in unexpected ways due to its handling of both indices and string-based properties. For instance, while `0 in []` returns `true`, `[].hasOwnProperty(0)` can return `false` under specific circumstances, which highlights a potential source of inconsistency.
Proxy objects also introduce a layer of complexity when used with the `in` operator. It activates the `has` trap, providing the ability to customize property checks, which can alter the intended outcomes of your code.
Objects built using `Object.create(null)` do not inherit properties, causing `in` to only check directly defined properties. This might be unexpected for developers accustomed to prototype-based inheritance.
JavaScript objects are mutable, so the results of `in` can change over time as properties are added, removed, or changed. If relying on `in`, you need to account for these changes throughout the object's lifetime.
Finally, performance can be affected by using the `in` operator, especially in performance-critical sections of your code. It's advisable to use profiling tools to monitor its impact, particularly when dealing with complex prototype chains, to ensure that its performance characteristics align with your application's needs.
Understanding these subtleties of the `in` operator helps avoid potential errors and ensures your JavaScript code is efficient and predictable.
More Posts from :