Step-by-Step Guide Creating and Initializing Arrays in Java for Beginners
Step-by-Step Guide Creating and Initializing Arrays in Java for Beginners - Declaring an Array Variable in Java
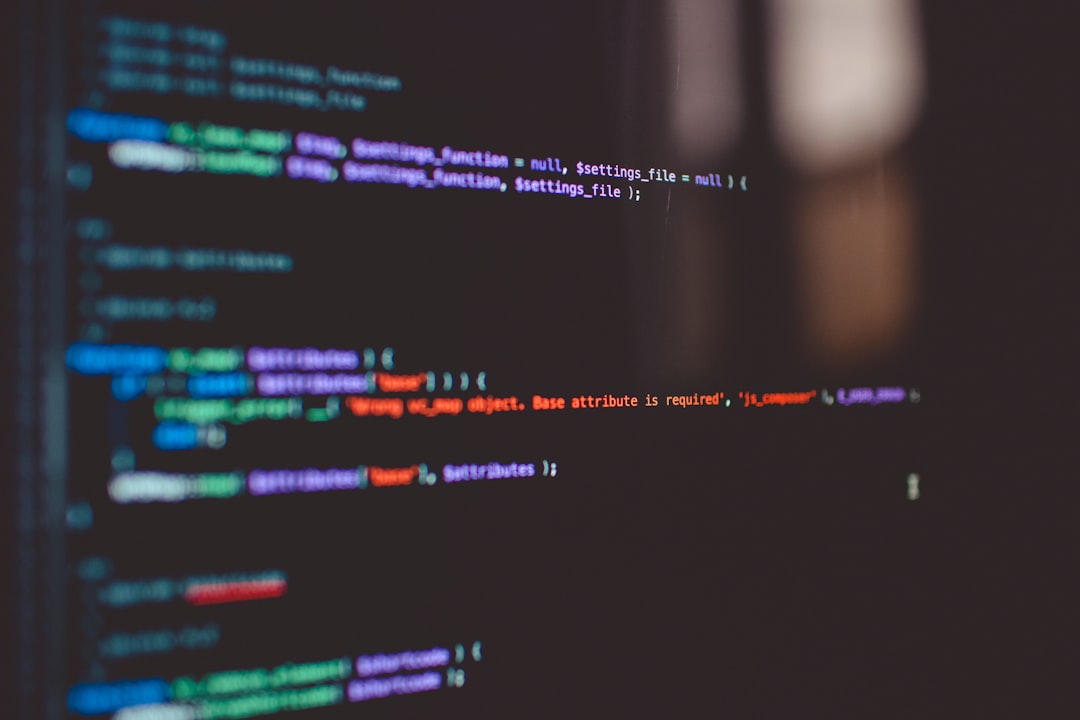
Declaring an array variable in Java requires defining the data type and allocating memory using the `new` keyword. The syntax follows the pattern `datatype arrayName`, where `datatype` specifies the kind of elements the array will hold. The size of the array is set at runtime, making it fixed. You can initialize an array during declaration by specifying the size, for example, `int[] numbers = new int[5];`, or directly assign values with an array literal, like `int[] numbers = {1, 2, 3};`. It's crucial to remember that the size of the array is fixed once established. While you can use variables to determine the length during declaration, you can't change the size after initialization. Therefore, consider your needs carefully when creating an array.
Let's delve deeper into the world of arrays in Java, focusing on how they interact with the language's object-oriented nature. While arrays might seem like simple data structures, they're actually objects in Java, which means they have methods associated with them. This can be a point of confusion for newcomers as it doesn't always align with their initial understanding of arrays.
One of the first things you'll notice is the syntax for declaring an array. It's quite explicit: `int[] numbers`, clearly signaling that we're dealing with an array of integers. This clarity can be a lifesaver in larger projects where understanding data types is crucial.
Java arrays, unlike some other languages, are zero-indexed. This means the first element is accessed with index 0. It's a common design choice in programming, but it can be a stumbling block for beginners who might expect one-based indexing.
We need to be mindful of array size as well, since it's fixed once we declare the array. This means if we overestimate the size needed, we might end up with unused space. It's critical to be judicious about array size to avoid inefficient memory usage.
Another important detail is that arrays are homogeneous in Java – they can only hold elements of the same data type. This makes for good performance but limits flexibility. For scenarios where you need to mix data types, structures like ArrayLists are a better choice.
Let's talk about potential pitfalls. Trying to access an index that doesn't exist in your array will throw an `ArrayIndexOutOfBoundsException`. It's vital to understand this error as it highlights the importance of bounds checking to prevent crashes.
Now, what happens if you don't explicitly assign values to your array elements? Well, Java will initialize them based on their data type. Integers become 0, booleans become false, and objects get a null value. This automatic initialization can be helpful, but it's important to be aware of it since default values might not always be what you intended.
For multidimensional arrays, imagine arrays within arrays. They can even be "jagged," where each row can have a different number of columns. This provides a lot of flexibility for representing more complex data, but it can make dealing with them more complex, particularly when using loop structures.
Keep in mind that while arrays in Java don't allow their length to be changed after they're created, you can create a new array and copy elements if resizing is necessary. But this emphasizes why you might consider ArrayLists when you require a more dynamic solution.
Finally, there are several ways to initialize arrays. You can do it during declaration, use array literals, or assign values later on. Understanding these options will help you manage your arrays efficiently in Java.
Step-by-Step Guide Creating and Initializing Arrays in Java for Beginners - Allocating Memory for Arrays Using the 'new' Keyword
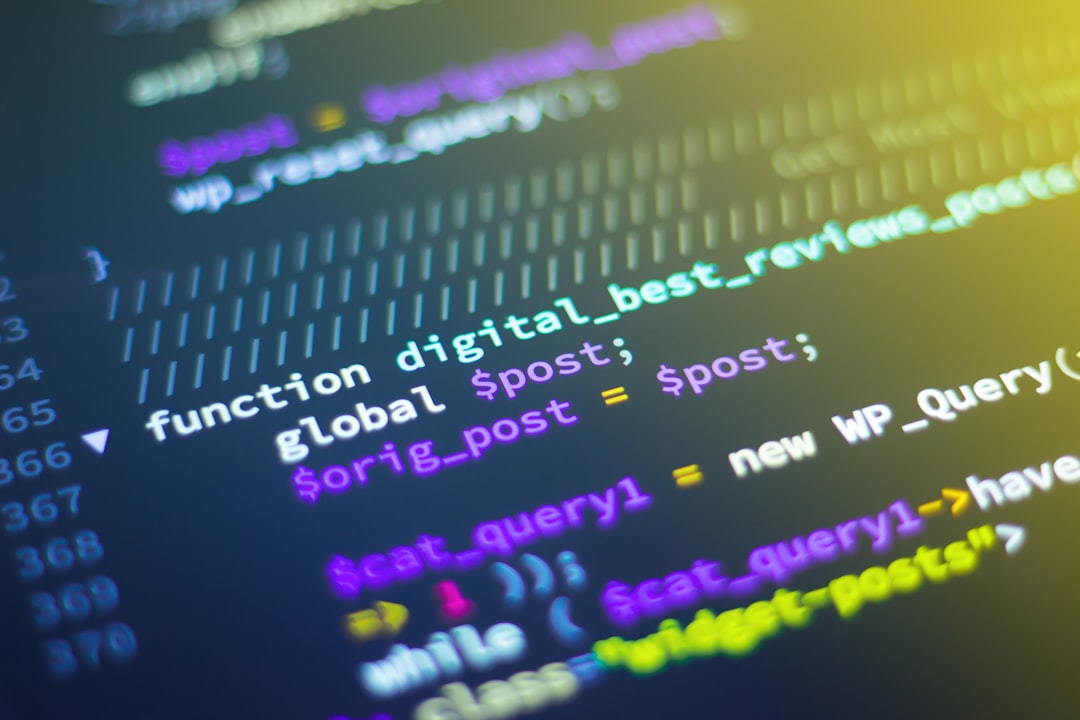
In Java, creating an array involves allocating memory using the `new` keyword. This tells the system to set aside a specific block of memory to hold the array elements. The size of the array is determined at the time you create it and cannot be changed later. For example, `int[] numbers = new int[5];` creates an array of integers called `numbers` with space for 5 elements.
It's important to note that if you overestimate the size of your array, you'll end up wasting memory. It's a good idea to estimate your needs carefully.
Java automatically sets the default values for array elements when you create them. So an array of integers, like `numbers` above, will be initialized with each element set to zero. If you have an array of objects, each element will be set to `null`.
While this might seem straightforward, understanding these points about how memory is allocated and initialized is crucial for beginners when learning to work with arrays in Java.
The `new` keyword in Java is the mechanism for creating arrays, which are fundamentally objects within the Java runtime. This allocation happens in the heap, a distinct region from the stack where local variables reside. When initializing an array, Java sets each element to its default value based on the data type. For instance, an integer array initialized with `new int[5]` will have all elements set to 0, which is helpful but can be deceptive if you assume an empty array. It's crucial to remember that arrays are objects and are subject to garbage collection, so if you replace an array with a new one, the previous array becomes eligible for garbage collection, impacting memory management in larger programs.
Arrays are known for their performance as accessing elements directly via an index takes constant time (O(1) time complexity). However, while this is a significant advantage, particularly for scenarios requiring rapid access, allocating memory for large arrays can lead to memory fragmentation. This can occur if the JVM struggles to efficiently allocate memory for new objects because of non-contiguous blocks of available memory.
The flexibility of multidimensional arrays comes with a twist: they can have different lengths for each "row," creating what's called a jagged array. While this adds flexibility, it also introduces complexities regarding memory management as each row may be stored in separate memory locations. Another key characteristic of Java arrays is that they are immutable in size. You cannot resize them once they are created. To get the length of an array, you use the `length` property. Attempting to initialize an array without the `new` keyword results in a compilation error, reminding us that explicit memory allocation is essential in Java's object-oriented structure. Arrays of objects are initialized with `null` by default, which can lead to `NullPointerExceptions` if not handled carefully. Finally, unlike some other languages like C where arrays can hold primitive types without object creation, Java requires object creation for arrays, which can impact performance and memory usage.
Step-by-Step Guide Creating and Initializing Arrays in Java for Beginners - Initializing Arrays with Default Values
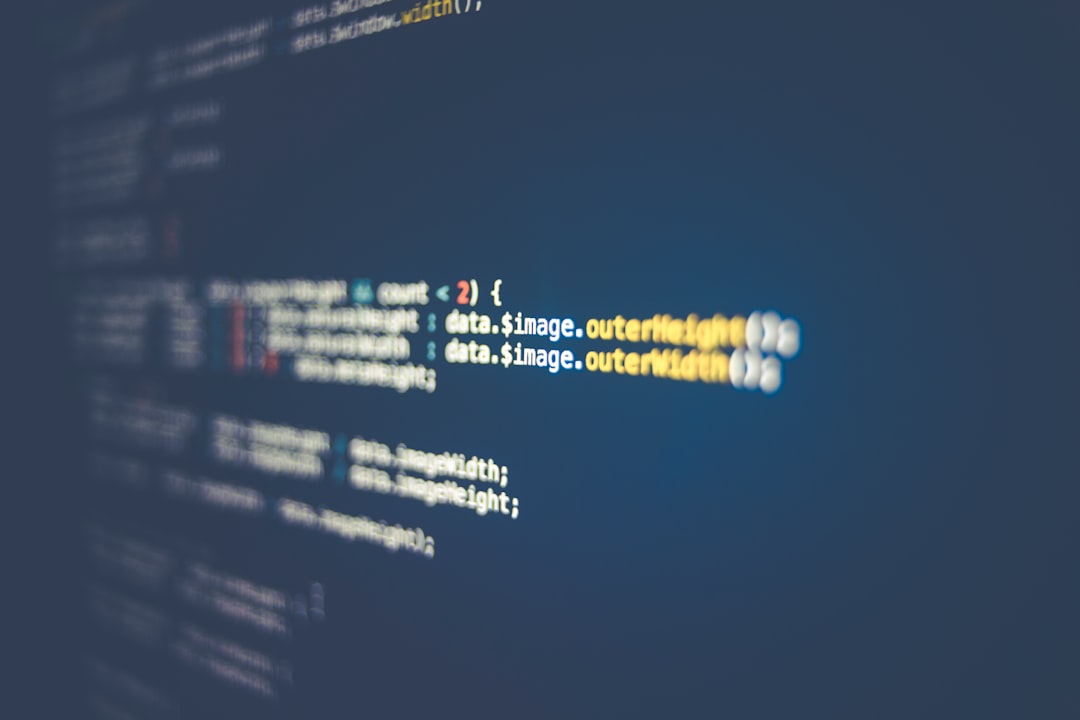
When you create an array in Java, it's automatically filled with default values based on the data type. For example, an array of integers will have all elements set to zero, boolean arrays will have `false` as their default, and object arrays will start with `null`. While this default initialization can be convenient, it's crucial to be aware of it. If you're not careful, you might end up with unwanted default values that could affect your calculations or operations. It's always a good practice to explicitly initialize your arrays with the values you intend to use, especially for arrays of objects. Understanding how default values work is essential for beginners when working with arrays in Java.
Understanding how Java initializes arrays with default values is crucial when working with these fundamental data structures. When you use the `new` keyword to create an array, Java doesn't leave it empty. Instead, it automatically populates each element with a default value depending on the data type: 0 for integers, 0.0 for doubles, `false` for booleans, and `null` for object references. This behavior can be deceptive as you might initially assume an array is empty, only to discover it's already filled with defaults, which can potentially lead to bugs if you don't consider this implicit initialization.
Beyond these initial values, Java allocates arrays in the heap memory, separate from the stack where local variables are stored. When an array reference is reassigned, the original array becomes eligible for garbage collection, freeing up memory resources. This dynamic memory management is a key part of Java's efficient memory handling.
One of the significant advantages of arrays is their fast access time. Accessing any element in an array has a time complexity of O(1), meaning it takes constant time to retrieve a value, no matter where it is in the array. However, when dealing with large arrays, allocating and managing memory for them can cause memory fragmentation. This occurs when the Java Virtual Machine (JVM) has to allocate new objects in non-contiguous blocks of free memory, which can hinder performance.
Java supports multidimensional arrays that can be "jagged," meaning each row can have a different number of elements. While this adds flexibility for representing complex data structures, it increases the complexity of memory allocation because each row might be stored in different memory locations, making management more challenging.
It's vital to remember that arrays in Java are immutable in size once they are created. This means you cannot resize an array after its initial declaration. To get the array's size, you can use the `length` property, which returns the number of elements. Attempting to initialize an array without the `new` keyword will result in a compilation error, a clear indication of Java's strict requirement for explicit memory allocation in its object-oriented environment.
Arrays of objects are initialized with `null` values by default, which can lead to `NullPointerExceptions` if you try to access or use an element without first assigning it a valid object. Unlike some languages like C, Java requires object creation for arrays, even for primitive types. This can impact performance and memory usage because of the overhead associated with creating objects.
Multidimensional arrays provide a way to structure data more efficiently, but their complexity increases with the number of dimensions, leading to more challenging code management. Additionally, these structures require numerous bounds checks during iteration, which can affect performance.
Finally, the importance of careful array size estimation cannot be overstated. Overestimating the size of an array leads to wasted memory, while underestimating forces you to create new arrays and copy data from the previous one, adding overhead and potentially impacting performance. As you become more experienced with arrays in Java, understanding these nuances of initialization, memory allocation, and performance characteristics will allow you to manage and use them efficiently within your applications.
Step-by-Step Guide Creating and Initializing Arrays in Java for Beginners - Single-Step Array Declaration and Initialization
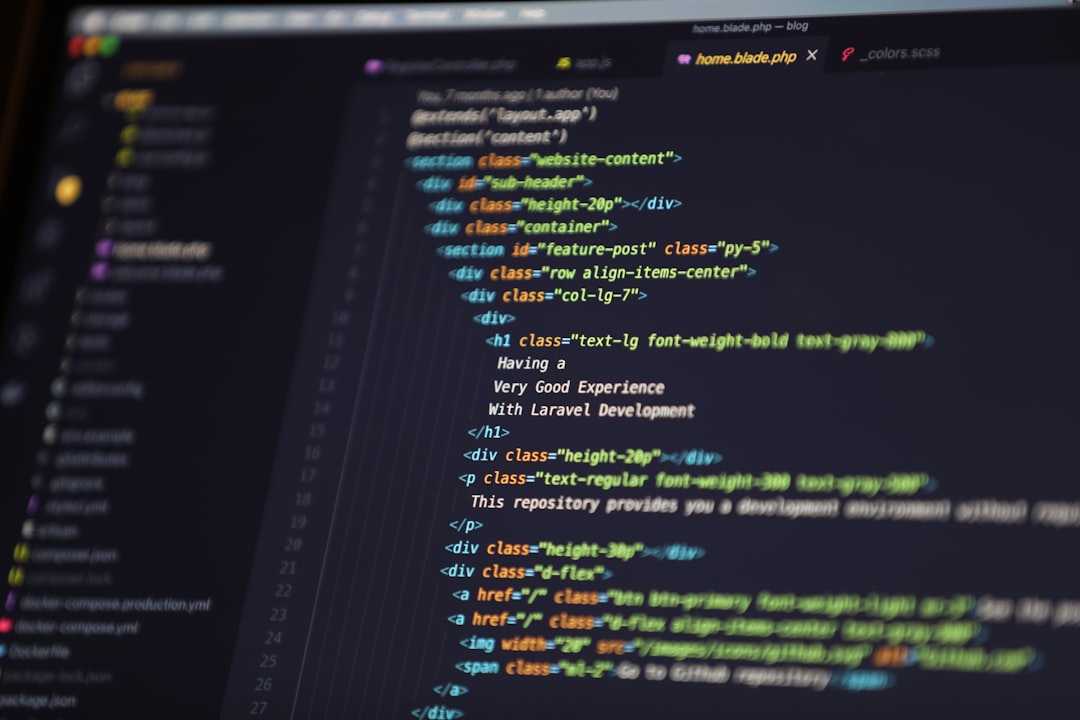
In Java, you can declare and initialize an array in a single step, making code more concise and easier to understand. This is done by directly assigning values to the array during declaration, like `int[] myArray = {1, 2, 3, 4, 5};`. This combines two steps into one, which is helpful for beginners learning to code. It's important to remember though that once an array is created, its size is fixed and can't be changed. Therefore, you need to consider the size you need carefully when using this method.
Let's dive deeper into the realm of array declaration and initialization in Java. While it might seem simple, there's a world of detail hidden beneath the surface. Single-step array declaration and initialization, where you both create and fill the array in a single statement, presents some interesting aspects.
First, remember that when you declare an array like `int[] numbers = {1, 2, 3};`, you're not just telling Java to set aside some space. Java actually goes ahead and immediately populates that space with the values you provided. This might seem trivial, but it can lead to performance gains, especially when dealing with large arrays. It's a bit like pre-packaging your groceries versus going to the store and filling your bags individually.
Second, Java, being the smart language it is, optimizes for these single-step initializations. It allocates the necessary memory and fills it in one go, making it more efficient. This is just one example of the optimizations Java performs under the hood to make your code run faster.
But let's get back to reality. We're not living in a world of pure optimizations. What if you want to be a little more flexible? You can use the `var` keyword to allow Java to infer the type of the array. This can help you avoid redundancy. Imagine using `var numbers = new int[] {1, 2, 3};` instead of explicitly stating `int[] numbers...`.
One crucial aspect is that when you initialize an array in this single step, you also get immediate access to the `length` property. This allows you to manage the array efficiently without any extra steps. It's as if you had a built-in ruler to measure your array's size right from the start.
However, we must remember that this single-step initialization also highlights the immutability of the array's size. You can't change the size of the array after its creation, which can lead to the dreaded `ArrayIndexOutOfBoundsException` if you try to access elements outside its bounds.
There are more subtle aspects to consider. What about multidimensional arrays? If you only initialize some parts of the array, the uninitialized elements retain their default values (0 for integers, `false` for booleans, and `null` for objects). This underscores the importance of complete initialization, especially when working with more complex arrays.
Java also makes sure that the data types you use for single-step initialization match the declared type of the array, doing the checks during compilation. This can help to avoid unexpected errors at runtime.
Finally, these arrays, like any other Java objects, are subject to garbage collection. So when you declare and initialize them in a single step, the garbage collector can keep track of their memory usage more effectively. This is a significant advantage for applications that work with numerous short-lived arrays.
The single-step initialization is not just a convenient shortcut; it reflects Java's underlying mechanisms for memory management and performance optimization. It also reinforces the essential concept of array immutability, highlighting the need for careful planning when working with these important data structures.
Step-by-Step Guide Creating and Initializing Arrays in Java for Beginners - Assigning Custom Values to Array Elements
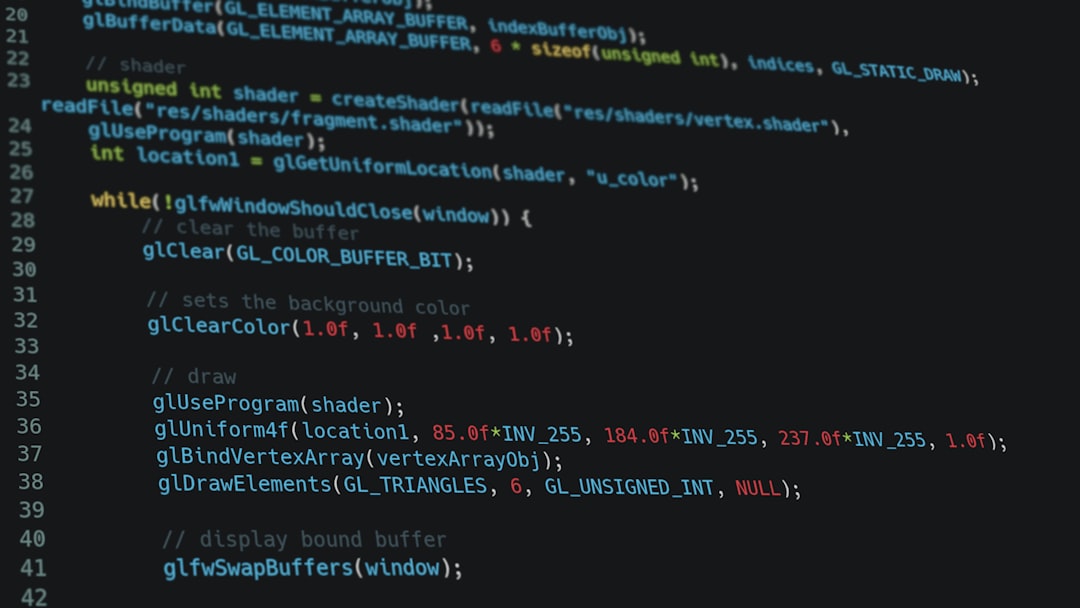
Assigning custom values to array elements in Java requires careful attention to ensure your program functions correctly. You can set these values during array declaration or later by using index assignment. For example, after creating an array of integers called `numbers`, you could assign specific values like `numbers[0] = 10;` and `numbers[1] = 20;`. Remember that array sizes are fixed once established. Attempting to access an element beyond this limit will trigger an `ArrayIndexOutOfBoundsException`. Therefore, it's crucial to plan your array sizes and carefully manage the assignment of values to elements to ensure your program's logic and data flow operate as intended.
Assigning custom values to array elements in Java is a fundamental concept that beginners need to grasp. While arrays are inherently simple data structures, understanding how they are initialized and interact with Java's object-oriented nature is crucial.
One of the key aspects of array initialization is type safety. Java ensures that your code won't compile if you try to create an array with mixed data types. This design choice prevents runtime errors and ensures that your data remains consistent.
However, when you declare an array using the `new` keyword, you are allocating memory in a contiguous block. This can lead to memory fragmentation if large arrays are frequently created and destroyed. If you aren't mindful of the memory allocation consequences, your runtime performance can be negatively impacted.
When you create a new array, remember that Java automatically initializes all elements with default values – 0 for integers, `false` for booleans, and `null` for objects. While this might seem helpful, forgetting about these initial values can lead to unexpected behavior. You should always initialize your arrays with the values you need.
If you use single-step array initialization, you have direct access to the `length` property, simplifying array management. This direct access makes it much easier to manage the array size, eliminating the need for additional declarations. This approach is also optimized for performance. Java can allocate memory and fill the array in a single operation, resulting in less overhead.
But there's a catch. Once an array is created, it's immutable, meaning that you cannot change its size. This limitation necessitates careful planning during development as attempting to access elements outside the defined range will result in the notorious `ArrayIndexOutOfBoundsException`.
Arrays of objects are a bit tricky since their elements default to `null`. If you're not careful and try to access them without initialization, you might get a `NullPointerException`. Always remember to handle null references properly!
Java also allows for jagged arrays – arrays where each row can have a different length. These structures provide more flexibility for data representation but can complicate memory management since rows may be allocated in non-contiguous blocks.
While Java uses garbage collection to automatically manage memory, keep in mind that frequent creation and destruction of arrays can increase garbage collection cycles, possibly impacting performance.
Finally, you can use the `var` keyword in Java to infer the type of the array. This feature can save you time and makes your code more expressive.
Arrays are a fundamental building block of Java programming, but as you gain more experience, you'll appreciate the intricacies and nuances of memory allocation, performance considerations, and garbage collection that are tied to array initialization.
Step-by-Step Guide Creating and Initializing Arrays in Java for Beginners - Accessing and Manipulating Array Elements
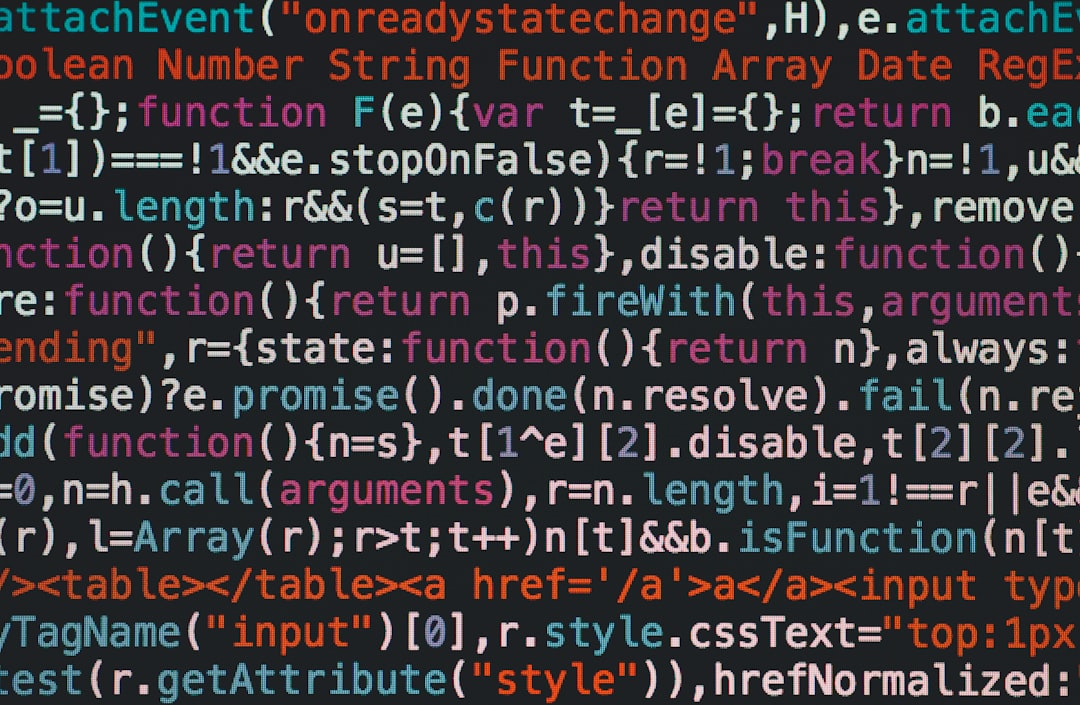
Accessing and manipulating array elements in Java is done using their indices, which are numbers that represent the position of each element within the array. These indices are crucial for both retrieving and modifying data. It's essential to remember that Java arrays are zero-indexed, meaning the first element is located at index 0. This can be a common source of confusion for beginners as they might expect the first element to be at index 1. Not understanding this can lead to errors like `ArrayIndexOutOfBoundsException`.
To access a specific element, you simply use the array name followed by the index in square brackets. For example, `arrayName[0]` would access the first element of the array. To change the value of an element, you can assign a new value to the corresponding index. For instance, `arrayName[0] = 10;` would change the value of the first element to 10.
An important thing to keep in mind is that arrays in Java have a fixed size. This means that once you declare an array, you cannot change its size afterwards. You have to decide the size of the array beforehand based on how many elements you will need. If you need a more flexible data structure, you should look into using a `List`.
The way you access and manipulate elements is fundamental to working with arrays. Mastering these concepts will give you a solid foundation for using arrays and enable you to handle data effectively in your Java programs.
Arrays in Java offer a way to store and manage multiple values of the same data type efficiently, but they come with their own quirks. They're fundamentally objects in Java, allocated in the heap memory, which can affect how garbage collection and overall memory management works. You'll often find yourself interacting with arrays in the context of object-oriented programming, adding another layer of complexity for beginners.
One key aspect that often trips up newbies is that Java arrays are zero-indexed, meaning the first element is accessed using index 0, not 1. This can lead to errors if you're used to other languages with one-based indexing. Also, Java initializes arrays with default values when they're created. Integer arrays start with all elements set to 0, booleans to `false`, and objects to `null`. This default initialization can be helpful, but it's important to be aware of it.
Another crucial point is that Java arrays are fixed in size after creation. This can lead to inefficiency if you overestimate the necessary size. While you can create new arrays and copy data if you need to resize, this can add overhead and affect performance. Also, keep in mind that Java arrays are type-safe, meaning they can only hold elements of the same data type. This can be restrictive, especially if you need to store diverse data.
Finally, accessing elements in an array is very efficient - it takes constant time, denoted as O(1) time complexity, meaning you can access any element quickly, regardless of the array's size. This performance is one of the main benefits of using arrays.
In summary, while arrays provide a convenient way to work with sequences of data, there are several things to consider, including the zero-based indexing, default values, fixed size, type safety, and efficient access time. Understanding these characteristics will help you leverage arrays in your Java projects effectively.
More Posts from :