Mastering JavaScript's String Manipulation A Deep Dive into the 'replace' and 'replaceAll' Methods
Mastering JavaScript's String Manipulation A Deep Dive into the 'replace' and 'replaceAll' Methods - Understanding the basics of JavaScript's replace method
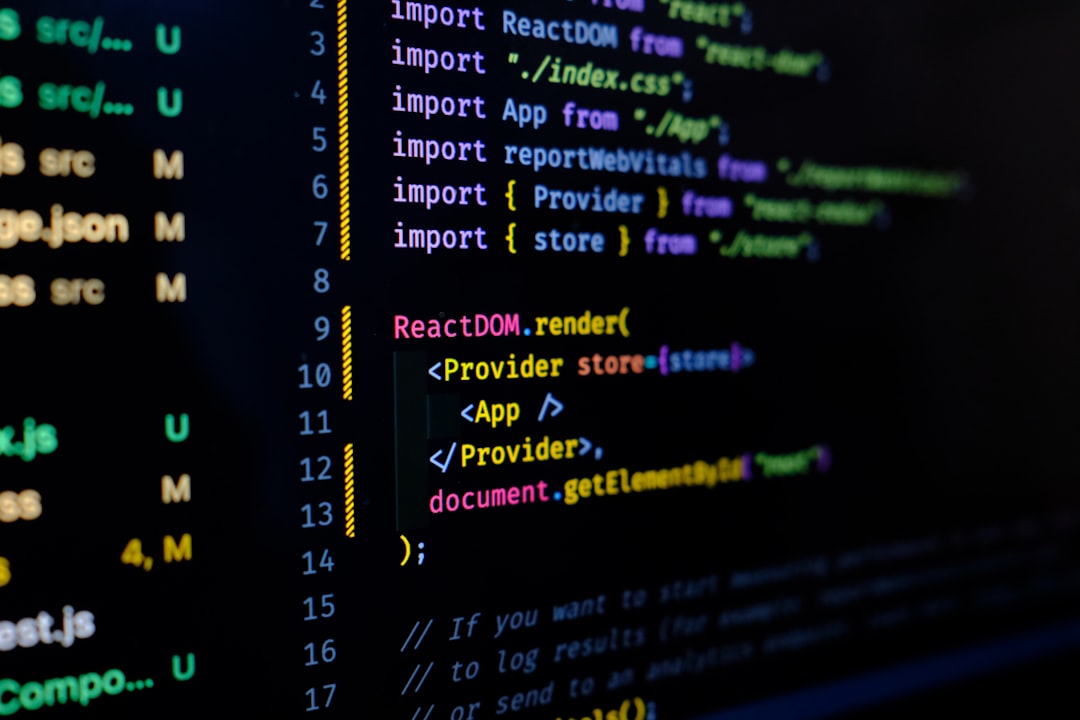
The `replace` method in JavaScript is a fundamental tool for manipulating strings. It operates by searching a string for a specific value or pattern, and then returns a new string with the matched occurrences replaced. While the `replace` method does not modify the original string, it generates a new one with the modifications.
An important aspect of `replace` is its behavior with string searches. If you provide a plain string as the search term, only the first instance of that string will be replaced. To target and replace all instances, you need to employ a regular expression with the global (`g`) flag.
The versatility of `replace` extends to its second argument. You can specify a string as the replacement value, or even a function. This function can dynamically determine the replacement based on the matched content.
Mastering the `replace` method is crucial for effectively manipulating strings in JavaScript. It enables operations such as formatting, sanitizing input, and modifying identifiable text within web applications.
The JavaScript `replace` method offers a powerful way to manipulate strings by searching for specific patterns and substituting them with new values. These patterns can be either plain strings or regular expressions, providing a remarkable level of flexibility in string transformation.
However, `replace` by default only alters the first occurrence of the pattern within a string. This can lead to unexpected behavior if you intend to replace all instances without using a specific technique.
To replace all matches, you need to use regular expressions with the `g` (global) flag, which underscores the importance of understanding regular expressions for effective string manipulation.
Importantly, `replace` doesn't modify the original string; instead, it creates a new string with the replacements applied. This principle aligns with functional programming paradigms and the concept of immutability.
The replacement value itself can be more than just a simple string. You can utilize placeholders like `$1`, which represent captured groups from regular expressions, allowing for dynamic replacements based on the matched patterns.
Furthermore, you can employ a function as the replacement parameter. This enables complex, dynamic substitutions, where the replacement value is calculated based on the matched content rather than being a static value.
While working with string patterns, remember that `replace` is case-sensitive when using strings as search patterns. "hello" won't match "Hello," potentially leading to bugs if overlooked.
The `replace` method is a fundamental tool for manipulating strings, particularly when working with data sources. It plays a crucial role in data cleansing and preprocessing stages, ensuring data is formatted correctly before further processing.
Importantly, `replace` can also handle Unicode characters effectively, vital in today's globalized software environment where text data can include various international scripts and symbols.
Despite its simplicity, `replace` can lead to performance issues if mishandled. Failing to optimize regular expressions or excessively chaining multiple `replace` calls can hinder application performance. Therefore, a careful approach to string handling is essential, especially when dealing with large-scale applications.
Mastering JavaScript's String Manipulation A Deep Dive into the 'replace' and 'replaceAll' Methods - Exploring the replaceAll method and its browser compatibility
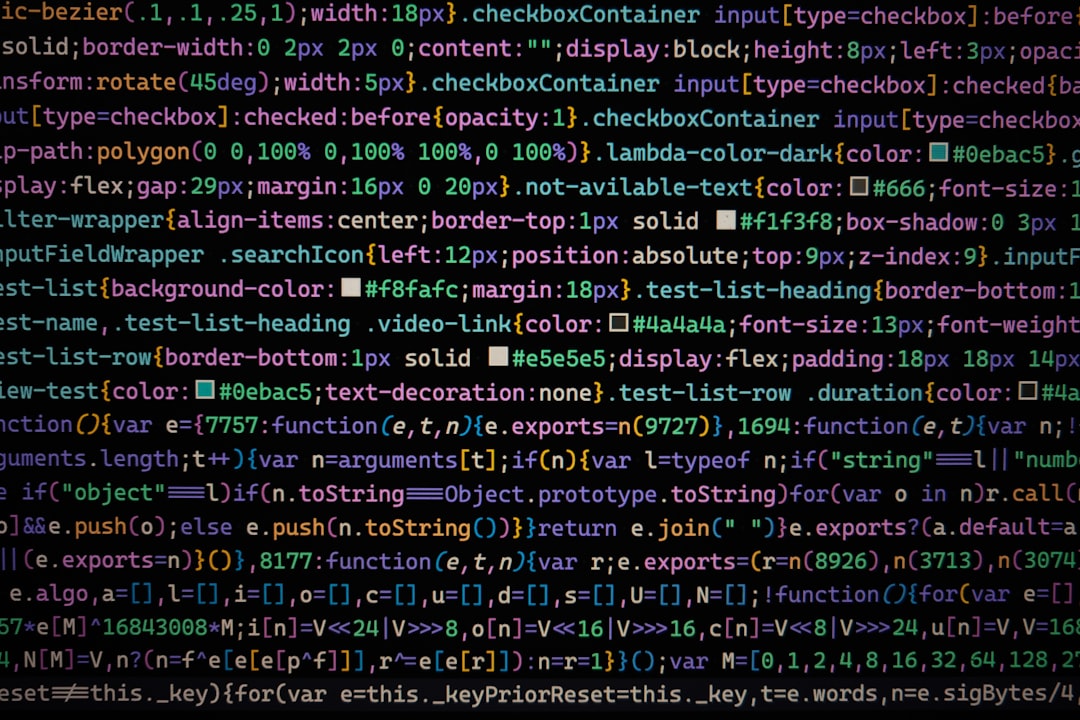
The `replaceAll` method, introduced in JavaScript as part of ECMAScript 2021, gives us a cleaner way to replace all instances of a substring within a string compared to the older techniques. This method, while simple to use, is case-sensitive and only returns a new string with the replacements made, leaving the original untouched.
You can use either a simple string or a regular expression to specify what you're looking for to replace. Importantly, when using regular expressions, you can't use the `g` flag, as `replaceAll` already handles replacing all occurrences. However, as with all new JavaScript features, you must be aware of browser compatibility. `replaceAll` is not supported by Internet Explorer, so you'll need to think carefully about your target audience if you use this method. By understanding these details, you can effectively use `replaceAll` to manipulate strings in your modern JavaScript code.
The `replaceAll` method arrived in JavaScript with ECMAScript 2021 (ES12), offering a new approach to replace all occurrences of a substring within a string. This addressed a common limitation of the `replace` method, which only replaced the first instance by default. With `replaceAll`, there's no need for the cumbersome approach of specifying a global flag (`g`) in regular expressions to handle multiple occurrences.
It's crucial to remember that `replaceAll` is only designed to work with regular expressions, unlike its predecessor. This means it doesn't accept plain strings as the first argument, ensuring that every instance is targeted for replacement. While this might seem like a restriction, it simplifies the process and removes ambiguity for the user.
However, `replaceAll` is case-sensitive by default, so if you're trying to replace "Hello" with something else, it won't affect instances of "hello" unless you explicitly handle the case difference. This reinforces the importance of carefully considering the case sensitivity in your string manipulations, especially when dealing with user input.
Browser compatibility, unfortunately, still presents challenges for this new feature. Modern browsers like Chrome, Firefox, and Edge have excellent support, but Internet Explorer and older versions often need polyfills to mimic the functionality. It's essential to always check compatibility and address potential issues for diverse user audiences.
While `replaceAll` simplifies the process, it's not without its quirks. It can lead to performance issues when handling large strings or numerous replacements, as it always generates a new string for each operation. This can significantly impact application speed and resource consumption. Additionally, handling special characters (such as `$` or `\`) requires additional caution, as `replaceAll` might not treat them as escape sequences unless wrapped in a regular expression, potentially producing unintended results.
One notable aspect of `replaceAll` is its adherence to immutability, like its counterpart `replace`. It doesn't modify the original string but instead returns a new one with the replacements applied. This aligns with best practices in JavaScript development and provides an added layer of safety when manipulating strings.
In the context of performance, the choice between using `replace` with a global regular expression and `replaceAll` depends on your specific use case. While using a single `replaceAll` call can be more efficient for replacing multiple occurrences, remember that if you're dealing with large strings or extremely performance-sensitive applications, it might be beneficial to explore other approaches.
Although `replaceAll` offers a simplified and convenient way to handle string replacements, it's important to recognize potential pitfalls. For developers unfamiliar with regular expressions, `replaceAll`'s implementation may lead to difficulties with code readability. Clear documentation and well-placed examples are essential to ensure efficient and reliable usage.
Mastering JavaScript's String Manipulation A Deep Dive into the 'replace' and 'replaceAll' Methods - Leveraging regular expressions for advanced string replacements
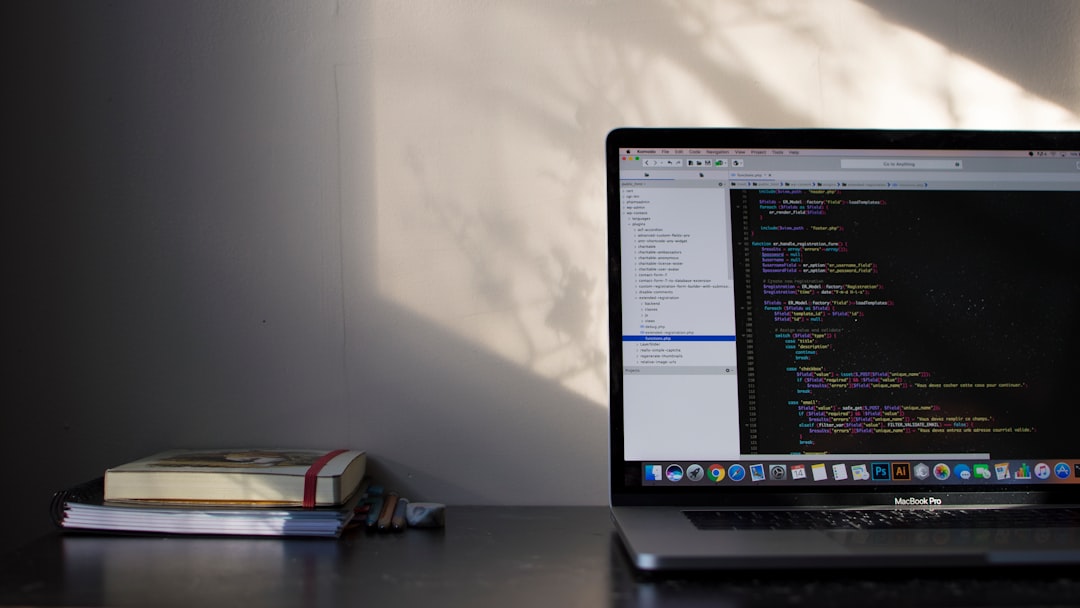
Leveraging regular expressions for advanced string replacements opens up a world of possibilities in JavaScript's string manipulation capabilities. While the `replace` and `replaceAll` methods provide fundamental tools, regular expressions empower us to go beyond basic replacements.
Regular expressions, often referred to as regex, allow for intricate pattern matching. This is especially valuable when you need to target specific elements within a string, like replacing only certain occurrences or substituting values based on matched content. Think of it like having a powerful magnifying glass for your text data, enabling you to locate and modify precise parts of a string.
The `RegExp` constructor allows you to create regular expressions on the fly, based on string patterns. This dynamic approach gives you more flexibility to adapt your string manipulations to the specific needs of your code.
Beyond simple replacements, regular expressions open the door to dynamic substitutions. You can use placeholders within the replacement string, such as `$1` or `$2`, to represent captured groups from your regex pattern. This allows you to insert different values based on what your regular expression has matched.
However, this added power comes with a responsibility to be mindful of browser compatibility. Some advanced features of regular expressions might not be supported by older browsers, requiring you to check your target audience and potentially use workarounds if necessary.
It's also crucial to consider performance, particularly when dealing with large amounts of text. Regular expressions, especially complex ones, can be computationally demanding, potentially impacting your application's speed. You'll need to balance the benefits of precise manipulation with the need for efficient code.
Overall, regular expressions are an indispensable tool for mastering JavaScript's string manipulation capabilities. They allow you to tackle complex tasks, replace text dynamically, and achieve sophisticated string processing, but require careful consideration for compatibility and performance to ensure the effectiveness of your code.
Regular expressions provide a powerful toolbox for manipulating strings beyond simple replacements. The `replace` and `replaceAll` methods in JavaScript can be utilized to perform advanced string replacements, but the full potential of regular expressions in this context often goes unexplored.
Here's a breakdown of some intriguing facets of regular expression usage for sophisticated string manipulation:
1. **Beyond Basic Matching**: Regular expressions excel at handling complex patterns, going far beyond just simple substring matches. Using `\d+`, for example, you can search for any sequence of digits, enabling you to extract and manipulate numerical data embedded within text.
2. **Capture Groups and Dynamic Replacements**: Capturing groups, using the syntax `(pattern)`, allow you to reference portions of a matched pattern in the replacement string using `$1`, `$2`, etc. This allows for rearranging or manipulating specific components of a matched text, significantly enhancing replacement capabilities.
3. **Context-Aware Replacements**: Regular expressions, when combined with nested pattern matching, can perform context-sensitive replacements. You could, for instance, modify a date format from `MM/DD/YYYY` to `YYYY-MM-DD` by individually matching groups, effectively performing a transformation without extensive conditional logic.
4. **International Text and Special Characters**: JavaScript regular expressions can handle Unicode characters, a crucial capability for applications dealing with multilingual text input. This ensures robust functionality across diverse languages and scripts, accommodating the need for globalization in software.
5. **Lookarounds for Precise Matching**: Advanced regular expressions leverage lookaheads (`(?=...)`) and lookbehinds (`(?<=...)`) to match patterns based on context without including that context in the match itself. This fine-grained control enables precise data extraction and replacements, furthering the depth of string processing capabilities.
6. **Performance Trade-Offs**: Regular expressions, especially complex ones, can be computationally demanding. When working with large datasets, poorly optimized regex can lead to performance bottlenecks. It's crucial to strike a balance between regex functionality and efficiency to avoid hindering application performance.
7. **Immutability and Safer String Manipulation**: Both `replace` and `replaceAll` adhere to JavaScript's principle of string immutability, generating new strings with replacements rather than modifying originals. This practice mitigates potential side effects and accidental modifications, particularly in larger codebases.
8. **`g` Flag and `replaceAll`**: While the `g` (global) flag is essential for `replace` to target all occurrences, `replaceAll` conveniently handles all instances without the need for the flag. This can simplify usage, but it may initially cause confusion for developers used to the `g` flag in `replace` operations.
9. **Cross-Browser Considerations**: Despite the adoption of modern standards, discrepancies in browser interpretation of regular expressions can still arise. Thorough cross-browser testing is crucial to guarantee consistent behavior in string replacements.
10. **Security Concerns**: Improperly handling user-generated input with string replacements using regular expressions can lead to security vulnerabilities such as injection attacks. It's essential to validate and sanitize input to prevent exploitation when employing regex-based replacements.
Regular expressions provide an unmatched level of flexibility and power in string manipulation. By embracing their intricacies and understanding the nuances of how they work with `replace` and `replaceAll`, JavaScript developers can build more robust, efficient, and secure applications.
Mastering JavaScript's String Manipulation A Deep Dive into the 'replace' and 'replaceAll' Methods - Handling special characters in search patterns
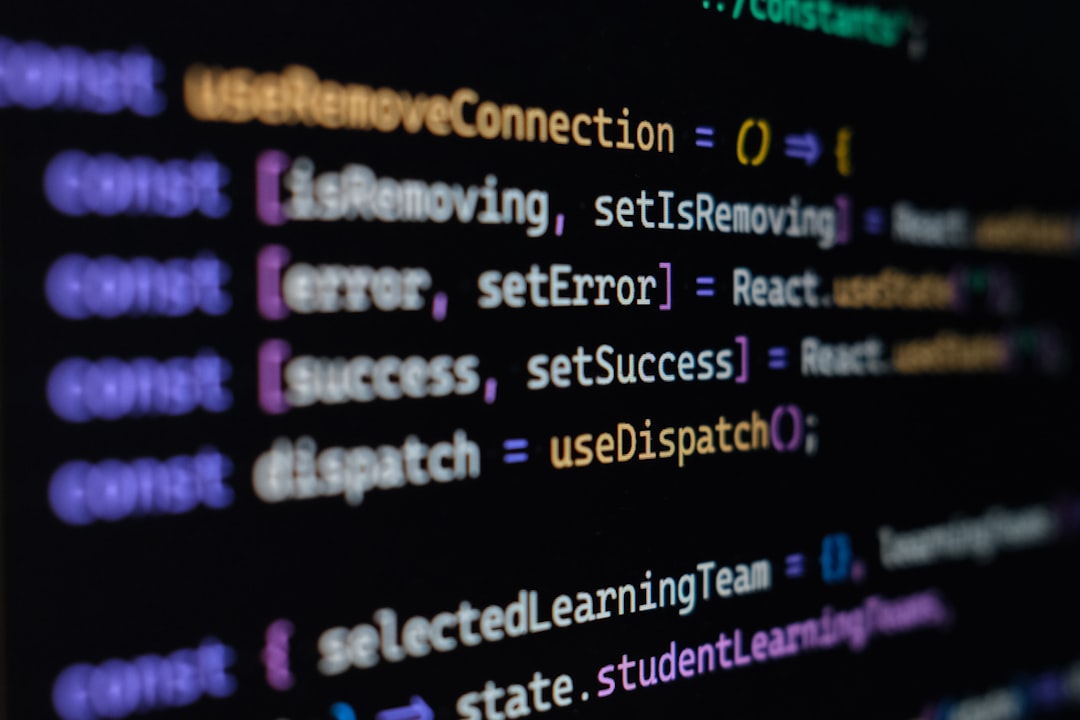
Handling special characters in search patterns is essential for working with JavaScript's `replace` and `replaceAll` methods. These characters, like `$` and `\`, have specific meanings within regular expressions. To avoid unintended consequences, you must escape them using a backslash. For example, if you want to replace a literal `$`, you'd use `\$` in your search pattern.
This is especially important with the `replaceAll` method, as it automatically replaces all instances. Unlike `replace`, it doesn't use the `g` flag in regular expressions. This can lead to confusion if you're used to working with traditional regex techniques.
Failing to properly escape special characters can result in syntax errors or incorrect replacements, leading to unpredictable behavior and bugs in your application. Understanding how these characters work with search patterns will improve the accuracy and efficiency of your string manipulation.
Handling special characters within search patterns in JavaScript's `replace` and `replaceAll` methods can be a bit of a head-scratcher, but it's a critical aspect of mastering these functions. Let's dig into some of the key points:
First, remember that in regular expressions, special characters like `*`, `+`, and `?` have specific meanings, acting as quantifiers or operators. If you want to treat these as literal characters, you need to escape them using a backslash (e.g., `\*`). Failure to do so can lead to unintended consequences in pattern matching.
Secondly, you need to consider case sensitivity. JavaScript's `replace` and `replaceAll` are case-sensitive by default, meaning they won't replace "text" with something else if you're searching for "Text." It's crucial to carefully manage case sensitivity or you'll end up with some unexpected results.
Next up is the concept of greedy vs. lazy matching, controlled by the `*` (greedy) and `*?` (lazy) quantifiers. Greedy quantifiers try to match as much text as possible, while lazy quantifiers prefer the shortest match. Your choice of quantifiers can significantly impact the outcome of your search patterns, so make sure you're using the one that aligns with your intentions.
Another fascinating feature in JavaScript is the use of lookbehind assertions. These allow you to match patterns based on what precedes them, without including that preceding text in your results. While this can be beneficial for complex replacements where context is important, the syntax can be confusing, especially for those new to regular expressions.
On the topic of international text and special characters, JavaScript's regular expressions have your back. Unicode support allows for recognition of special characters from diverse languages and scripts. The `\uXXXX` syntax enables precise matching of these characters, expanding the versatility of string manipulation in a globalized context.
However, there is a potential pitfall: double escaping. In JavaScript, special characters within regular expressions sometimes need to be escaped twice, once for the JavaScript string itself and once for the regex engine. For instance, a newline character (`\n`) would be represented as `\\n` in a regex pattern within a JavaScript string.
The `RegExp` constructor allows you to dynamically generate regex patterns. This offers flexibility in creating regex based on user input or variable data, but it also introduces the possibility of errors if the input is not properly validated or sanitized.
Now, let's address the issue of performance. Complex regex patterns can put a strain on performance, particularly when dealing with large strings. An inefficient regex can lead to long runtime delays, making optimization essential when designing your application.
Even though modern browsers mostly adhere to ECMAScript standards, some discrepancies can exist in regex interpretation across different browsers. This is especially true for advanced features, so rigorous cross-browser testing is crucial for consistent functionality.
Finally, keep in mind that when using regular expressions with user input, a lack of validation or sanitization can lead to security vulnerabilities. Attackers can use techniques like regex "rebinding" or malicious injections, making security vigilance paramount when dealing with user-generated data.
Overall, mastering special character handling within `replace` and `replaceAll` is essential for achieving accurate and robust string manipulation in JavaScript. Always remember to escape special characters correctly, carefully manage case sensitivity, understand the nuances of greedy and lazy matching, and be aware of the intricacies of lookbehind assertions. And, don't forget to prioritize performance, compatibility, and security when crafting your regular expressions.
Mastering JavaScript's String Manipulation A Deep Dive into the 'replace' and 'replaceAll' Methods - Case sensitivity considerations in string manipulation
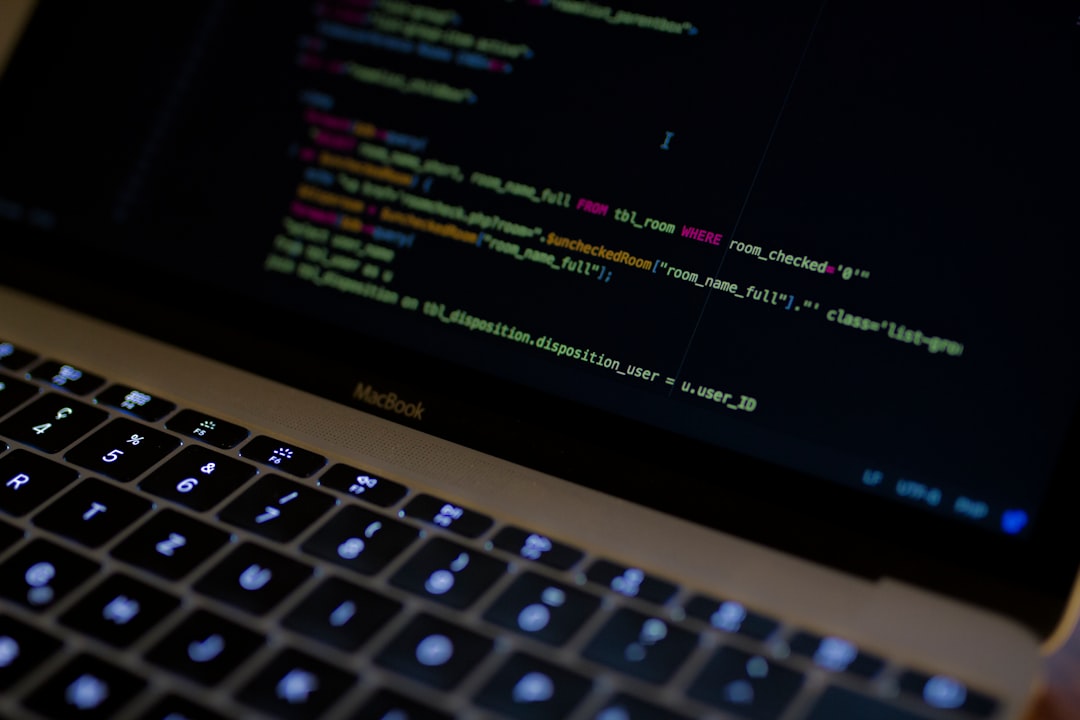
Case sensitivity is a fundamental aspect of string manipulation, particularly when using JavaScript's `replace` and `replaceAll` methods. These methods, in their default behavior, treat strings in a case-sensitive manner. This means searching for "text" will not match "Text" unless you explicitly address the difference in capitalization.
A simple solution to this limitation is converting strings to a consistent case before comparison or replacement. You could use `toUpperCase()` or `toLowerCase()` methods to ensure uniformity.
However, if you're working with regular expressions, you can use the `i` flag for case-insensitive matches, adding flexibility to your text replacements.
Neglecting case sensitivity can lead to unexpected bugs and inconsistent functionality in your application. Therefore, always carefully consider the impact of case sensitivity in your string manipulation code.
Case sensitivity is a tricky aspect of string manipulation in JavaScript. While the `replace` and `replaceAll` methods seem straightforward, the underlying case-sensitive nature can lead to some surprising results.
Firstly, by default, "apple" and "Apple" are considered distinct, potentially causing silent failures in code expecting a match. Secondly, addressing case sensitivity with regular expressions, particularly with complex patterns, can impact performance due to the added overhead.
Thirdly, the `/i` flag in regex is crucial for case insensitivity, yet often overlooked. Developers might assume case insensitivity without verifying, which can cause bugs.
Then, combining variables with string literals in different casing can result in unexpected mismatches, particularly when dealing with dynamic content.
Even cultural context comes into play. In internationalization, case transformations might behave differently based on specific language rules, like those in the Turkish alphabet, making cultural awareness essential for accurate string manipulation.
Unicode characters, too, add a layer of complexity. Case conversion for non-ASCII characters might not work as expected, potentially causing incorrect results or performance issues.
Case sensitivity also leads to potential bugs in password validation. A case-sensitive comparison can unintentionally lock users out or introduce security vulnerabilities.
Additionally, JavaScript's string immutability means new strings are generated with every case transformation or comparison, which can significantly increase memory usage, especially with large datasets.
Furthermore, different JavaScript engines might handle case sensitivity and Unicode normalization inconsistently, highlighting the need for thorough cross-browser testing.
Finally, data formats like JSON and XML often treat keys as case-sensitive. Failing to account for this can result in data retrieval errors, causing functional issues within applications.
So, while seemingly simple, case sensitivity in JavaScript string manipulation demands careful consideration to ensure accurate, performant, and secure code.
Mastering JavaScript's String Manipulation A Deep Dive into the 'replace' and 'replaceAll' Methods - Comparing replace and replaceAll performance across different scenarios
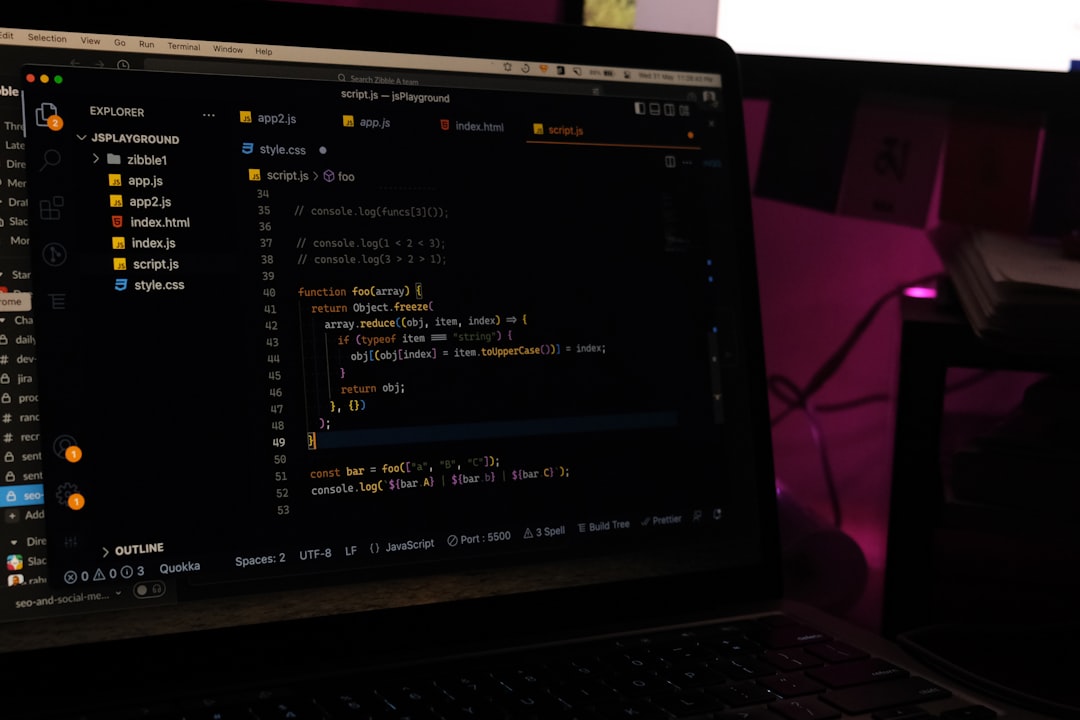
While both `replace` and `replaceAll` methods are designed for replacing parts of strings, the `replaceAll` method stands out by automatically replacing all occurrences without requiring a global flag, simplifying its use. Although performance tests show that there's no significant difference between these two methods in most cases, certain scenarios involving massive datasets or intricate patterns might favor one approach over the other. Developers need to stay alert for potential snags when working with these methods. Properly escaping special characters within patterns and being aware of case sensitivity are critical elements for accurate and efficient string manipulation. Understanding these aspects will be crucial for optimizing string handling within your modern JavaScript applications.
While `replaceAll` offers a more concise way to replace all occurrences of a substring, its performance can be influenced by a few factors. Firstly, for large strings with many replacements, `replaceAll` can be slower than `replace` with a global flag because `replaceAll` internally creates a new string for each replacement. This constant string creation can lead to performance overhead.
Secondly, the complexity of the regular expression used can also impact performance. Complex regex patterns can cause inefficiencies during execution, making them slower than simple replacements. The choice of regex engine, though JavaScript utilizes a highly optimized one, can influence performance, especially for intricate patterns.
Furthermore, both `replace` and `replaceAll` generate new strings, which can increase memory consumption. This is particularly noticeable when dealing with large strings or numerous replacements.
Beyond performance, the specific patterns used with `replace` can result in unexpected outcomes if they are not accurately defined. A greedy quantifier, for example, can unintentionally match broader segments than anticipated, causing unexpected replacements, even within massive datasets.
Also, keep in mind that both methods adhere to functional programming principles by not modifying the original string. While this offers safety and predictability, it can also affect execution speed, especially when performing multiple transformations on the same string.
Additionally, handling dynamic content can lead to noticeable performance drops. When continuously modifying strings based on user input or dynamic content generation, it's critical to optimize for intermediate states, as excessive use of `replace` or `replaceAll` can create noticeable lags.
Finally, using global patterns can introduce unforeseen edge cases, particularly when dealing with special characters. Failure to account for escape sequences can result in incorrect behavior, especially within a regular expression context.
It's important to be aware of the performance implications of using `replace` and `replaceAll` and to test thoroughly across different browsers to ensure consistent results.
More Posts from zdnetinside.com: