Kotlin JSON Parsing Troubleshooting the String to JSONObject Conversion Error in 2024
Kotlin JSON Parsing Troubleshooting the String to JSONObject Conversion Error in 2024 - Understanding the String to JSONObject conversion error in Kotlin
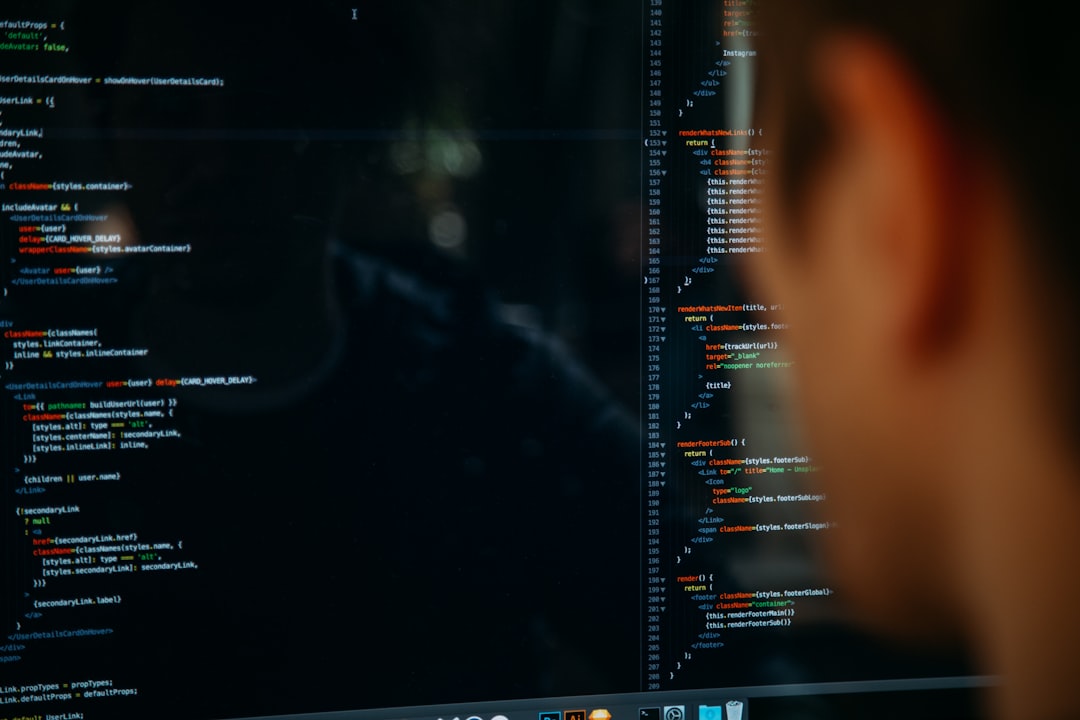
When converting a JSON string into a structured object like a JSONObject in Kotlin, things can go wrong if the string itself isn't formatted correctly. This often happens when the JSON string has syntax errors or isn't in a format the parser expects. Libraries like Gson and kotlinx.serialization have been developed to help make this conversion easier and less error-prone. However, even with these tools, you still need to be prepared for potential hiccups and handle exceptions appropriately to prevent crashes in your application.
Furthermore, defining data classes in Kotlin that mirror the structure of your JSON data plays a critical role in the conversion process. Essentially, you're providing a blueprint for the parser to understand how the JSON should be interpreted into Kotlin objects. Without this, you risk the conversion going awry, potentially losing data or producing unexpected results.
By understanding the potential for errors, leveraging the available libraries, and ensuring you've appropriately defined your data classes, you can significantly reduce the likelihood of encountering issues when converting JSON strings to JSONObjects within your Kotlin applications.
1. When converting a JSON string to a JSONObject in Kotlin, one frequent issue stems from a mismatch in data types. If a JSON string element isn't in the expected data format—for instance, an integer being treated as text—the conversion process will likely fail.
2. A common cause of conversion errors is having a JSON string with incorrect formatting. A single misplaced comma or bracket can trip up the parser, as it’s quite strict about adhering to JSON syntax rules.
3. JSON strings need to be encoded in UTF-8. If a string contains characters not properly encoded using this standard, conversion issues can arise. Making sure the input string is properly encoded is important to avoid these problems.
4. While JSON parsers are usually pretty tolerant of extra whitespace, unexpected spaces at the start or end of a string can lead to errors. It's a good idea to remove any leading or trailing whitespace before converting the string.
5. Kotlin has a strong type system, and using a nullable type where a non-nullable type is expected can lead to issues. Maintaining type consistency between Kotlin data classes and the JSON structure is crucial for smooth parsing.
6. Without proper error handling, unexpected crashes can occur, making it hard to understand why things went wrong. Using Kotlin's `try-catch` blocks during conversion helps diagnose and understand specific errors during parsing.
7. Different JSON parsing libraries like Gson and Moshi can have varying approaches to JSONObject conversions. It's beneficial to be aware of these differences to improve error handling and reliability during the parsing process.
8. When you work with Kotlin data classes, improperly setting default values can lead to unexpected null values after conversion. If not managed carefully, this can result in odd runtime behavior.
9. JSON parsing libraries might change how they work between different versions. An update to a library could introduce new behavior that leads to code that worked before throwing unexpected conversion errors.
10. Not testing your JSON conversion logic with unusual cases can lead to hidden errors. Using large strings, complex nested JSON structures, or input with rare characters can help find vulnerable parts of the parsing logic that might fail during real-world usage.
Kotlin JSON Parsing Troubleshooting the String to JSONObject Conversion Error in 2024 - Common pitfalls in JSON parsing with Kotlin in 2024
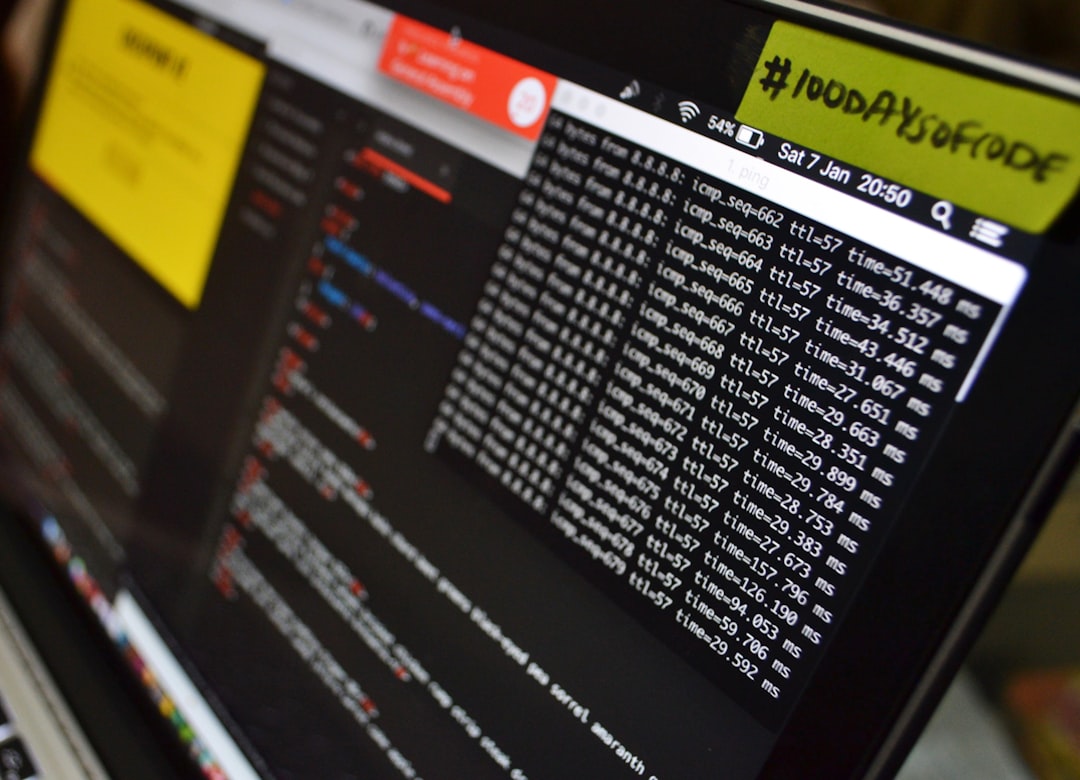
Navigating JSON parsing in Kotlin in 2024 presents a few common challenges. One key hurdle is ensuring that data types in the JSON string align with the expected Kotlin types. A discrepancy here can lead to difficult-to-diagnose conversion failures. Furthermore, JSON's rigid syntax means that even minor formatting mistakes like a misplaced comma can disrupt the parsing process and result in exceptions. Kotlin's robust type system also introduces its own set of pitfalls. Mismatches between nullable and non-nullable types in your Kotlin data classes and the JSON structure can lead to unwanted null values after conversion. Finally, the evolving landscape of JSON parsing libraries means that updates to these libraries can sometimes introduce new behaviors that affect previously functional code. Because of this, developers must thoroughly test their JSON parsing logic with a wide variety of inputs and include appropriate error handling to mitigate potential problems.
1. **Flexibility's Downside: Dynamic Schema Woes**. JSON's lack of a fixed structure can be a double-edged sword. If the incoming JSON doesn't match your Kotlin data model, it can lead to confusing and unpredictable runtime problems. You're essentially relying on the hope that the incoming JSON is in line with what your code expects, but there's no guarantee.
2. **The Booleans' Trickery**. JSON uses `true` and `false` for boolean values. However, issues crop up when these are improperly formatted, like being enclosed in quotes. Kotlin might not recognize them as boolean types, which can lead to issues when your logic relies on those boolean expressions. This can result in situations where true/false conditions aren't handled as expected.
3. **Decimal Points and Data Type Mismatches**. JSON typically uses floating-point numbers, but Kotlin provides several numeric types, such as `Long`. When these types don't align during conversion, you could lose precision or get unexpected conversion results. If your Kotlin code expects an integer type but receives a decimal, that's a prime example of where these errors occur.
4. **Escaping Woes: Unescaped Characters**. Certain characters in JSON strings, like quotation marks or backslashes, need to be escaped to avoid parsing errors. If they're not, it can create cryptic errors during the parsing process, adding another layer of difficulty to troubleshooting.
5. **Size Limits and Memory Pressure**. Kotlin's collection types, like Lists, have limits to how much data they can hold. Parsing very large JSON arrays can push these boundaries, potentially resulting in `OutOfMemoryError`. The worst part? These errors can pop up at runtime, making debugging and identifying the root cause challenging.
6. **Naming Discrepancies: Case Sensitivity**. Key names in JSON can have different conventions, like camelCase or snake_case, and these may not line up with your Kotlin property names. If these names aren't carefully aligned, conversion errors can pop up because the parser may not be able to find the corresponding property for a given key.
7. **Key Order Misconceptions**. While the order of keys in JSON objects is generally insignificant, some parsers may retain this order. This can create unexpected outcomes if your Kotlin code relies on a specific key sequence during the parsing phase, leading to logic errors.
8. **Deep Nesting and Object Structure Challenges**. Kotlin struggles a bit with complex, deeply nested JSON structures due to its flatter object model. This can make it harder to efficiently map the nested structure, requiring you to write more code for nested data classes which can be tedious to maintain.
9. **Default Values and Missing Data**: When JSON data is missing a key that your Kotlin data class anticipates, the default values you set may not be invoked correctly. This can lead to null references or unexpected behaviors in your application if it wasn't anticipated.
10. **Dealing with Extraneous Content: Parsing the Right Part**. If the JSON payload comes along with headers or other data before the actual JSON content, it could break your parsers. It’s important to isolate and pass only the JSON portion to the parser to prevent disruptions to the parsing logic. The parser is designed for valid JSON and can behave unexpectedly if fed something it's not expecting.
Kotlin JSON Parsing Troubleshooting the String to JSONObject Conversion Error in 2024 - Leveraging Gson library for efficient JSON string conversion
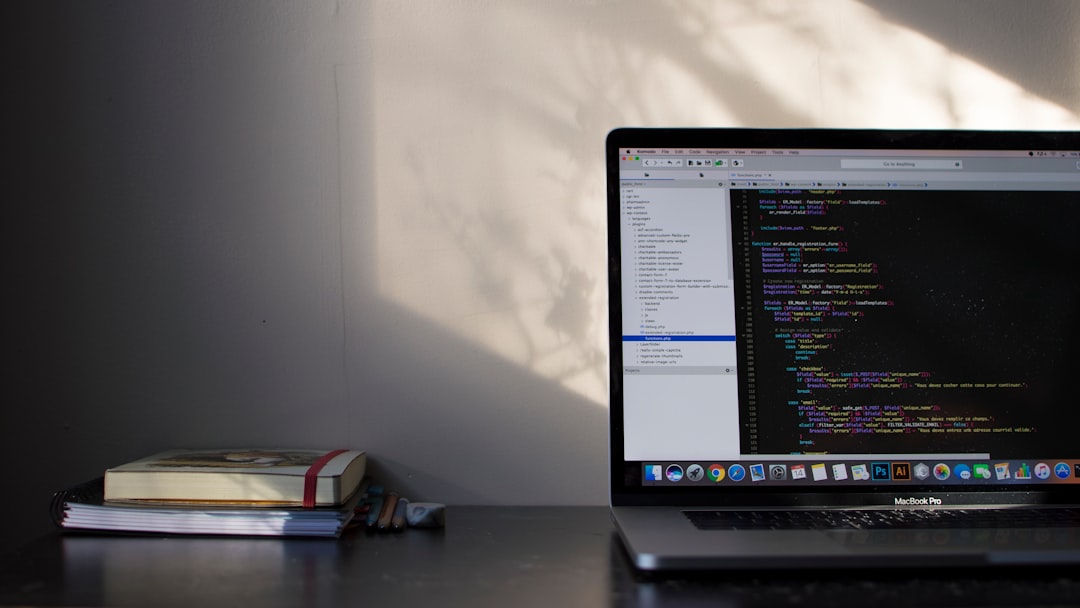
Gson is a valuable library within the Kotlin ecosystem for efficiently handling JSON string conversions. Its core strength is the ability to seamlessly translate Kotlin data classes into JSON strings and vice versa, making integration with external APIs and data sources much easier. This bidirectional conversion simplifies data manipulation and reduces the manual work involved in formatting or parsing JSON. Gson also offers flexibility in adapting to various JSON formats through features like the ability to customize the JSON keys associated with your Kotlin data classes using annotations. However, Gson's effectiveness relies on a firm understanding of its capabilities and potential issues. Errors during conversion can often be traced back to mismatches in data types or formatting errors within the JSON string itself. While Gson generally provides a convenient solution, developers should be aware of these pitfalls to ensure their JSON conversions are reliable. When used correctly, Gson is a crucial tool that boosts efficiency and reduces the likelihood of conversion errors when dealing with JSON in Kotlin applications.
Gson is a widely-used library in Kotlin for handling JSON, primarily for converting data between JSON strings and Kotlin objects. It's been a popular choice due to its performance and features. It utilizes reflection, allowing it to swiftly convert objects into JSON strings and vice-versa.
Gson excels at dealing with generic types, which is helpful when working with JSON arrays where maintaining the proper types is important. It avoids issues you can get when dealing with raw types. Furthermore, if you come across unusual JSON structures, you can create custom type adapters within Gson to tailor the parsing process. This is a powerful feature that lets you handle specific formatting requirements or data nuances.
Another useful feature is its ability to manage different naming conventions. It can help you overcome issues that might pop up because of mismatches between how your JSON data is structured and the way Kotlin data classes handle names. A common example of this would be camelCase vs snake_case for naming keys.
Gson also incorporates advanced methods for handling null values in JSON data. It allows you to configure how the library interprets missing keys, making it possible to prevent null-related exceptions.
If something does go wrong during parsing, the error messages Gson produces are often very helpful in identifying the source of the problem. They often contain specific details on what went wrong and where, which helps to pinpoint problematic sections of your JSON.
It has a streaming API, enabling you to parse huge JSON files more efficiently. Instead of loading the whole JSON into memory, which can lead to performance problems, it parses the data incrementally. This is particularly useful when dealing with large JSON documents that might cause problems when loaded into memory in a traditional way.
Gson is a library that keeps up with newer features in Java and Kotlin, including aspects like `Optional` and support for functional programming patterns. This means it integrates more seamlessly with other libraries or coding styles.
Gson also leverages caching mechanisms aggressively to keep track of data structures. This feature speeds things up when parsing the same structures repeatedly. It avoids needless computations. You can find performance gains in scenarios where your code might be processing similar JSON data many times.
Lastly, the library gives you control over what data is included or excluded. You can decide which data elements are serialized or deserialized using annotations. This helps in cases where there is sensitive data or when you need to optimize the JSON payload size for efficiency reasons.
Gson remains a relevant library for JSON parsing, but it's not without its quirks. Like any library, it has its own design and features that influence how it handles data. Developers need to keep this in mind as they work with the library.
Kotlin JSON Parsing Troubleshooting the String to JSONObject Conversion Error in 2024 - Implementing kotlinx.serialization for robust JSON object creation
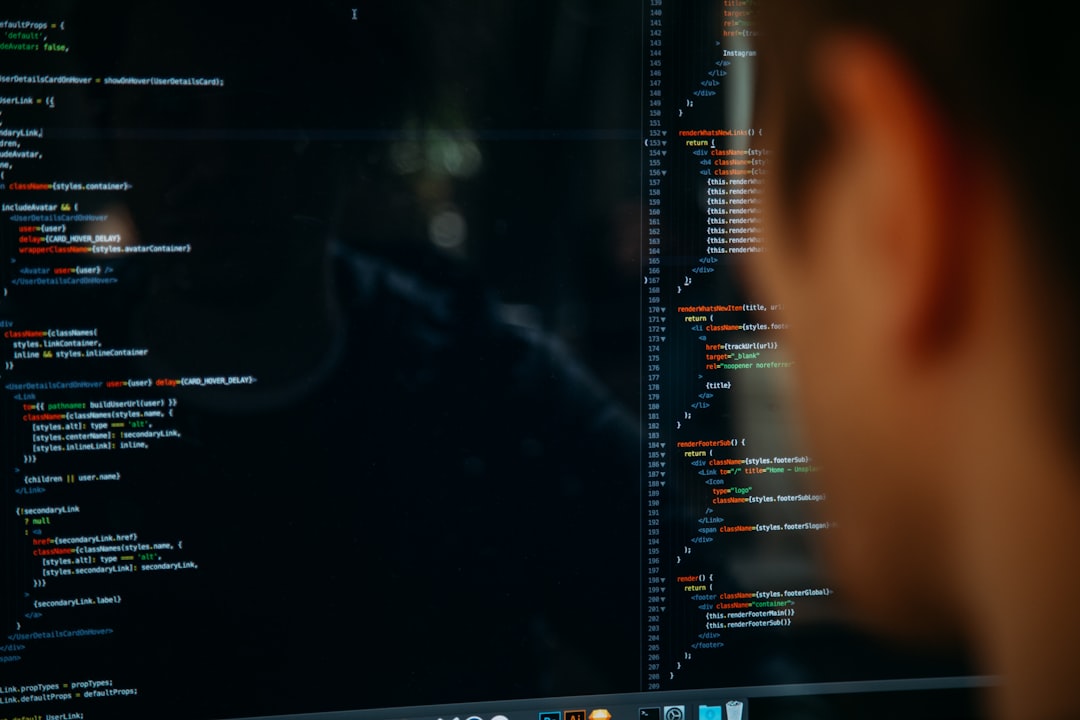
`kotlinx.serialization` simplifies JSON object creation and parsing in Kotlin. By annotating data classes with `@Serializable`, you can easily translate them to and from JSON format. Functions like `encodeToString` and `decodeFromString` streamline the process of converting between Kotlin objects and JSON strings, smoothing out the integration with various data sources and APIs. The `buildJsonObject` function empowers you to create JSON in a more controlled and structured manner, leading to a more streamlined development workflow. However, as with any parsing, it's critical to be mindful of possible data type issues and JSON formatting errors to prevent parsing hiccups. While `kotlinx.serialization` makes many aspects easier, these potential pitfalls still require attention.
Kotlin's `kotlinx.serialization` library provides a compelling approach to crafting JSON objects. It leverages annotations like `@Serializable` to define how Kotlin data classes are translated into JSON. Functions like `encodeToString` and `decodeFromString` handle the conversion process, making it relatively simple to move between Kotlin objects and JSON strings.
The `JsonObject` class, representing JSON objects as name-value pairs, is central to this process. We can construct these objects programmatically using `buildJsonObject`, giving us fine-grained control over the JSON structure.
One thing that makes it a bit easier to work with is that `kotlinx.serialization` integrates well with Kotlin's broader ecosystem, particularly the `Json` class. The `Json` class acts as a central point for configuring the serialization process, including registering custom serializers if needed. It's handy when you need to alter how JSON is generated or consumed. And, in situations where we're dealing with JSON parsing errors—common when trying to translate a string into a `JSONObject`—the library's features come in handy, helping us pinpoint issues related to JSON validity or serialization itself.
The `JsonElement` interface is valuable because it gives us a consistent way to work with various JSON elements, whether they're primitive types, arrays, or objects. Plus, since `JsonObject` implements the `Map` interface, we can leverage common methods like `get` or `getValue` to extract information, keeping data retrieval straightforward.
From a practicality standpoint, it's useful that `kotlinx.serialization` supports multiple platforms like JVM, JavaScript, and Native. It even expands beyond JSON to support other formats such as CBOR and Protocol Buffers. This platform independence is a big help for developers who want a consistent way to handle serialization in a variety of contexts.
While `kotlinx.serialization` offers a modern and powerful approach to JSON serialization, I've found that dealing with evolving data formats and deeply nested JSON structures requires careful planning and code adaptation. Sometimes, the process of keeping the Kotlin data classes and JSON data synchronized isn't always seamless, especially when JSON data is unpredictable. That being said, `kotlinx.serialization` is definitely worth considering as a potential tool for managing JSON interactions within Kotlin applications.
Kotlin JSON Parsing Troubleshooting the String to JSONObject Conversion Error in 2024 - Debugging techniques for JSON parsing issues in Kotlin applications
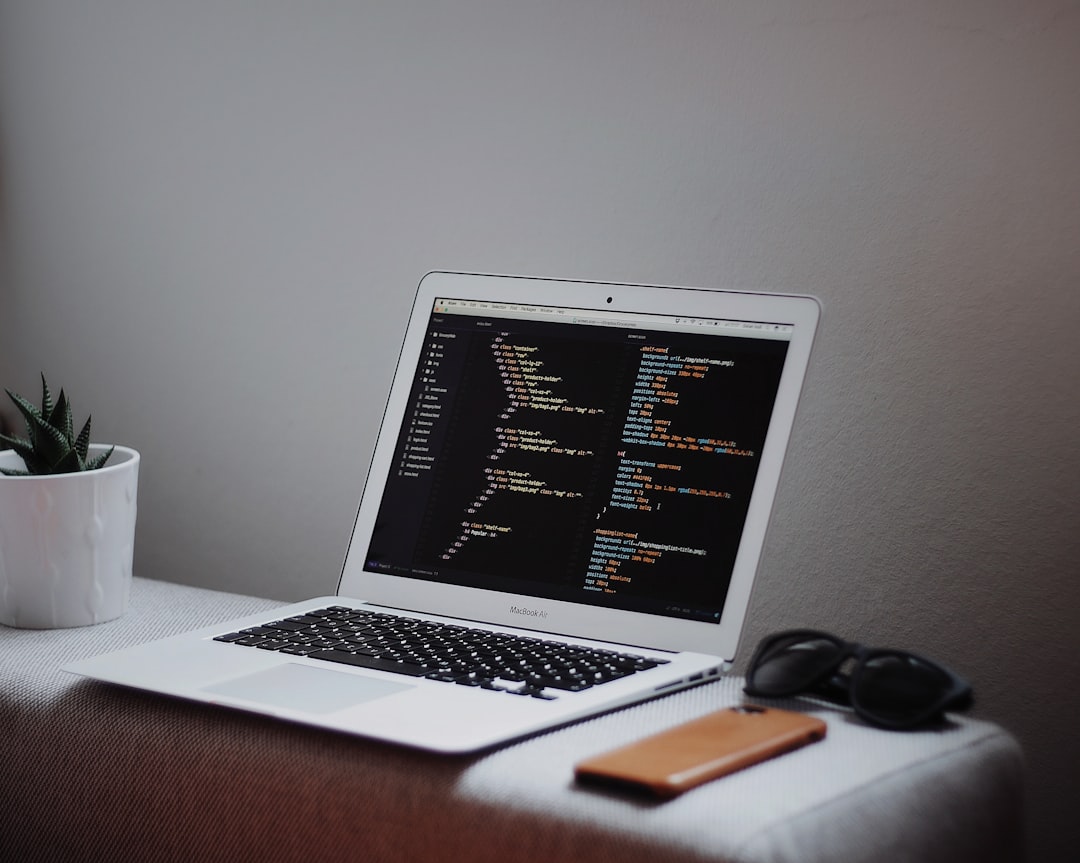
When tackling JSON parsing problems within Kotlin applications, employing smart debugging methods can help you find and fix issues more quickly. A common technique involves carefully comparing the JSON data's structure with the way your Kotlin data classes are designed. If there's a mismatch, like the JSON having a different data type than expected, this can cause the conversion to fail. You can also use logging to capture specific error messages during the parsing process. These error messages provide hints as to what's gone wrong. Additionally, it's crucial that the JSON string is free of typos or extra whitespace, since even small formatting errors can throw the parser off. By utilizing these techniques, developers can cut down on problems during JSON parsing, and create more robust applications.
When tackling JSON parsing in Kotlin applications, several debugging strategies can help you navigate the intricacies of converting strings into JSON objects. Libraries like Gson and kotlinx.serialization are crucial tools in this process, offering a path towards smooth conversions. However, even with these powerful tools, certain challenges remain.
One common hurdle is dealing with inconsistencies between the JSON structure and the anticipated Kotlin data class. If the JSON's schema unexpectedly deviates, you might encounter runtime errors that are difficult to diagnose. Moreover, minor formatting discrepancies within the JSON string, like a misplaced bracket or comma, can derail the entire parsing process, causing errors and throwing off your application's logic.
Another issue arises when JSON's boolean values aren't formatted as expected. If these values are presented as strings (e.g., `"true"`), Kotlin might fail to interpret them as booleans, which can lead to unforeseen consequences. Similarly, number formats can sometimes cause problems. JSON primarily uses floating-point numbers, but Kotlin offers various numeric types. Discrepancies in these types during conversion could lead to precision loss or strange results in your code, especially if you're expecting integers but get decimals.
Characters that require escaping within JSON strings can also trigger parsing failures. If these special characters aren't properly escaped, cryptic errors can occur. Managing the proper escaping of characters like backslashes and quotation marks becomes crucial in this context. Additionally, parsing extremely large JSON files can stretch memory resources to their limit, possibly leading to `OutOfMemoryError` issues. These can be frustrating to debug because they might only appear under specific circumstances and require deep analysis to track down.
The order of JSON object keys often isn't considered important, but some libraries might retain this order during parsing. If your code relies on a particular key sequence during the parsing phase, it can lead to unexpected outcomes. You can further encounter issues if your JSON data is missing certain keys that are expected by the corresponding Kotlin data class. In this case, the default values set in your Kotlin data classes might not behave as anticipated, resulting in null pointer exceptions or unexpected application behavior.
Furthermore, customizing how certain data types are parsed and serialized is essential. Custom type adapters in Gson can be helpful when the JSON structure doesn't adhere to standard formats. The regular update cycles of libraries like Gson or kotlinx.serialization introduce a new challenge—previously working code might break due to version changes. Developers need to be vigilant about compatibility as they upgrade the libraries they use. Deeply nested JSON structures can add complexity to Kotlin applications. Handling these structures may involve using numerous data classes or intricate mappings, requiring care and potentially leading to more maintenance work and a higher probability of errors.
These are just some of the debugging considerations you'll encounter while managing JSON string-to-object conversions in Kotlin. The combination of careful data class definition, robust error handling, and a good understanding of the intricacies of the libraries used during parsing can help significantly in navigating these potential issues. The better you understand the quirks of both Kotlin and JSON libraries, the better equipped you'll be to navigate these challenges and create more reliable and robust Kotlin applications.
Kotlin JSON Parsing Troubleshooting the String to JSONObject Conversion Error in 2024 - Best practices for handling complex JSON structures in Kotlin
Dealing with intricate JSON structures in Kotlin requires careful consideration to avoid common pitfalls during parsing and conversion. Libraries like `kotlinx.serialization` simplify the process by enabling you to define data classes that reflect the JSON structure and annotate them with `@Serializable`. This approach makes it easy to move back and forth between JSON and Kotlin objects. However, ensuring data types in your Kotlin classes align with those in the JSON is crucial; any discrepancies can lead to errors during the conversion. Complex JSON layouts, especially those with extensive nesting or unconventional formatting, can present unique challenges. In those cases, it can be very useful to utilize features like custom serializers or type adapters offered by libraries like Gson. Thoroughly testing your code with a range of input JSON is extremely important to catch problems. Additionally, incorporating proper error handling within your parsing logic is vital to prevent unexpected crashes and improve the overall reliability of your application. By focusing on these aspects, you can navigate even the most intricate JSON structures in a smooth and reliable way within your Kotlin applications.
1. **JSON's Flexibility Can Lead to Parsing Troubles**. JSON's ability to have different structures can cause problems when you're working with fixed data types in Kotlin. If the JSON you get doesn't match the format your Kotlin code expects, you can get runtime errors that are tough to track down. This can be frustrating, especially when you're dealing with data from sources outside your control.
2. **Type Mismatches Can Cause Unexpected Results**. Kotlin has a strong type system, but JSON doesn't always follow the same rules, particularly when it comes to numbers. If you have a mismatch between a JSON integer and a Kotlin float, you could lose some accuracy or end up with unexpected outcomes in your code. This is especially noticeable when you're doing calculations or comparing numbers.
3. **Default Values Can Create Unexpected Nulls**. If your JSON is missing some keys that your Kotlin data class is looking for, the default values might not get set the way you want. This can lead to `null` references that could cause problems later in your app. It's often not obvious where the issue started, making debugging trickier.
4. **Escaping Special Characters Is Key**. In JSON strings, characters like quotes and backslashes need to be "escaped" using special codes. If you don't do this correctly, the parser might get confused and throw errors. These errors can be difficult to understand, especially in larger projects with lots of JSON parsing.
5. **Parsing Huge JSON Files Can Be Memory Intensive**. When you're working with large JSON arrays, Kotlin's collection types might not be able to handle all the data at once. If you try to parse a file that's too big, you could get an `OutOfMemoryError`. These kinds of errors can be hard to fix because they often pop up at runtime and require some digging to find the source of the problem.
6. **Naming Inconsistencies Can Cause Parsing Errors**. JSON and Kotlin use different naming conventions for keys (like snake_case vs. camelCase). If these don't match up, you can lose data during the conversion process, and you might not always get clear error messages about what's wrong. This can create hidden issues that are hard to find unless you're carefully examining the conversion process.
7. **Key Order Can Be Surprising**. Generally, the order of keys in a JSON object doesn't matter. However, some libraries might keep track of the order. If your Kotlin code relies on the keys being in a specific order, but the incoming JSON doesn't follow that pattern, you could get errors in your logic that are challenging to find.
8. **Deep Nesting Adds Complexity**. Kotlin struggles a bit with complex, nested JSON structures because of its flatter object model. This means you'll likely need to use a bunch of different data classes and write custom code to handle the nesting, which can make your code harder to maintain and more prone to errors.
9. **Library Updates Can Break Things**. JSON parsing libraries get updated pretty frequently. Sometimes, these updates include changes that might break code that worked before. It's important to be aware of these changes and test your code after library updates to make sure everything still works.
10. **A Lack of Knowledge Can Lead to Parsing Issues**. Not everyone understands how JSON types relate to Kotlin types. This can lead to errors when converting data between the two formats. It's important for developers to have a good understanding of how these types work together, but sometimes documentation or good examples are not easily accessible which could hinder knowledge dissemination.
More Posts from zdnetinside.com: