JavaScript Array Manipulation 7 Efficient Methods to Remove Elements in 2024
JavaScript Array Manipulation 7 Efficient Methods to Remove Elements in 2024 - Efficient Array Splicing for Element Removal
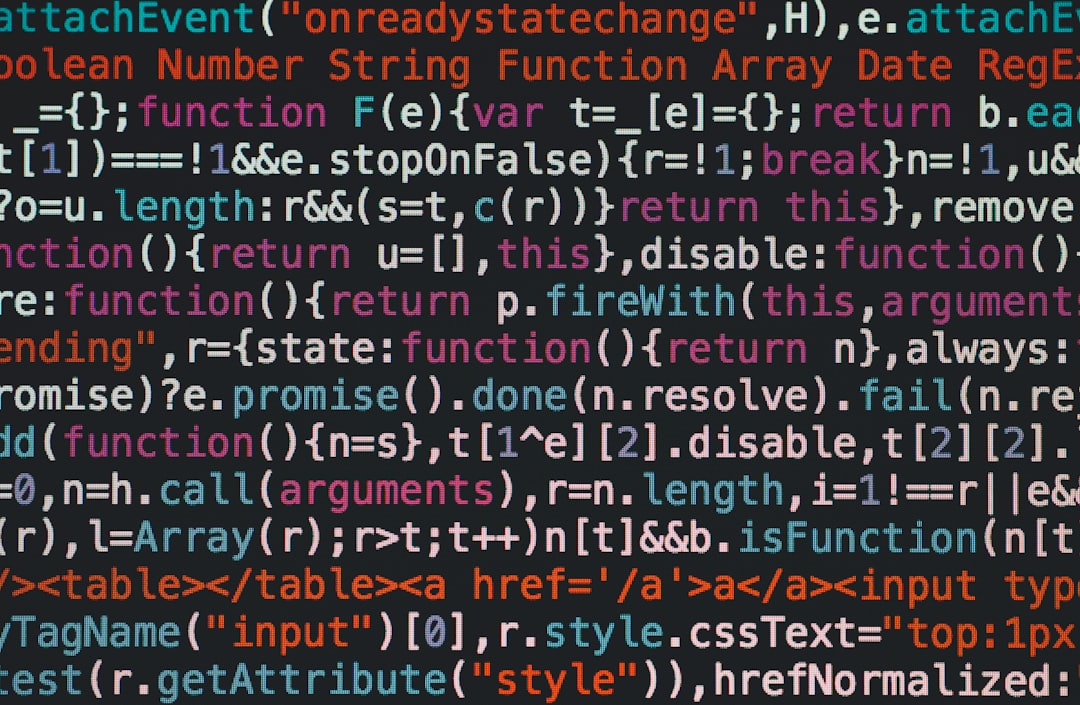
When it comes to efficiently removing elements from a JavaScript array, `splice` is a powerful tool. It offers precise control, letting you remove elements at any index, not just the ends like `pop` and `shift`. `splice` can even replace elements or insert new ones within the same operation, which can be helpful when dealing with dynamic data. However, while `splice` offers flexibility, the `delete` operator should be used with care. Deleting elements doesn't automatically reindex the array, leaving gaps and potentially creating a "sparse array" – an unexpected and inefficient outcome. As always, when choosing methods, it's important to balance code readability with the performance impact for the best possible approach.
JavaScript arrays, while seemingly straightforward, offer a world of intricacies when it comes to element removal. Efficient splicing isn't just about deleting elements; it's about understanding the underlying mechanics of array manipulation. A naive approach can lead to O(n) time complexity, particularly when dealing with large datasets.
`splice()`, while powerful, operates directly on the original array. This can lead to unexpected behavior if the array is being referenced elsewhere in your code. While it does save memory by avoiding the creation of a new array, potential side effects are worth considering.
Removing elements from the beginning of an array is generally less efficient than doing so from the end due to how memory is managed and the shifting of indices. This highlights the importance of understanding the internal workings of arrays for optimizing performance.
While traditional looping might be a common solution for element removal, functional techniques like `filter()` can offer a more concise and expressive approach. However, this comes at the cost of creating a new array.
It's worth noting that JavaScript engines implement optimizations that can significantly impact the performance of array operations. Understanding these optimizations can lead to more effective coding practices, but this is a complex area requiring further research.
The concept of "splice" stems from the underlying contiguous memory blocks used to implement arrays. Efficient manipulation requires understanding how these blocks are structured and how elements are accessed within them.
Destructuring assignment, a feature introduced in ES6, provides a more readable way to manipulate arrays, including element removal. However, it's crucial to use this feature with care to avoid unintended consequences.
Finally, a lack of understanding of asynchronous operations can lead to common pitfalls when dealing with array manipulation. Asynchronous operations can introduce complexities when arrays are modified within callback functions or promises. This aspect of asynchronous behavior requires further investigation and understanding.
JavaScript Array Manipulation 7 Efficient Methods to Remove Elements in 2024 - Filtering Out Unwanted Elements
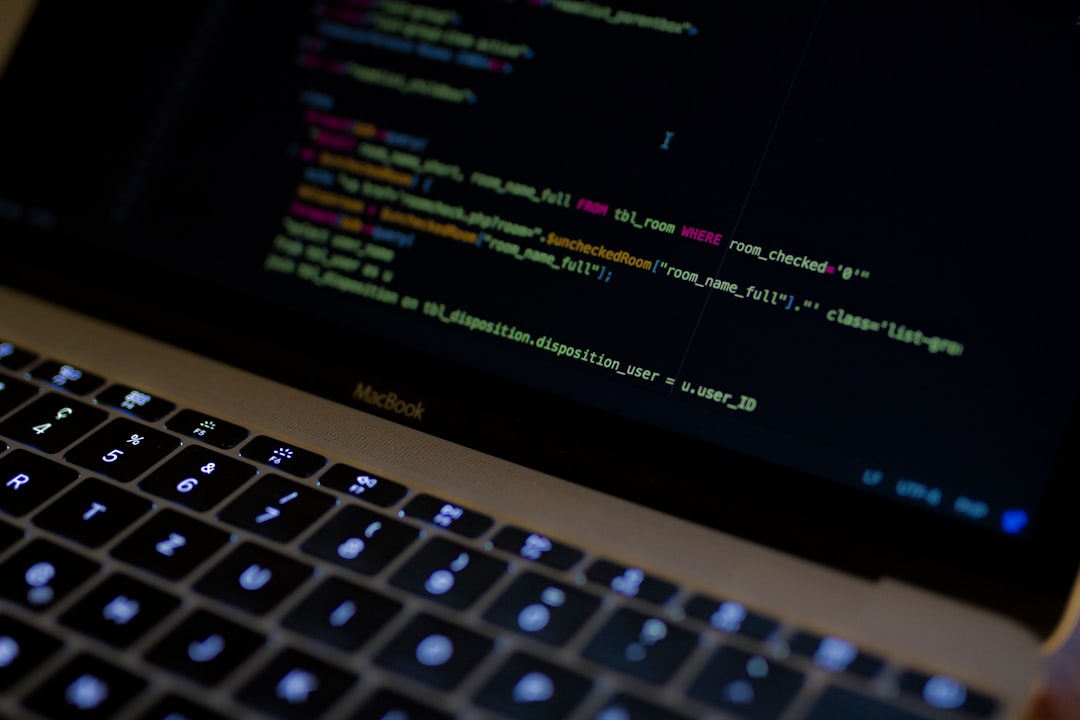
Filtering out unwanted elements from JavaScript arrays is a fundamental task in many applications. While you can manually loop through an array and selectively remove elements, JavaScript provides more efficient and elegant solutions. The `filter` method stands out as a powerful tool for this purpose. It generates a new array containing only the elements that pass a specified test, leaving the original array untouched. This is a safer and more readable approach than directly manipulating the original array.
However, there are other ways to remove elements. The `splice` method directly alters the original array by removing elements at a specific index. While this can be helpful in certain situations, it's essential to be aware of the potential side effects. Modifying the original array can lead to unintended consequences if the array is being used elsewhere in your code.
Libraries like Lodash offer additional methods such as `remove`, which operates similarly to `splice` but offers a more focused approach to removing elements that match a particular condition. Ultimately, choosing the right method depends on your specific needs and the desired outcome. Remember that a clear understanding of the trade-offs associated with each method is key to effective and efficient array manipulation in JavaScript.
When it comes to getting rid of elements in JavaScript arrays, there's more to it than just calling a method and expecting the best. The `filter` method, while straightforward, creates a fresh array. This means extra memory usage and potentially a slower run time, especially with massive datasets. On the other hand, methods like `splice` directly edit the original array, offering a potential performance advantage. But with this power comes responsibility. Careless use of `splice` can lead to side effects if the array is being used elsewhere in the code, causing unexpected problems.
Remember, even when you remove something, it's not instantly erased from memory. The garbage collector, like a recycling team, cleans up the unused spaces later. It's also important to understand the difference between a "sparse array" and a regular array. Using the `delete` operator without reindexing leaves holes in the array, making it less efficient. This can be particularly tricky when dealing with arrays containing millions of items.
One way to think about the process is the contrast between functional and imperative approaches. The functional approach, like `filter`, feels cleaner, but may have performance drawbacks. `splice`, on the other hand, gives you more direct control, but you need to be more careful to prevent issues.
There are also practical limits to consider. JavaScript arrays can be huge, but performance drops as they reach their maximum size. Adding or removing items at the end is usually quicker than manipulating the beginning or middle, due to how JavaScript stores arrays in memory. It's also worth remembering that modifying the default behavior of arrays can lead to frustrating bugs if you're not careful. And, structures like the `arguments` object are like arrays but lack some important features, making their manipulation a little more complex.
Overall, mastering the intricacies of JavaScript arrays is crucial for building efficient and stable applications. There is no one-size-fits-all approach when it comes to element removal. The best choice depends on the specific context of your application. Don't be afraid to get into the details and experiment, as the deeper you understand these complexities, the better equipped you'll be to tackle any array-related challenge.
JavaScript Array Manipulation 7 Efficient Methods to Remove Elements in 2024 - Popping Off the Last Element
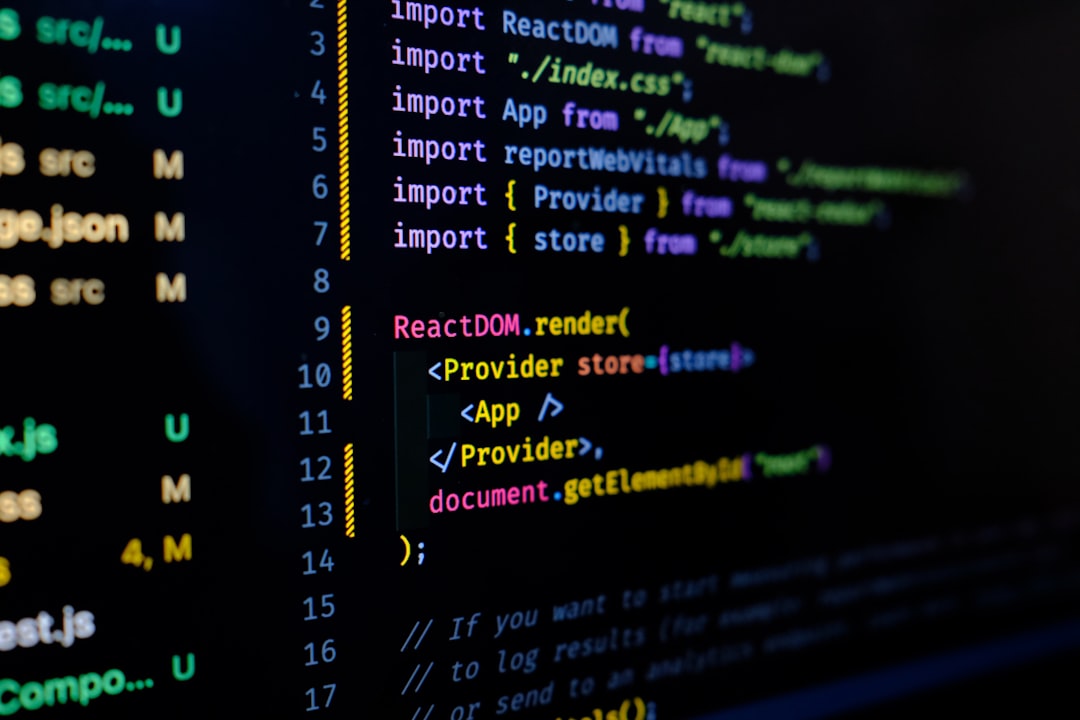
Removing the last element of a JavaScript array is a common task, and the most straightforward and efficient way to do so is using the `pop` method. This method does the job of both removing the element and returning it, effectively reducing the array's length without affecting the rest of the elements. While the `splice` method can be used to remove elements from any position in the array, it modifies the original array and might involve shifting elements, making it less efficient for single removals at the end. For a non-destructive approach, the `slice` method can be utilized to create a new array excluding the last element. However, remember that creating a new array, as opposed to directly modifying the original one, might come with performance implications, especially when dealing with large datasets. It's important to choose the appropriate method based on the specific requirements of your code and the size of the array. Understanding these options allows you to maintain both code readability and efficiency when manipulating arrays in JavaScript.
Popping off the last element in JavaScript might seem like a simple operation, but the "pop" method hides a surprising depth of complexity. While it's generally efficient, removing elements from the end of an array in constant time (O(1)), its performance can be affected by other factors like memory management and the data types within the array. The "pop" method's direct manipulation of the original array can also create unexpected side effects, particularly if that array is being used elsewhere in your code.
A critical aspect of "pop" is its non-functional nature. Unlike `filter()` or `map()`, which create new arrays and leave the original untouched, "pop" alters the original array directly. This can be a source of bugs in large projects if the array is shared between functions.
The "pop" method is also not immune to the quirks of sparse arrays. Using the `delete` operator, which removes an element without shifting the remaining elements, can create these arrays. This causes gaps in the array and can slow down future lookups. It's important to be mindful of how sparse arrays affect the behavior of the "pop" method and be prepared for potential performance implications.
Another important consideration is the asynchronous nature of JavaScript. Modifying arrays using "pop" within asynchronous operations can lead to race conditions if other actions happen simultaneously, which may disrupt the order of execution and result in unforeseen outcomes.
Finally, even though "pop" directly modifies the original array, the removed element is not immediately garbage collected. This means it remains in memory until other references are cleared, potentially impacting performance. It's a subtle point but one worth remembering when dealing with large datasets.
As always, the best approach to array manipulation depends on the specific context and goals. While "pop" might seem straightforward, its underlying complexities deserve a close look, especially when working with large-scale projects or performance-critical applications.
JavaScript Array Manipulation 7 Efficient Methods to Remove Elements in 2024 - Shifting Away the First Element
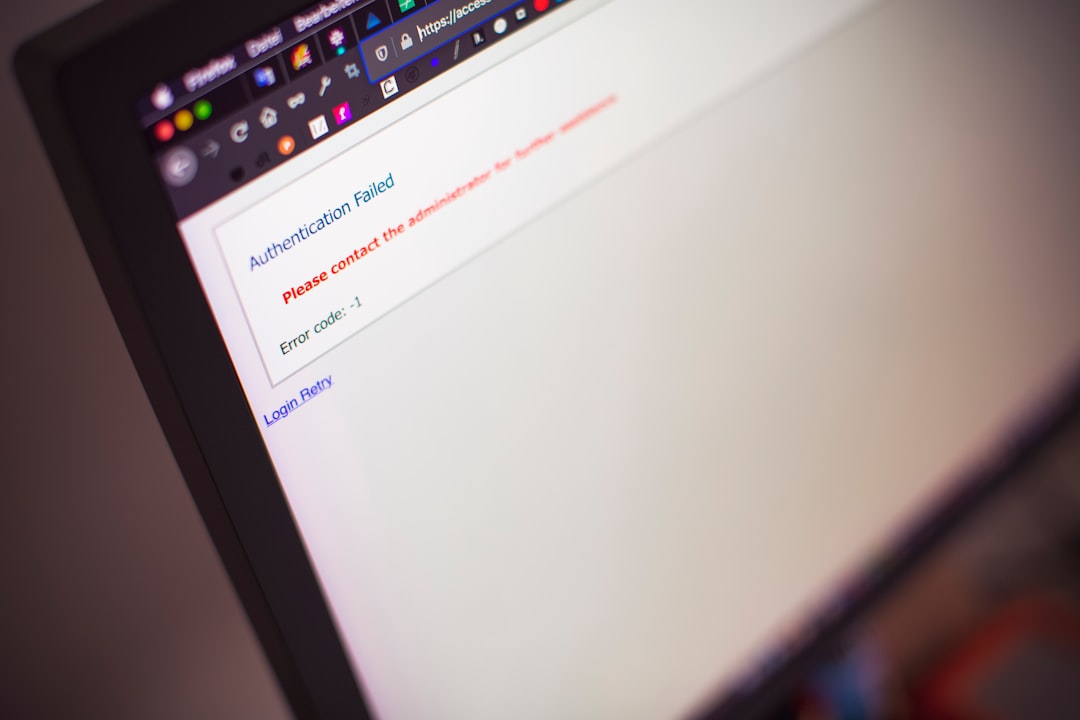
Removing the first element from a JavaScript array is a common operation, often handled by the `shift()` method. This method removes the element at index zero and returns it, modifying the original array in the process. However, `shift()` has a significant drawback: its time complexity is O(n), meaning the time required to perform the operation increases linearly with the size of the array. This is because all subsequent elements need to shift their positions to fill the gap left by the removed element.
While `shift()` is easy to use, it might not be the most efficient solution for large arrays where performance is crucial. Alternatively, the `splice()` method provides more control by allowing you to remove elements at any desired index within the array. However, `splice()` also modifies the original array, which can have unintended consequences if the array is used elsewhere in your code.
Ultimately, the choice between `shift()` and `splice()` depends on the specific needs of your application and the potential performance impact. Understanding these methods' strengths and weaknesses will enable you to choose the most efficient and appropriate way to remove the first element from your arrays.
Shifting away the first element in a JavaScript array using the `shift()` method might seem simple, but there are some surprising intricacies. Here's a look at ten key points to consider:
First, the time complexity of `shift()` is O(n), meaning it takes longer as the array grows. Why? Because every element after the removed one needs to be shifted to fill the gap. This makes `pop()`, which removes the last element in O(1), a faster choice if removing from the end of the array. Second, the nature of JavaScript arrays as contiguous memory blocks means that shifting elements over is not just about discarding a value, it can lead to memory fragmentation, potentially affecting performance.
Third, `shift()` returns the removed element, which can be helpful in certain scenarios. It allows you to directly use the value without additional variable assignments, unlike `splice()`.
Fourth, just like `splice()`, `shift()` mutates the original array. If you're working with shared references, unexpected changes can arise, making proper scope management crucial.
Fifth, be mindful of sparse arrays. Using `delete` to remove elements leaves undefined values, creating potential issues for `shift()`, as it reindexes the remaining elements, potentially causing gaps and slowdowns.
Sixth, for massive datasets, repeatedly using `shift()` can significantly impact performance. Using queue-like structures, designed for this purpose, might be a better choice in such situations.
Seventh, it's crucial to understand that while `shift()` can be computationally expensive, modern JavaScript engines employ various optimizations. These optimizations might mask the true cost, so profiling is vital to understand the impact in real-world scenarios.
Eighth, when working with an empty array, `shift()` returns `undefined`. Failing to account for this behavior can introduce subtle bugs, making robust error handling essential.
Ninth, if you favor a functional programming approach, creating a new array without the first element using the spread operator or `slice(1)` might be preferable, preserving immutability and preventing unintentional side effects.
Tenth, remember that while `shift()` is a valid option, alternatives like `slice(1)` can offer a more functional, clear, and potentially faster approach depending on your context, although they might introduce a slight memory overhead.
In conclusion, understanding these nuances about `shift()` is vital for building efficient JavaScript applications. Being aware of potential performance issues, memory implications, and alternatives helps us make more informed decisions when manipulating arrays.
JavaScript Array Manipulation 7 Efficient Methods to Remove Elements in 2024 - Flattening and Mapping for Complex Removals
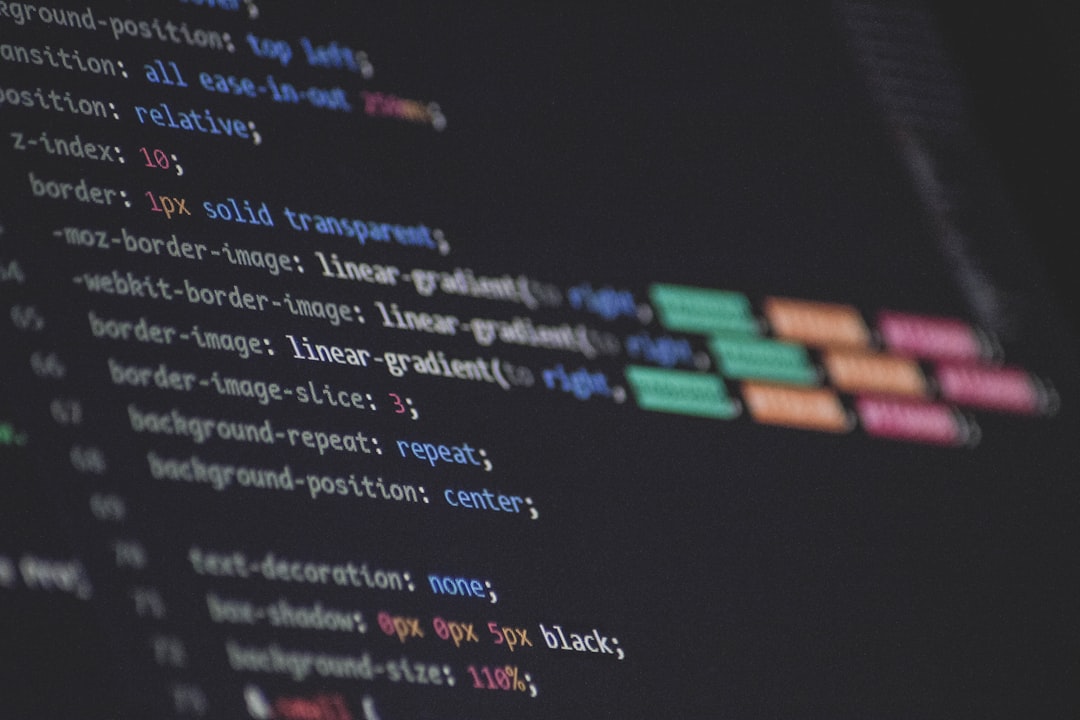
### Flattening and Mapping for Complex Removals
When you're dealing with complex data structures like nested arrays, JavaScript's `flat` and `flatMap` methods become your allies. `flat` helps you untangle these nested arrays, creating a simpler, single-level array for easier access and manipulation. This is especially useful when working with data from APIs, where multi-dimensional data structures are common. `flatMap` takes things a step further, allowing you to both transform and flatten the data simultaneously. This makes it incredibly useful for applying operations and reshaping your data in a single step. While powerful, remember that unnecessary flattening can lead to performance issues, especially when dealing with large datasets. The key is to use these methods strategically to optimize your code for clarity and efficiency.
Flattening and mapping can be powerful tools for complex removals in JavaScript arrays. Flattening transforms a nested array into a single level, streamlining element identification for removal. However, it's important to be aware of the performance implications. Flattening large nested arrays can lead to O(n) complexity, meaning the processing time increases with each level of nesting, which can become problematic in performance-sensitive applications.
Combining mapping functions with flattening adds flexibility. Using functions like `map()`, we can transform array elements based on specific criteria before flattening. This allows us to tailor the removal process to our specific needs and optimize the array's state directly. Deeply nested arrays might require recursion for flattening, but it's essential to carefully manage stack depth to avoid performance issues.
ES6's spread syntax provides a clean and immutable way to flatten arrays, avoiding direct mutations of the original array. This approach aligns with functional programming practices, promoting code readability and preventing unintended consequences. Combining `filter()` with flattening can also be effective. Filtering out elements before flattening can optimize the removal process by eliminating unnecessary elements earlier on.
However, keep in mind the potential memory overhead associated with flattening. Creating new arrays during flattening can increase memory usage, especially when used in loops or large-scale data processing. Additionally, it's important to manage the potential creation of sparse arrays during the process. Deleting elements while flattening can leave gaps in the array, leading to inefficient lookups and performance degradation.
For complex removals, libraries like Lodash offer specialized functions for flattening and array manipulation that can sometimes outperform native JavaScript methods. They can provide enhanced performance and readability when working with large datasets.
Finally, combining flattening and mapping with asynchronous programming requires careful consideration. Race conditions can occur if operations are modified asynchronously, potentially leading to inconsistent results due to changes in the array's state before the operation completes. Proper synchronization techniques are crucial for maintaining data integrity in such scenarios.
JavaScript Array Manipulation 7 Efficient Methods to Remove Elements in 2024 - Lodash Remove Method for Direct Array Modification
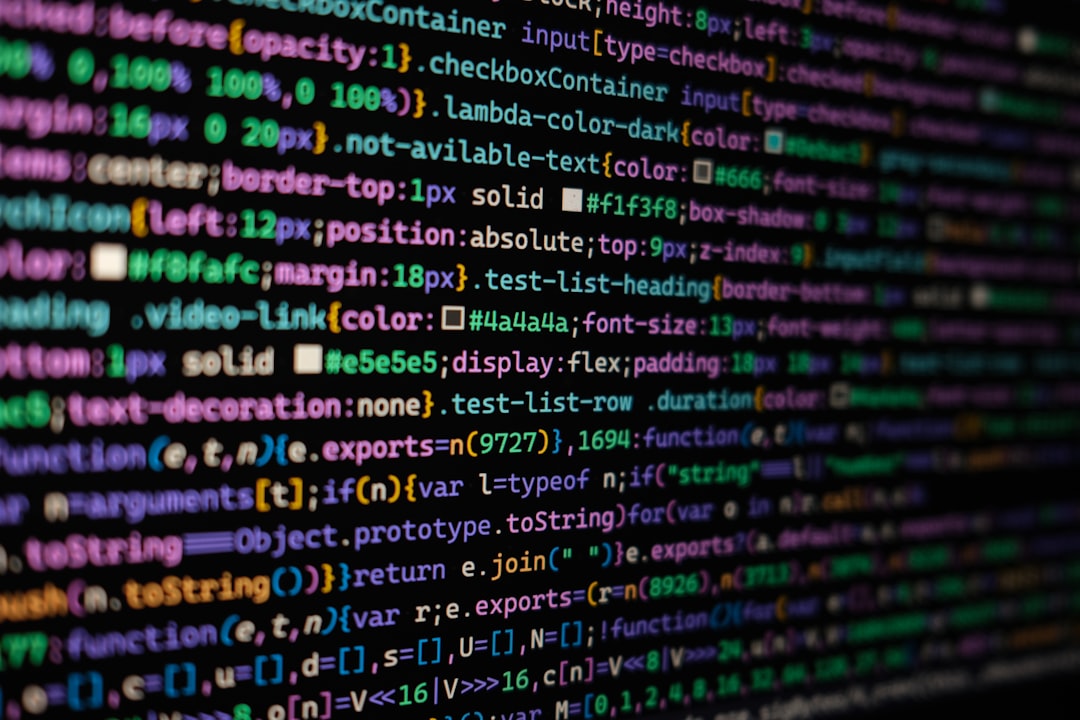
Lodash's `remove` method provides a direct way to modify arrays by eliminating elements that fulfill a specific condition. It takes an array and a predicate function as arguments, modifying the original array by removing any element that evaluates to a truthy value when passed through the predicate. This differs from methods like `filter`, which create a new array while leaving the original array untouched. One noteworthy aspect of `remove` is that it returns an array containing the elements that were removed, making it helpful when you need to track what elements were eliminated. Furthermore, its capacity to handle nested arrays enables more intricate removal operations, expanding its applicability in various JavaScript programs.
Lodash's `remove` method is a powerful tool for directly modifying arrays, but it's crucial to understand its nuances and potential trade-offs. It lets you remove elements based on a predicate, streamlining the process of filtering unwanted data, which can be very useful. Unlike methods like `filter` that create new arrays, `remove` directly alters the original array, saving memory but potentially introducing side effects if the array is referenced elsewhere. This highlights the importance of carefully managing array references to avoid unintended consequences.
While `remove` can be quite efficient, especially for bulk removal, its performance can degrade with large datasets as it potentially involves shifting remaining elements, just like any method that alters the length of an array. Its chainable syntax is a valuable feature for making code more concise, allowing you to combine `remove` with other Lodash methods in a single operation, particularly useful for complex data transformations. The flexibility of the predicate function gives you control over how elements are identified for removal, enabling dynamic removals based on specific conditions.
One significant advantage of `remove` is that it avoids creating sparse arrays, those arrays with gaps left by deleted elements, which can impact performance. This makes it a reliable choice for maintaining efficient array operations. Lodash also provides other methods like `filter` that return new arrays, promoting the functional programming paradigm of immutable data. However, `remove` offers a more direct approach for direct array modification, making it a useful tool when you want to modify the array in place.
It's worth noting that while `remove` is compatible with older JavaScript environments, it doesn't provide the same level of control as methods like `splice` or `filter`, which might be needed for more nuanced scenarios. Ultimately, the choice between these approaches depends on your specific use case and the desired outcome. It's important to consider factors like performance, the need for immutability, and the complexity of your code when choosing the most appropriate approach.
JavaScript Array Manipulation 7 Efficient Methods to Remove Elements in 2024 - Flag-based Removal for Optimized Processing
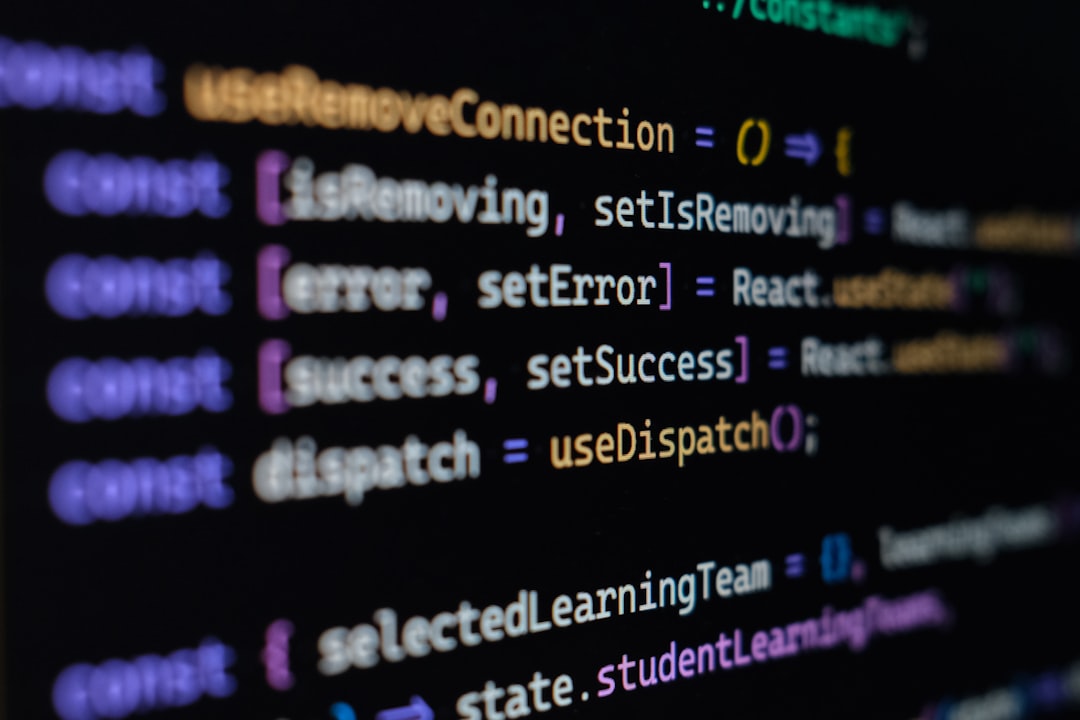
Flag-based removal is a new approach to JavaScript array manipulation. Instead of directly deleting elements, it marks them for removal using a flag. This is especially helpful for large datasets, as it avoids the overhead of shifting elements every time a deletion is needed. The technique optimizes processing by identifying elements for removal without physically altering the array until all operations are complete. This approach improves code maintainability and readability while prioritizing performance in a world of ever-growing datasets.
## A Deep Dive into Flag-Based Removal for Optimized JavaScript Arrays
Flag-based removal in JavaScript arrays is a clever technique that often goes unnoticed, yet it holds the potential for significant performance gains. Instead of directly removing elements during iteration, this method relies on marking them with a flag, indicating they should be removed later. This approach offers a surprising number of advantages.
Firstly, it's incredibly memory efficient. By avoiding the creation of new arrays, as often happens with traditional filtering methods, flag-based removal minimizes the overhead of temporary storage. It simply adds a tag to the existing element, which is much less demanding on your system's resources.
Secondly, flag-based removal can significantly reduce time complexity. What normally takes O(n) time – for both filtering and removing elements – becomes O(1) in a flag-based approach. Why? Because the actual removal of flagged elements can be efficiently batched and executed in a single pass after the flagging process is complete.
Third, this method provides a level of control that direct deletion methods like `splice` can't match. Developers gain the ability to choose when flagged elements should be removed, allowing for customized behavior. For instance, they could log the removal process, perform additional processing steps, or delay deletion entirely.
Furthermore, flag-based removal is safe for iteration over arrays. You can modify array structures while traversing them without the risk of index shifts or skipped elements, a crucial advantage in complex data manipulation scenarios.
Flag-based removal also aligns well with functional programming techniques. By treating removal as a separate operation, it fosters immutability, which improves code readability and maintainability for projects with a functional approach.
The potential for parallel processing is another intriguing aspect. Once elements are flagged as 'deleted,' they can be processed simultaneously in environments that support concurrent execution.
However, there's one crucial point to remember. Using flags completely prevents the creation of sparse arrays. These are arrays with gaps caused by deleting elements without re-indexing, which can dramatically slow down lookups. This is a significant benefit for performance-sensitive applications that rely on quick access to their data.
Finally, it's worth noting that the logic used to flag elements for removal can be tailored to various needs. Different predicate functions can be implemented to define the criteria for marking elements for deletion, making this method highly adaptable and suitable for changing business rules or conditions.
By carefully considering the advantages of flag-based removal, engineers can boost the performance and scalability of their JavaScript applications while ensuring robust and clear code. It's a technique worth exploring and incorporating into your workflow for a more efficient and adaptable approach to array manipulation.
More Posts from :