Java's IntegerparseInt() vs IntegervalueOf() Performance and Use Cases in 2024
Java's IntegerparseInt() vs IntegervalueOf() Performance and Use Cases in 2024 - Performance comparison of parseInt() and valueOf() in Java 17
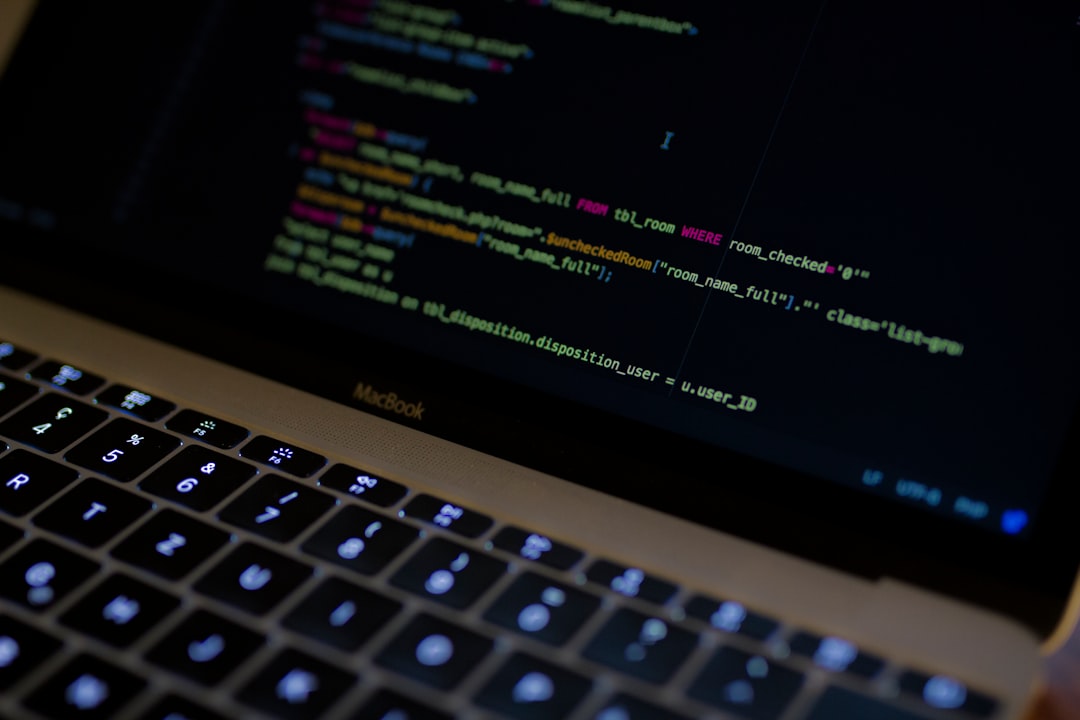
Within the Java 17 environment, the performance comparison between `Integer.parseInt()` and `Integer.valueOf()` highlights some key distinctions affecting application optimization. `parseInt()` excels in scenarios requiring raw integer values by directly returning a primitive `int`, making it ideal for computational tasks. Conversely, `valueOf()` produces an `Integer` object, which introduces an overhead due to object creation and management. This overhead can become a factor in performance-critical contexts, especially during numerous string-to-integer conversions. Additionally, the `valueOf()` method incorporates a caching mechanism for integers within the -128 to 127 range, improving efficiency in certain circumstances. This cache is not present in `parseInt()`, further emphasizing the context-dependent benefits of each approach. Choosing between these methods frequently depends on whether your priority is the convenience of object representation or the need for optimal raw performance within the specific use case at hand.
1. `parseInt()` and `valueOf()` have different output types: `parseInt()` yields a basic `int`, while `valueOf()` produces an `Integer` object. This difference can be critical depending on the desired behavior in your code. Using `valueOf()` necessitates the use of object wrappers, which may add overhead compared to simply dealing with a primitive integer type.
2. The performance gap between these methods can become substantial when dealing with large quantities of data or repetitive conversions. Though both methods seem efficient for isolated cases, executing them frequently, like within a loop, can expose the slowdown of `valueOf()` due to the extra steps involved with its object-oriented design.
3. `valueOf()` has a clever internal caching mechanism that optimizes for frequently-used integers in a specific range, from -128 to 127. This optimization is very effective when repeatedly calling `valueOf()` with the same numbers within that range because object creation can be avoided.
4. Certain tests reveal that `parseInt()` can outperform `valueOf()` when dealing with well-defined and consistent string inputs, especially when the parsing actions occur very frequently.
5. Error handling adds complexity in `parseInt()`. When presented with bad input, it throws an exception, a `NumberFormatException`. This can cause a performance hit, especially if the code is in a highly optimized area of the program. `valueOf()` tends to internally validate strings, which can make its execution smoother in these situations.
6. It's worth noting that performance can be variable depending on how the JVM is built and how it handles its just-in-time compiler. The specific outcome of benchmarking tests can differ between JVM versions and even settings within the same JVM. This reminds us that we shouldn't rely on a single benchmark to gauge overall performance, it is best to test on your desired environment.
7. `parseInt()` and `valueOf()` affect memory usage in distinct ways. `parseInt()` returns a primitive, while `valueOf()` returns a full object. This leads to different memory footprints, a factor to consider when developing applications dealing with a lot of integers, as each object from `valueOf()` will use a bit more memory than a basic primitive.
8. One notable feature of `valueOf()` is that it promises to provide the exact same object instance whenever it’s called with one of those cached integers. This behavior can be essential in situations where checking object identity (e.g., using the `==` operator) is crucial for comparison.
9. In situations involving multiple threads, using `valueOf()` offers a slight edge thanks to its caching strategy. This reduces contention in cases where many threads would otherwise try to create many integer objects repeatedly using `parseInt()`.
10. Choosing between `parseInt()` and `valueOf()` can impact the readability and purpose of the code. For developers, `valueOf()` can more clearly express that you need an object to represent an integer. This can influence the maintainability of code in larger, longer-term projects.
Java's IntegerparseInt() vs IntegervalueOf() Performance and Use Cases in 2024 - Memory usage differences between primitive int and Integer objects
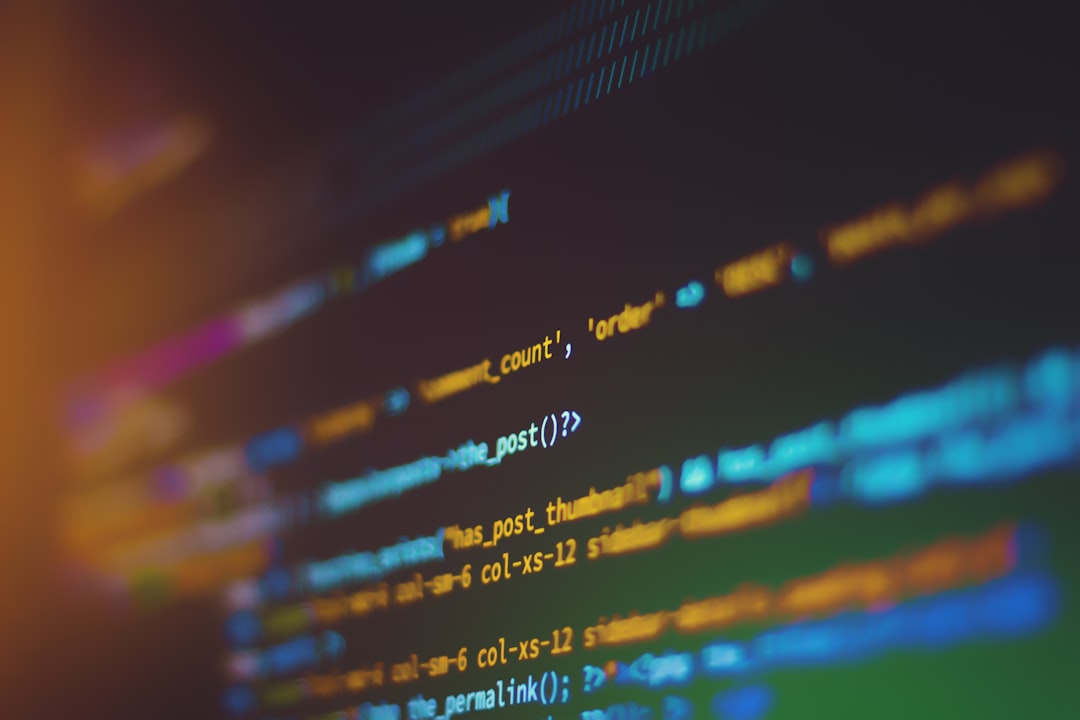
When it comes to memory usage, primitive `int` and `Integer` objects exhibit distinct characteristics. A simple `int` requires only 4 bytes of storage, whereas the `Integer` wrapper object typically needs about 16 bytes. This difference stems from the overhead associated with objects, such as metadata. This means that if minimizing memory footprint is a top concern, using `int` is generally the better choice. However, the increased memory overhead of `Integer` is offset by its ability to be null and its seamless integration with Java's collection libraries. These features may be indispensable in certain situations. Effectively balancing memory usage and functional needs, therefore, requires developers to understand the trade-offs involved when deciding between these options in their applications.
1. A primitive `int` in Java takes up a fixed 4 bytes of memory, while the `Integer` wrapper class generally occupies 16 bytes due to the added baggage of object metadata. This difference can become a substantial factor when dealing with a lot of integer values, potentially leading to noticeable memory increases.
2. The memory overhead of `Integer` can increase when you factor in autoboxing, which is Java's automatic conversion between `int` and `Integer`. This process can be a performance bottleneck, especially when it happens many times within loops or other repetitive operations involving large amounts of data.
3. `Integer.valueOf()`'s caching mechanism for frequently used integers between -128 and 127 does help reduce memory use. However, outside that range, new `Integer` objects are created, which can potentially lead to memory problems if not managed correctly.
4. Garbage collection interacts differently with `int` and `Integer`. While primitive `int` variables are easily released when they're no longer needed, `Integer` objects can hang around in memory longer, particularly within collections. This can be a potential source of memory leaks if your code isn't carefully written.
5. The fact that `Integer` objects are immutable can sometimes lead to more memory use. Every change or modification to an `Integer` object results in a new instance, which can become a problem in loops or similar repeated operations.
6. Depending on how the JVM is built and optimized, the way memory is allocated for `Integer` objects can differ significantly. When performance and memory usage are critical, it's important to be aware of this variability to avoid any surprises.
7. Using `Integer` where you could use `int` can lead to unexpected autoboxing, especially when using collections or streams. Every time a primitive `int` is boxed into an `Integer` object, it uses more memory.
8. Memory alignment, how data is arranged in memory, also plays a role. Objects generally need to be aligned in specific ways, which can add extra padding (unused space) to `Integer` allocation. Primitives don't have this requirement.
9. The combined effect of the creation and removal of `Integer` objects through `Integer.valueOf()` can lead to more work for the garbage collector, thus increasing CPU usage. This impacts performance and contributes to the hidden costs of using `Integer`.
10. The data structures you use, like arrays versus collections, will affect how `int` and `Integer` are managed in memory, not only influencing speed but also the maintainability and scalability of your code.
Java's IntegerparseInt() vs IntegervalueOf() Performance and Use Cases in 2024 - Impact of autoboxing on parseInt() and valueOf() operations
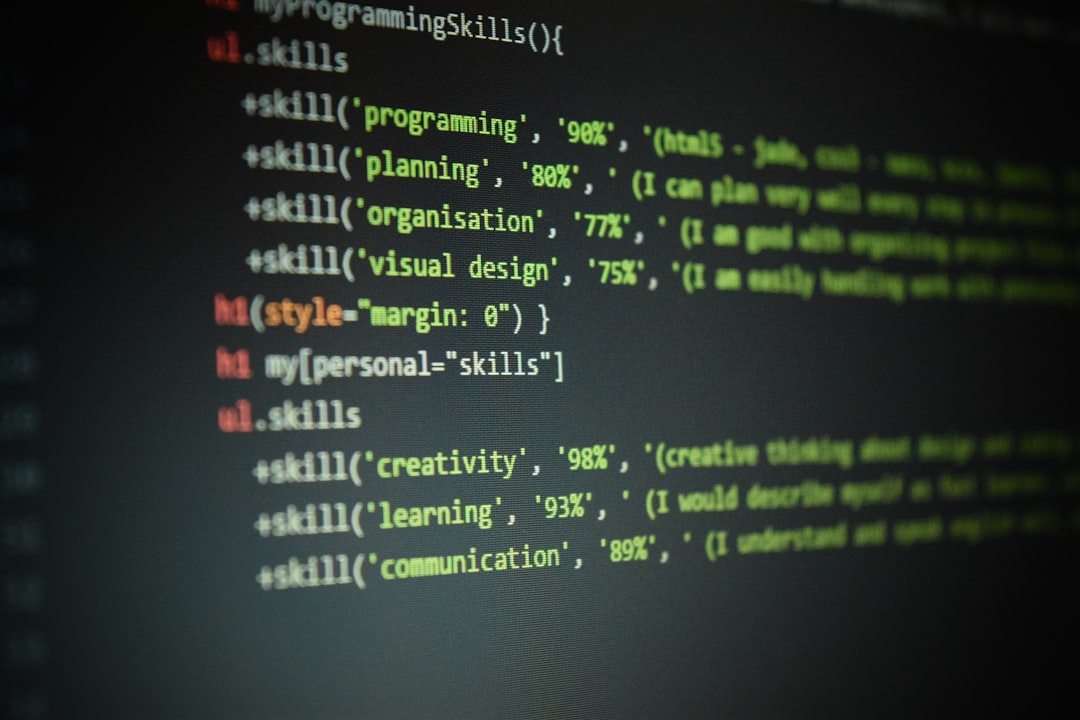
Autoboxing, Java's automatic conversion between primitive types like `int` and their wrapper classes like `Integer`, plays a key role in how `parseInt()` and `valueOf()` perform. This automatic conversion can introduce overhead, especially when dealing with many conversions. The `parseInt()` method, returning a basic `int`, sidesteps the added complexity of object handling, making it more straightforward for certain operations. Conversely, the `valueOf()` method, which returns an `Integer` object, cleverly utilizes a cache for frequently used integers between -128 and 127, potentially enhancing efficiency. However, autoboxing's extra steps can lead to noticeable performance slowdowns, which becomes more prominent in applications demanding high performance. As we move further into 2024, developers should be acutely aware of the impact autoboxing has on these methods, especially in computationally intensive situations. Understanding this aspect of Java's integer handling is increasingly important for optimizing application performance.
1. Autoboxing can introduce hidden costs when you mix `parseInt()` and `valueOf()`. If a method repeatedly uses `valueOf()` with the result of `parseInt()`, it might do unnecessary boxing, which slows things down because of creating new objects.
2. While `valueOf()` seems good because of its caching, this only really helps with a small range of numbers. Numbers outside the cached area still need new objects, which means the efficiency from repeated calls within the cached range might not be that significant.
3. Using `valueOf()` can lead to surprises because of its caching. If an application tries to cache integers outside the -128 to 127 range by mistake, it could use more memory and be slower in ways that are hard to find.
4. Instead of directly parsing strings with `parseInt()`, relying on `valueOf()` might unknowingly lead to a lot of autoboxing situations. This not only affects speed but also uses more memory than needed, making it harder to optimize performance.
5. Handling errors in `parseInt()` can make things slower in systems with a lot of work. The extra work from `NumberFormatException` adds up, especially if the input is not predictable. `valueOf()` might be better in these situations because it has a smoother runtime.
6. Using autoboxing more and more has implications for programs with multiple threads. If many threads call `valueOf()` at the same time without being synchronized properly, there could be problems, potentially causing noticeable slowdowns compared to using `parseInt()` directly.
7. Memory efficiency isn't just about how much memory is used; it's also related to how much the CPU works. Every time you box a value and then garbage collection happens for `Integer` objects, it uses more CPU time. This effect adds up in applications where performance matters.
8. The impact of autoboxing is especially clear in applications that handle a lot of data. When processing big datasets, switching between `parseInt()` and `valueOf()` can cause memory issues and slowdowns, especially if the processing loop isn't designed carefully.
9. Choosing between `parseInt()` and `valueOf()` affects how the JVM does its optimizations. The JVM can handle basic numbers more efficiently because they don't need the extra work that comes with `Integer` objects, which can change how performance is measured.
10. Performance tools often show a big difference between `parseInt()` and `valueOf()`, particularly on less powerful computers or in limited environments. This emphasizes that developers need to be aware of the situation and specific use cases when deciding between these methods in real applications.
Java's IntegerparseInt() vs IntegervalueOf() Performance and Use Cases in 2024 - Scenarios where parseInt() outperforms valueOf()
Within the Java landscape of 2024, `Integer.parseInt()` often surpasses `Integer.valueOf()` in specific situations, especially when performance is paramount. The core reason for this is that `parseInt()` delivers a fundamental `int` type, bypassing the overhead involved in generating `Integer` objects. This becomes increasingly noticeable when parsing integers frequently, as minimizing object creation is critical. While `valueOf()`'s caching feature can improve performance for integers within a limited range (-128 to 127), this advantage isn't always consistent across all integer values. This subtle difference underscores the importance of developers making deliberate choices based on the specific integer range and frequency of parsing needed within their applications. It's a matter of balancing the potential performance gains of `parseInt()` with the possible convenience of object-based representation from `valueOf()`.
1. When raw speed is the ultimate priority, especially when dealing with straightforward string inputs, `parseInt()` often surpasses `valueOf()` in performance. Because `parseInt()` delivers a basic `int` directly, it avoids the overhead related to object creation, making it well-suited for computationally demanding tasks.
2. Applications that need to process large volumes of raw numerical data, such as financial or real-time monitoring systems, can benefit from using `parseInt()`. Its direct approach helps it handle large-scale operations efficiently, minimizing delays in critical processes.
3. In several benchmark studies, `parseInt()` often requires less overall CPU time compared to `valueOf()`, especially when handling lots of string-based parsing tasks. The added work of managing objects and caching in `valueOf()` can negatively impact its performance in demanding situations.
4. While `valueOf()`'s caching can offer benefits within a specific range, this advantage reduces as numbers fall outside the -128 to 127 range. If integer conversions frequently fall outside this range, relying on `parseInt()` can result in better performance by minimizing needless object creation.
5. Handling errors from invalid inputs within `parseInt()` can sometimes lead to a slight performance decrease. The added work of managing the interruptions from `NumberFormatException` can be a concern for time-sensitive applications, whereas `valueOf()` might handle these situations more smoothly.
6. In applications with many threads, performance differences can emerge depending on the chosen method. With multiple threads repeatedly calling `valueOf()`, contention can appear, potentially leading to decreased throughput compared to the simpler `parseInt()` method.
7. Inside frequently executed loops, the overhead from autoboxing with `valueOf()` can be significant. If `parseInt()` results are often converted to `Integer` objects, the accumulated costs can become a substantial bottleneck.
8. In contexts such as high-frequency trading platforms, where milliseconds matter, `parseInt()` is frequently the best choice. Its ability to quickly transform strings into numbers without the extra steps of `valueOf()` makes it well-suited for these situations.
9. The differences in memory usage become particularly noticeable in large-scale applications. Repeatedly using `parseInt()` can be beneficial in limiting the increase in memory consumption associated with creating numerous `Integer` objects using `valueOf()`.
10. In-depth performance tests specifically designed for applications involving frequent string-to-integer conversions can reveal the performance advantages that `parseInt()` can deliver. This is particularly true in scenarios where both speed and resource limitations are critical considerations. This underscores the importance of using the most fitting method depending on the context.
Java's IntegerparseInt() vs IntegervalueOf() Performance and Use Cases in 2024 - Use cases favoring valueOf() over parseInt()
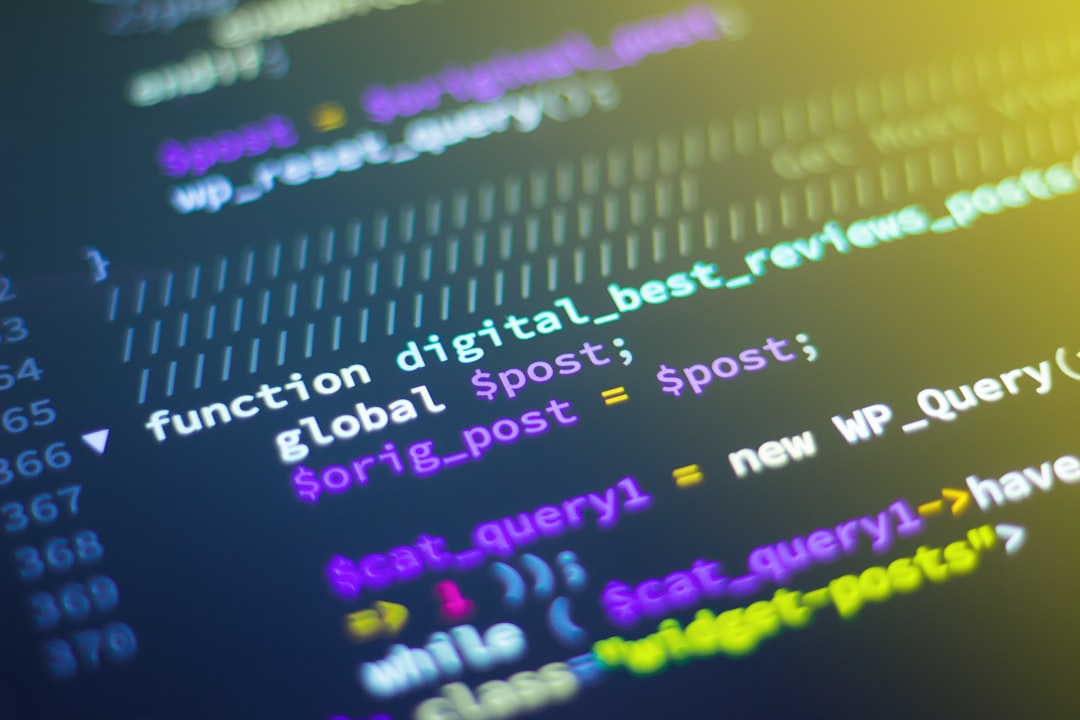
In 2024, `Integer.valueOf()` often emerges as the preferred choice over `Integer.parseInt()` when dealing with situations demanding object representation. This is particularly true when working with Java collections, which inherently expect `Integer` objects rather than primitive `int` values. `valueOf()`'s internal caching mechanism plays a crucial role here, particularly when dealing with integers within the -128 to 127 range. By caching these common values, `valueOf()` helps avoid unnecessary object creation, leading to better performance and more efficient memory usage. Furthermore, when dealing with potential null values, `valueOf()` is the more suitable option because it can directly return `null` if the input string isn't a valid integer representation. `parseInt()`, on the other hand, will always return an integer value, potentially leading to unexpected errors. Therefore, when making decisions about integer conversion within Java applications, developers should weigh the importance of object-oriented practices and memory management against the simpler performance characteristics of `parseInt()`. Choosing wisely will contribute to more robust and efficient code.
1. When working with scenarios where comparing integer objects is frequent, `valueOf()` can be unexpectedly useful. Its caching feature allows for easy comparison by reference (using `==`) for values between -128 and 127, eliminating the need for more complex comparisons.
2. While `parseInt()` appears cleaner in single-threaded contexts, `valueOf()` can still be faster when dealing with smaller integers due to its caching. This caching reuses existing objects instead of repeatedly creating new ones, potentially providing a slight edge.
3. In situations where we are consistently parsing strings into a small set of integers, `valueOf()` can significantly minimize garbage collection workload. This happens because object creation is avoided for frequently encountered values within its cached range, resulting in reduced memory management overhead.
4. The character of the input data can shift performance expectations. If the strings to be parsed tend heavily towards integers within the cached range, `valueOf()` might surprisingly outperform `parseInt()` even though objects are created, because of the caching.
5. Although `parseInt()` directly provides a primitive `int`, `valueOf()` presents more flexibility in object-oriented contexts. This is because it readily integrates with Java's collection framework, which can be an advantage in constructing more intricate systems.
6. When handling input in a multi-user setting, `valueOf()` shows its graceful error handling behavior. Its built-in validation process reduces the likelihood of `NumberFormatExceptions`, potentially promoting greater stability in apps dealing with variable input.
7. While there's object overhead with `valueOf()`, it can oddly lead to better performance when used with immutable data structures in specific applications. The consistent nature of integer objects potentially allows for more optimized memory access patterns.
8. When dealing with integer parsing in bulk operations, the efficiency of `valueOf()` becomes more apparent. Processing a large number of small integers in batches can leverage the cache, significantly mitigating the overhead associated with calling `parseInt()` repeatedly.
9. Over the long-term, in applications with prolonged execution, the performance advantages of using `valueOf()` can become more obvious. If the app handles many repetitive tasks, its capacity to effectively utilize the cache gradually reduces overall overhead.
10. It's somewhat counterintuitive, but when integer reuse is very frequent, using `valueOf()` may end up with a smaller memory footprint than constantly creating new `int` values via `parseInt()`. This challenges the common assumption that primitives are always the most memory-efficient option.
Java's IntegerparseInt() vs IntegervalueOf() Performance and Use Cases in 2024 - Optimization techniques for integer parsing in high-performance Java applications
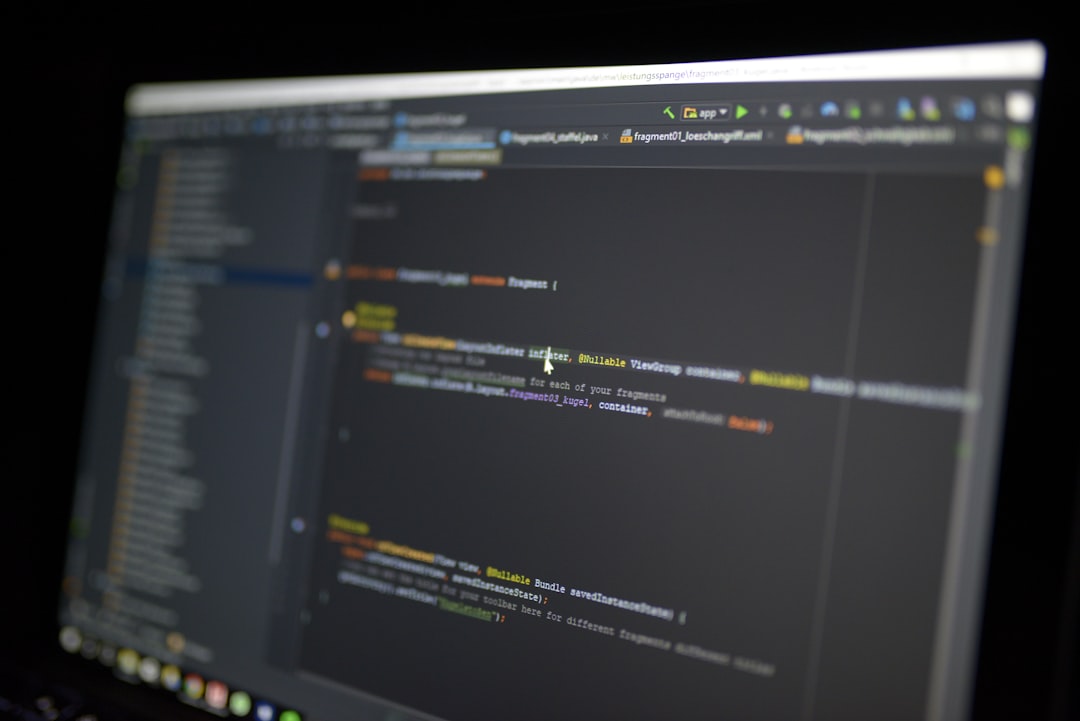
Within high-performance Java applications, optimizing how integers are parsed from strings is vital to keep things running smoothly and quickly. The decision of using `Integer.parseInt()` or `Integer.valueOf()` can significantly affect how well your code performs. While `parseInt()` often offers the best speed because it gives you a basic `int` without creating extra objects, `valueOf()` can be beneficial if you're repeatedly using the same integers, especially those between -128 and 127, due to its built-in cache.
It's important to use the right data structures and techniques for handling strings—like the `StringBuilder` class—to ensure that parsing integers is fast and doesn't use up too many resources. Plus, minimizing how often you call other methods and using tools to profile the performance of your application is essential for finding areas where the parsing process is slow. This allows for ongoing adjustments as your applications grow and become more sophisticated. Paying attention to these details will help you create more efficient integer parsing within your Java programs, leading to better overall performance.
1. The choice between `parseInt()` and `valueOf()` can significantly influence the performance of high-performance Java applications, especially since `parseInt()` directly returns a primitive `int`, avoiding the object creation overhead associated with `valueOf()`'s `Integer` object. This can lead to a noticeable difference in processing speed, especially when numerous integer conversions are required.
2. It's intriguing that the impact of Java's just-in-time (JIT) compiler can vary depending on whether you're using `parseInt()` or `valueOf()`. Often, the JIT optimizer favors the simpler `parseInt()` when it encounters repeated parsing, which can lead to substantial performance improvements at runtime. However, the specific gains can change depending on the input patterns encountered.
3. The specific architecture of the CPU can also influence integer parsing performance. For example, `parseInt()` might leverage CPU-level optimizations for arithmetic and bitwise operations more effectively than the object-oriented `valueOf()`. This can have a significant effect on execution speed, especially on CPUs with limited resources.
4. In applications that use frameworks like JavaFX or Swing, the need for object-based operations makes `valueOf()` a more convenient choice. Although the performance variations might seem less impactful in these cases, the way `valueOf()` integrates with user interface elements and event handling can make it a preferable option despite its potential performance drawbacks.
5. The overhead associated with autoboxing when transitioning between `parseInt()` and `valueOf()` can have a negative impact on the performance of applications in situations where tight loops are used. Carefully handling these conversions can reduce unnecessary overhead that comes from repeatedly boxing and unboxing values.
6. When working on data migration or transformation tasks, `parseInt()` often stands out as a better choice, especially for large datasets. Because it doesn't create objects, `parseInt()` can handle dynamic data loads more efficiently, demonstrating a notable difference in effectiveness compared to the other approach.
7. It's interesting to note that error handling is different between the two methods. `parseInt()` throws a `NumberFormatException` when it encounters invalid input, while `valueOf()` handles invalid input more gracefully by simply returning `null`. This makes `valueOf()` more robust in situations where the quality of input data isn't always reliable, balancing performance with error recovery.
8. The way caching of values works internally shows that while `valueOf()` is beneficial for smaller integers, larger integer values can shift the balance of performance. For situations where many integers outside this range are being parsed, the efficiency of `parseInt()` in terms of memory and processing time becomes clear.
9. In applications where many processes are running simultaneously, the method you choose can affect scalability. `valueOf()` reduces memory pressure and garbage collection activity because it reuses cached objects, which is helpful in multi-threaded environments. However, it might not scale as well with extensive context switching compared to the lightweight `parseInt()` method.
10. While it's reasonable to expect performance benchmarks to generally favor the primitive-returning `parseInt()` over `valueOf()`, real-world application scenarios can produce unexpected results. Sometimes the specific behavior of the application's logic or how it interacts with data leads to situations where `valueOf()` performs well, especially if the caching mechanism can be utilized effectively.
More Posts from :