7 Critical Time Zone Handling Techniques with ServiceNow's GlideDateTime API
7 Critical Time Zone Handling Techniques with ServiceNow's GlideDateTime API - Converting UTC Timestamps to Local Time Using GlideDateTime setTZ Method
Within the ServiceNow realm, specifically when working with the GlideDateTime API for time zone management, converting UTC timestamps to the local user's time zone is a cornerstone practice. This conversion is achieved primarily through the `setTZ` method, which necessitates a TimeZone object as input. It's important to emphasize that simply adjusting the time zone might not be enough. To accurately present the converted timestamp to users, utilize `getDisplayValue`. This ensures that the display correctly reflects the local time. The `getLocalTime` method then corroborates that the date and time are correctly represented in the desired local time. Furthermore, methods like `addDaysLocalTime` grant flexibility for adjusting date values, increasing the versatility of the API. Mastering time zone conversions not only guarantees accuracy in how time is presented but also mitigates the potential pitfalls of inconsistencies sometimes called "time warping". By adhering to these practices, you ensure that time-sensitive aspects of your ServiceNow applications function reliably across diverse locations and users.
ServiceNow's GlideDateTime class offers a `setTZ` method that's handy for converting UTC timestamps to local time zones. It works by directly changing the internal time representation, which is a quicker approach compared to methods that might involve database interactions, avoiding potential delays.
However, it's important to remember that different time zones have varying daylight saving rules, and the `setTZ` method cleverly handles these automatically, ensuring consistent results even when these adjustments occur. One thing to be mindful of is that time zone names are case-sensitive within GlideDateTime; "America/New_York" and "america/new_york" are treated differently, and forgetting this could lead to unforeseen issues.
Furthermore, the effect of `setTZ` extends beyond how the time is displayed. It also alters how GlideDateTime functions operate in scripts. This means any scripts relying on local times will behave as expected only if the time zone is correctly configured using `setTZ`. Being able to dynamically switch the timezone is an appealing aspect, particularly for applications catering to distributed teams, simplifying their workflows.
But this flexibility also presents a challenge: You need to validate the time zone inputs to prevent errors. Providing an invalid time zone to `setTZ` will throw exceptions, and we don't want this impacting operations. In addition to common time zones, `setTZ` also allows for more granular time adjustments, such as half-hour and quarter-hour offsets, which is helpful in places with unconventional time zone settings.
Also, the GlideDateTime class relies on the IANA time zone database to keep its time zone definitions and conversion logic up-to-date, a necessary step for applications operating across different regions and jurisdictions. While the `setTZ` approach is generally efficient, it's crucial to use it wisely. If a script frequently utilizes `setTZ` within loops, performance may suffer. Thus, optimizing the code for situations with repeated time zone conversions is essential.
Lastly, it's important to remember that simply converting from UTC to local time doesn't solve every time-related challenge. Some applications need even higher accuracy, especially when dealing with historical time zone changes or events like leap seconds, which are complexities that often get overlooked.
7 Critical Time Zone Handling Techniques with ServiceNow's GlideDateTime API - Scheduling Background Jobs Across Multiple Time Zones with GlideSchedule
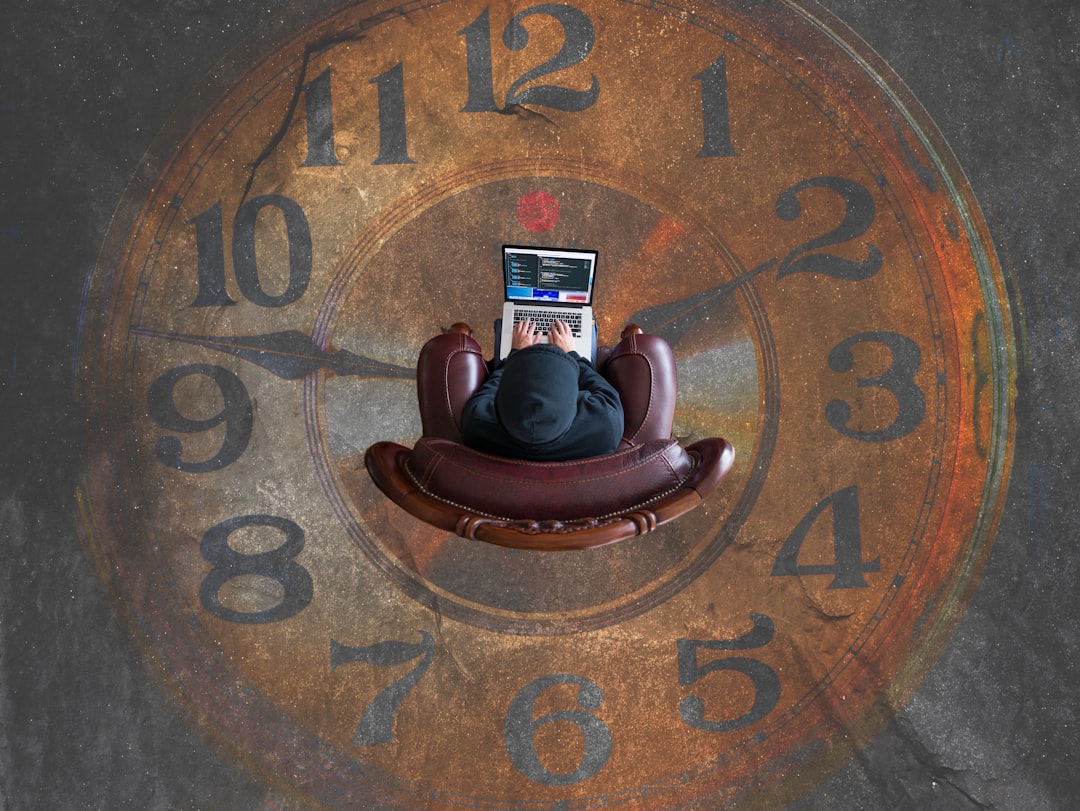
When dealing with background jobs across multiple time zones in ServiceNow, GlideSchedule provides a mechanism to schedule tasks based on the local time of the users impacted by those jobs. This means a job can be set to run at 9 AM, but that 9 AM will be interpreted as the local time of the specific user or group of users related to that job. GlideSchedule deftly handles daylight saving time adjustments automatically, taking one less thing off your plate. It also means you can, with reasonable confidence, avoid a lot of errors in time-sensitive processes.
However, there's a catch. Just like with the `setTZ` method in GlideDateTime, you have to be cautious of bad input. Providing an invalid time zone to GlideSchedule could cause disruptions you may not anticipate. It's worth noting that while GlideSchedule simplifies time zone management, there's a level of complexity that arises in global environments. The need to reconcile different local times with the need to maintain the integrity of your ServiceNow processes, becomes a bit of a balancing act. While GlideSchedule reduces a lot of the headaches that time zones cause, it is still a feature you have to carefully consider when designing processes in ServiceNow, especially those tied to time-sensitive elements.
ServiceNow's GlideSchedule offers a powerful way to automate tasks across multiple time zones, but it's not without its complexities. When working with GlideSchedule, it's crucial to be mindful of how different time zones can interact with your jobs.
For example, not every region uses Daylight Saving Time (DST), and even among those that do, the dates can change. This variation can create problems if not handled carefully when scheduling jobs. You also have to deal with time zones that are not full-hour offsets, like India's UTC+05:30. GlideSchedule can manage this, but it adds another layer to your design.
Imagine you have a job in one time zone that starts another job in a different one. If the initial job fails, it can cascade through your system, potentially causing widespread disruptions. And, because of this, error handling becomes even more important, as it can help prevent these ripple effects.
Historical data can be tricky too. Time zones change over time– countries adjust their GMT offsets, borders shift – and if you're not prepared for this, it can lead to headaches. One way to deal with this is to make sure the IANA time zone database used by GlideDateTime is always up-to-date, but it's still a source of possible issues.
Then there's the issue of timezone abbreviations. Abbreviations like PST or EST can be a bit ambiguous, particularly in multi-regional environments. While UTC is always clear, these abbreviations aren't, which can lead to problems if you're not cautious.
Also, it's important to consider how your ServiceNow instance's server time zone can interact with your jobs. If there's a mismatch between the server time and the time zone of your job, you could end up with unexpected results.
And, like many other programming aspects, you need careful input validation to prevent incorrect time zone values from creating problems. It's easy to forget to check this and end up with errors that could interrupt your workflows.
Lastly, consider the performance implications of how frequently you plan to schedule your jobs across multiple time zones. While GlideSchedule can handle frequent scheduling, keep in mind that a job that runs every minute in a dozen time zones can cause performance issues. Also, you should never assume that your code is necessarily fast– if you frequently change time zones within loops, it can become slow, and you might need to consider alternative code structures.
These are just a few things to keep in mind when working with GlideSchedule across multiple time zones. If you take the time to understand the nuances of time zone management, you can use GlideSchedule to create powerful automated processes for your workflows.
7 Critical Time Zone Handling Techniques with ServiceNow's GlideDateTime API - Managing Date Calculations Between Different Regions Using addDaysLocalTime
When dealing with date calculations across different geographical regions within ServiceNow, the `addDaysLocalTime` method offers a practical solution. It enables developers to adjust dates by adding a set number of days, all while factoring in the user's local time. This ensures that date manipulations are in sync with regional time zone settings. This is especially crucial when applications cater to a diverse user base spanning multiple time zones, as inaccurate date calculations can disrupt schedules and lead to operational problems. The `addDaysLocalTime` method helps mitigate these issues by ensuring the calculations are consistent with the user's location, promoting a seamless user experience. However, this is not a panacea. It's important to validate inputs carefully to prevent errors and also acknowledge that daylight saving time adjustments can influence date calculations. For developers seeking to build robust global applications, understanding and employing methods like `addDaysLocalTime` becomes vital for ensuring consistent and accurate date handling, improving application reliability and minimizing the potential for errors due to conflicting time zones.
ServiceNow's GlideDateTime API offers the `addDaysLocalTime` function, which lets you tweak dates by adding or subtracting days. This is especially useful when planning events or tasks across different regions, since you can easily calculate both past and future dates.
One neat thing about `addDaysLocalTime` is its awareness of local calendars. It can automatically factor in holidays and non-working days specific to certain regions, which helps make date calculations more accurate for scheduling purposes. You might not expect this, but it does improve the usability of the function.
While adding days might seem basic, `addDaysLocalTime` cleverly handles daylight saving time changes. This is significant because crossing a daylight saving boundary can drastically change the time portion of the calculated date. This feature ensures the calculated date takes daylight savings into account, which can be a major issue to work around otherwise.
When you use `addDaysLocalTime`, the API always adjusts the dates based on the user's local timezone, not the server time. This makes the result seem more immediate and intuitive from the user's perspective. It's a simple but effective way to improve the user experience.
From a performance perspective, `addDaysLocalTime` is efficient in its approach. It only performs calculations when you call it, rather than constantly keeping multiple time representations. In situations with limited resources, this can be a real benefit.
Interestingly, `addDaysLocalTime` handles unexpected inputs pretty well. If you mistakenly use a non-existent date (like February 30th), instead of causing errors, it rolls the date forward to accommodate it. This shows a degree of resilience in dealing with user errors that can often be overlooked when working with dates and times.
`addDaysLocalTime` is quite helpful in applications that span across different parts of the world, as it easily adapts dates without requiring a lot of complex timezone management code. This makes things easier for developers to get up and running with globalized processes, but it also highlights that using this approach in a more ad-hoc manner may cause issues down the line if testing is not done properly.
Since timezone laws change occasionally, `addDaysLocalTime` depends on the up-to-date IANA time zone database. This is crucial for ensuring operations stay accurate and predictable as laws change. While this is not a particularly challenging aspect, it's a key design decision to keep in mind.
It's easy to overlook that `addDaysLocalTime` works nicely with the `getDisplayValue` method. This allows dates to be displayed in a format that matches the user's local preferences. This greatly minimizes confusion when working in international applications.
While `addDaysLocalTime` is a powerful tool, improperly managing local time references can still lead to scheduling errors. It highlights how important it is to do thorough testing, especially in multi-regional projects that span different parts of the world and rely on dates and times to execute.
7 Critical Time Zone Handling Techniques with ServiceNow's GlideDateTime API - Standardizing DateTime Display Formats Across Global User Bases
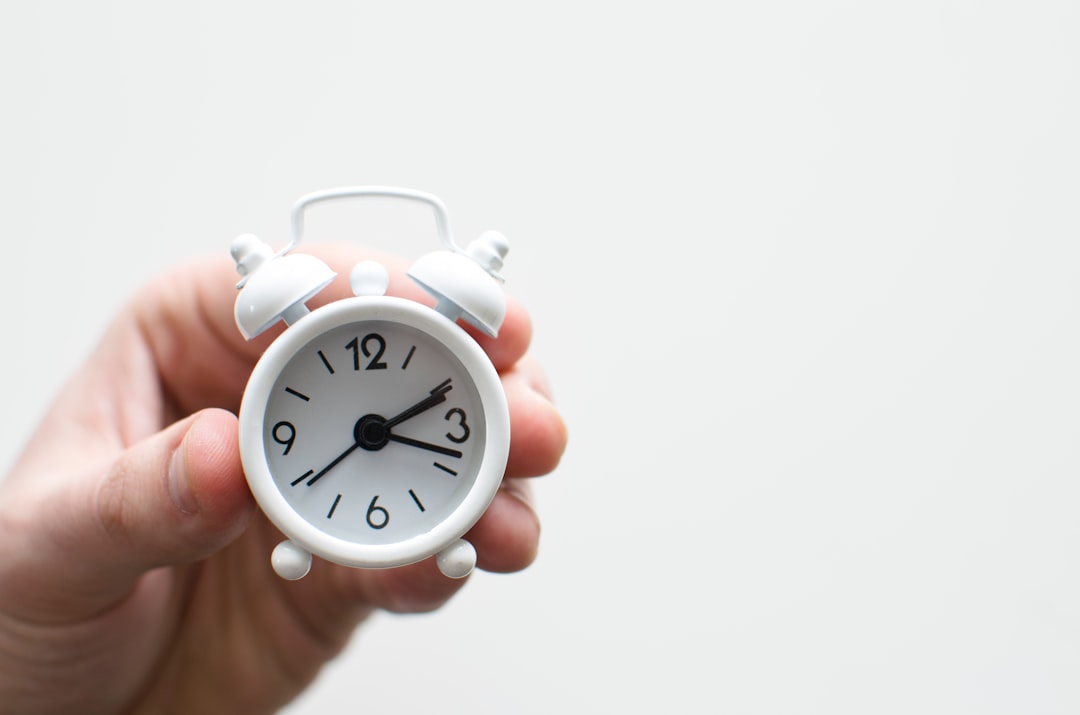
When building applications for a global user base, ensuring that date and time information is presented in a consistent and understandable way is crucial. Different regions use different conventions for displaying times, with variations in the use of 12-hour versus 24-hour clocks, the inclusion of AM/PM indicators, and other formatting differences. This can lead to confusion and misunderstandings if not addressed properly. ServiceNow's GlideDateTime API helps us tackle this challenge by allowing us to set and display date and time values in a format tailored to the user's specific time zone. This can create a more seamless and intuitive experience for users.
However, relying on this flexibility introduces a new challenge: if we don't use format specifications carefully, we can encounter unexpected runtime errors. Ensuring consistency requires paying attention to the format parameters within the API to avoid these errors. As more applications reach a global audience, standardization of date and time displays has moved beyond just a helpful feature to something that is vital for maintaining clarity and user-friendliness across all users, regardless of their location. If we don't consider these diverse display preferences, our applications risk becoming frustrating or even unusable for a large portion of our potential audience.
When designing applications that cater to a diverse global audience, handling date and time information accurately becomes paramount. Different regions often have distinct preferences for how dates and times are presented, leading to potential misunderstandings if not addressed carefully. For instance, while the United States often favors the MM/DD/YYYY format, many European countries prefer the DD/MM/YYYY convention. This disparity can lead to a confusing user experience if not addressed through a universal display standard.
Furthermore, time zone abbreviations can be a source of ambiguity. An abbreviation like CST could represent Central Standard Time in the US or China Standard Time, creating a challenge for accurate scheduling or communication in global applications. Simply relying on abbreviations might not be sufficient when precision is required.
Adding to this complexity is the fact that time zones are not static. Many regions have adjusted their UTC offsets throughout history, often due to political or economic shifts. Failing to account for these historical changes can introduce inaccuracies in applications that rely on timestamps from the past, necessitating consideration of historical timezone updates for accurate results.
Another factor that can impact timekeeping in global applications is the introduction of leap seconds. These seconds are occasionally inserted into UTC to keep the clock synchronized with Earth's rotation, and if not handled properly, can create issues in systems that depend on highly precise timekeeping. This highlights the importance of careful time handling in situations where high precision is demanded.
Moreover, the handling of Daylight Saving Time (DST) further complicates matters. Regions have varying DST practices, if any, leading to differing start and end dates. Failing to account for these differences can lead to scheduling conflicts and errors in applications designed to work across various locales. It's a fine line to walk, as many applications are only locally relevant.
Beyond technical considerations, user preferences for date display formats add another layer of complexity. Users may prefer a date display that differs from their local standards, emphasizing the need for flexibility in how date and time information is presented. While this is a desirable trait, it can lead to more complex development processes.
In addition, many regions utilize different calendar systems. For example, the Gregorian calendar is widely adopted in the west, but other regions rely on calendars like the Islamic or Chinese calendars. Providing applications that are sensitive to the cultural context of the users can contribute to a better overall experience. It's a feature that is often overlooked during design.
It's also important to acknowledge that systems often don't handle invalid dates gracefully. When functions like `addDaysLocalTime` are used, developers must consider how to handle situations like accidentally entering February 30th. Implementing error handling for these situations can enhance the robustness of applications in diverse environments. Not dealing with these sorts of scenarios can quickly degrade user trust in an application.
Furthermore, time zone databases, like the IANA database, are continuously updated to reflect ongoing changes in global time zones. Applications that rely on these databases should incorporate regular updates to ensure they are always presenting current and accurate information. It's a recurring maintenance chore that's easily overlooked.
Finally, the impact of regional holidays on business operations should not be overlooked. Incorporating regional holiday calendars within applications helps streamline operational efficiency and demonstrate sensitivity to cultural norms in different areas. It's a good way to show that you understand your user's culture.
These are some key considerations for ensuring the reliable management of date and time information across global users. Developing effective solutions involves balancing user experience, functionality, and technical robustness, making it a challenge worth researching.
7 Critical Time Zone Handling Techniques with ServiceNow's GlideDateTime API - Implementing Time Zone Aware Notifications for Global Teams
When working with global teams spread across multiple time zones, sending notifications that are aware of each person's local time becomes crucial for smooth communication and collaboration. If you don't consider time zones, you risk sending notifications at inconvenient times, potentially leading to missed responses or frustration. This means notifications need to be adjusted to the user's time zone so that they arrive when they are likely to be working or otherwise available, increasing the chances of being read.
Beyond simply avoiding annoyance, being sensitive to time zones demonstrates respect for people's individual work schedules and helps maintain a healthy work-life balance. If you constantly interrupt people outside of their normal work hours with notifications, it can create a negative experience. The idea is to design notifications so that they appear at a time that aligns with the recipient's typical working day, improving the likelihood of prompt responses.
Implementing this kind of awareness can involve using tools that automatically convert notification times to the user's local time zone, eliminating the need for manual adjustments. The aim is to provide a straightforward experience for users, regardless of their location. While seemingly simple, designing notifications that are time zone aware can help optimize workflow by ensuring timely delivery of information, and ultimately, this can contribute to greater productivity. It may seem like a minor detail, but implementing time zone-aware notifications can significantly impact team effectiveness when dealing with geographically diverse teams.
When designing notification systems for global teams, ensuring they're time zone aware presents a fascinating set of challenges. One unexpected issue is that time zones aren't static. Political decisions or legislation can lead to changes in a region's time zone, making it crucial to stay updated to maintain accuracy.
Daylight saving time adds another layer of complexity. While some regions use it, others don't, and even within those that do, the start and end dates differ. These discrepancies can alter when notifications are perceived, making scheduling a bit trickier.
Furthermore, the GlideDateTime API, while helpful, requires careful attention to detail due to its case-sensitive nature when specifying time zones. Using "Europe/London" instead of "europe/london" might lead to problems, highlighting the importance of precise input validation.
Another interesting quirk is that not all time zones are aligned to full hours. Regions like India use UTC+05:30, requiring meticulous care when crafting notification schedules to avoid delivering messages at unexpected times.
Individual users often have preferences for how time is displayed, such as 12-hour or 24-hour formats. Catering to these preferences through a notification system can significantly improve the user experience, but it also increases the development complexity.
Moreover, as our applications deal with events from the past, we often need to be aware that time zone boundaries and UTC offsets have changed over time. Notifications tied to historical events may necessitate a deeper dive into historical time zone data to ensure accuracy.
Research suggests that the time of email delivery significantly affects open rates. Sending notifications at the optimal time, localized for each recipient, enhances engagement, again emphasizing the importance of careful time zone handling.
Occasionally, leap seconds are introduced into UTC to keep clocks aligned with Earth's rotation. These can cause unexpected behavior in scheduled notifications if not properly addressed, showcasing the need for high-precision timekeeping in global applications.
Should a notification trigger further actions across different time zones, a single error can easily disrupt the entire process. Implementing a thorough error handling strategy for these cascading workflows is essential to maintain the stability of the system.
Lastly, when optimizing the timing of notifications, running A/B tests across diverse time zones can yield different outcomes due to regional habits and preferences. This need for experimentation highlights the complexities involved in balancing diverse user experiences across global teams.
It's clear that building a system that handles notifications effectively across different time zones is a complex endeavor. It requires a good understanding of the nuances of time zones and how they interact with a notification system to provide users with the best experience.
7 Critical Time Zone Handling Techniques with ServiceNow's GlideDateTime API - Building Custom Time Zone Selection Interfaces with GlideDateTime API
When building ServiceNow applications for users around the world, providing a way to select their own time zone is a must for a good experience. The GlideDateTime API gives you the tools to make this happen. Using methods like `setTZ`, you can adjust how dates and times are displayed based on each user's settings. But there are things to keep in mind. Time zone names are case-sensitive, so "America/New_York" and "america/new_york" are different. You also need to carefully validate the time zone a user selects to prevent errors. Handling daylight saving time changes correctly is another crucial aspect that requires careful consideration when developing the interface. Designing a custom time zone selector that works well can make a big difference in how people interact with your application, especially for things like notifications. If you get this right, your app will be more user-friendly and reliable for users across the globe. But if you don't, you risk introducing problems that could impact application reliability and user trust.
ServiceNow's GlideDateTime API provides a way to dynamically adapt to individual user time zones. This means when people in different parts of the world interact with your applications, the time is automatically adjusted, without requiring anyone to worry about time differences. This significantly cuts down on potential errors caused by mismatched time zones during collaboration.
However, this flexibility comes with a catch: time zone names within GlideDateTime are case-sensitive. A simple error like using lowercase characters in a time zone name can lead to errors you might not anticipate. Because of this, during development, input validation needs to be thorough.
The GlideDateTime API not only supports whole hour offsets but also granular time settings like UTC+05:30, which reflects the reality that time zone settings aren't always simple, full-hour adjustments. This added flexibility lets you account for those unusual, but real-world time zone settings in different regions.
Daylight Saving Time changes are automatically accounted for within the API, ensuring that scripts and processes aren't tripped up by the annual transitions. This might seem like a minor point, but it’s vital for applications that rely on accurate timekeeping.
One thing to watch out for when using the GlideDateTime API is errors caused by invalid inputs. If you provide a time zone that isn't recognized, the API will throw an error. To ensure smooth operation, any application using this API needs a strategy for managing those exceptions so that they don't cause problems for users.
It's also important to remember that time zones aren't static. Countries and regions can shift their UTC offset because of political events or other factors. The GlideDateTime API must stay updated with these changes if it's going to keep historical time data accurate.
Using the `setTZ` function too frequently within code, like within loops, can lead to a performance hit. For applications that are heavily used or that rely on frequent time zone adjustments, it's important to carefully consider the code structure and choose the most efficient approach to ensure performance doesn't suffer.
The GlideDateTime API also shows a degree of sensitivity to local calendars. This means that it can take into account the specific holidays and non-working days in a given region. This flexibility helps improve the overall usability of applications in culturally diverse environments.
It's a good practice to avoid relying too heavily on time zone abbreviations like CST. The same abbreviation can represent different standards in different regions (CST can mean Central Standard Time or China Standard Time). This can lead to scheduling errors if you're not careful about the specific meaning of the abbreviation, particularly in situations where high accuracy is required.
Leap seconds might not seem like a big deal, but they can impact the accuracy of highly precise applications. If you're designing an application that needs extremely accurate timekeeping, it's crucial to make sure that you've properly considered the effects of leap seconds to prevent scheduled processes from being unexpectedly disrupted.
More Posts from zdnetinside.com: